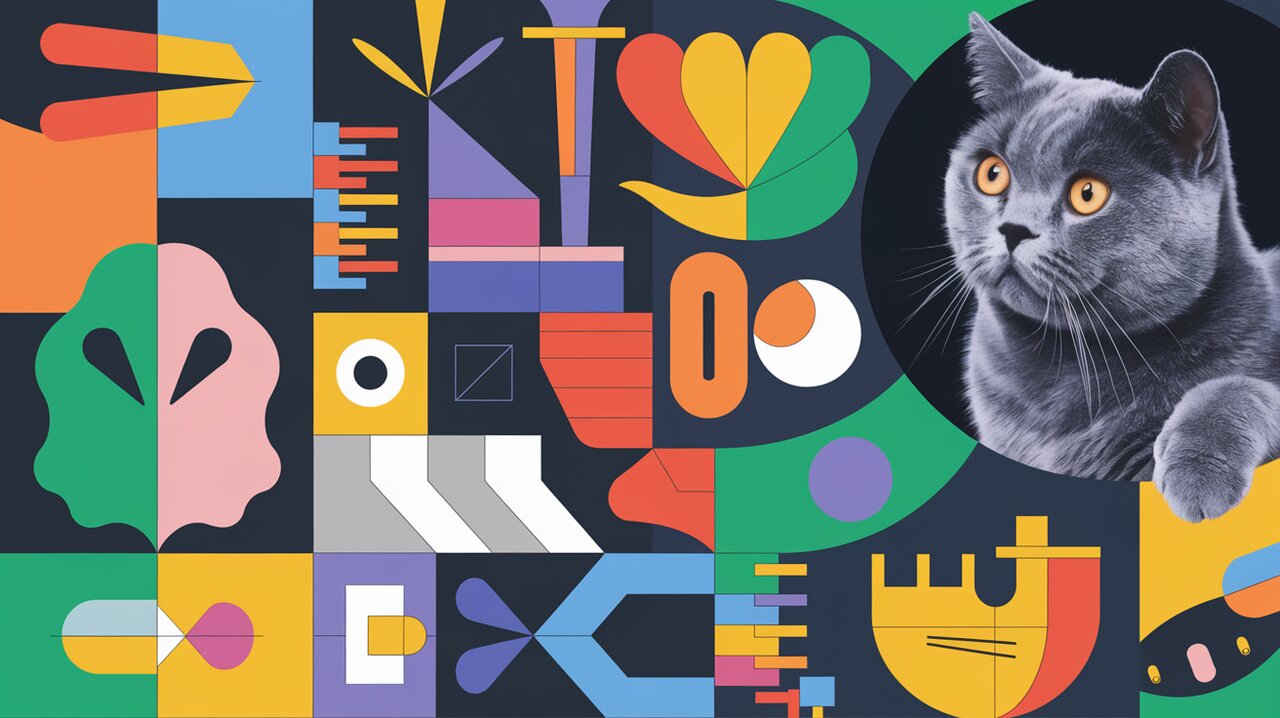
Grommet: Orchestrating React UI Symphonies
Grommet is a powerful React-based framework that has been making waves in the world of web development. It offers a unique blend of design system principles and development tools, allowing developers to create stunning, accessible, and responsive web applications with ease. In this article, we’ll explore the key features of Grommet and how it can revolutionize your React development process.
What is Grommet?
Grommet is more than just a UI component library; it’s a comprehensive framework that provides a solid foundation for building modern web applications. At its core, Grommet is:
- A React-based framework
- A design system
- A collection of accessible and responsive components
- A theming engine
With these elements combined, Grommet offers a powerful toolkit for developers and designers alike.
Key Features of Grommet
Accessibility First
One of Grommet’s standout features is its strong focus on accessibility. The framework provides built-in support for W3C’s WCAG 2.1 specification, ensuring that your applications are usable by people with diverse abilities. This includes:
- Keyboard navigation support
- Screen reader tags
- Color contrast compliance
By using Grommet, you’re taking a significant step towards creating inclusive web experiences.
Responsive and Mobile-First
In today’s multi-device world, responsiveness is crucial. Grommet embraces a mobile-first approach, ensuring that your applications look great and function well on devices of all sizes. The framework’s responsive design system allows you to create layouts that adapt seamlessly to different screen sizes.
Modular and Customizable
Grommet follows atomic design principles, allowing you to build complex UIs from simple, reusable components. This modular approach not only speeds up development but also promotes consistency across your application. Moreover, Grommet’s theming capabilities enable you to easily customize the look and feel of your components to match your brand identity.
Rich Component Library
Grommet offers a wide range of pre-built components that cover most common UI needs. From basic elements like buttons and inputs to more complex components like data tables and charts, Grommet has you covered. These components are not only functional but also aesthetically pleasing and customizable.
Getting Started with Grommet
To start using Grommet in your React project, you can install it via npm or yarn:
npm install grommet styled-components --save
or
yarn add grommet styled-components
Once installed, you can import and use Grommet components in your React application:
import React from 'react';
import { Grommet, Box, Button, Heading } from 'grommet';
const theme = {
global: {
colors: {
brand: '#228BE6',
},
font: {
family: 'Roboto',
size: '18px',
height: '20px',
},
},
};
function App() {
return (
<Grommet theme={theme}>
<Box align="center" pad="large">
<Heading>Welcome to Grommet!</Heading>
<Button primary label="Click me" onClick={() => alert('Hello, Grommet!')} />
</Box>
</Grommet>
);
}
export default App;
This simple example demonstrates how easy it is to get started with Grommet. You can customize the theme to match your design requirements and start building your UI with Grommet’s components.
Advanced Usage
Theming
Grommet’s theming system is powerful and flexible. You can create custom themes to ensure your application aligns with your brand guidelines:
const customTheme = {
global: {
colors: {
brand: '#1ADAEC',
'accent-1': '#FFC107',
},
font: {
family: 'Helvetica',
},
},
button: {
border: {
radius: '4px',
},
},
};
<Grommet theme={customTheme}>
{/* Your app components */}
</Grommet>
Responsive Layouts
Grommet’s Box
component is a versatile tool for creating responsive layouts:
import { Box, ResponsiveContext } from 'grommet';
function ResponsiveLayout() {
return (
<ResponsiveContext.Consumer>
{size => (
<Box
direction={size === 'small' ? 'column' : 'row'}
pad="medium"
gap="small"
>
<Box background="brand" pad="large">First</Box>
<Box background="accent-1" pad="large">Second</Box>
</Box>
)}
</ResponsiveContext.Consumer>
);
}
This example demonstrates how to create a layout that adapts to different screen sizes, switching between a row and column layout based on the available space.
Conclusion
Grommet offers a comprehensive solution for building accessible, responsive, and beautiful React applications. Its focus on accessibility, combined with a rich set of components and powerful theming capabilities, makes it an excellent choice for developers looking to create modern web experiences efficiently.
By leveraging Grommet, you can streamline your development process, ensure consistency across your application, and deliver user-friendly interfaces that work well on all devices. Whether you’re building a small project or a large-scale application, Grommet provides the tools and flexibility you need to bring your vision to life.
As you continue your journey with React development, consider exploring other UI libraries and frameworks to expand your toolkit. You might find our articles on Chakra UI React Symphony and Ant Design UI Symphony helpful for comparing different approaches to building React UIs.
Embrace the power of Grommet and start orchestrating your own React UI symphonies today!