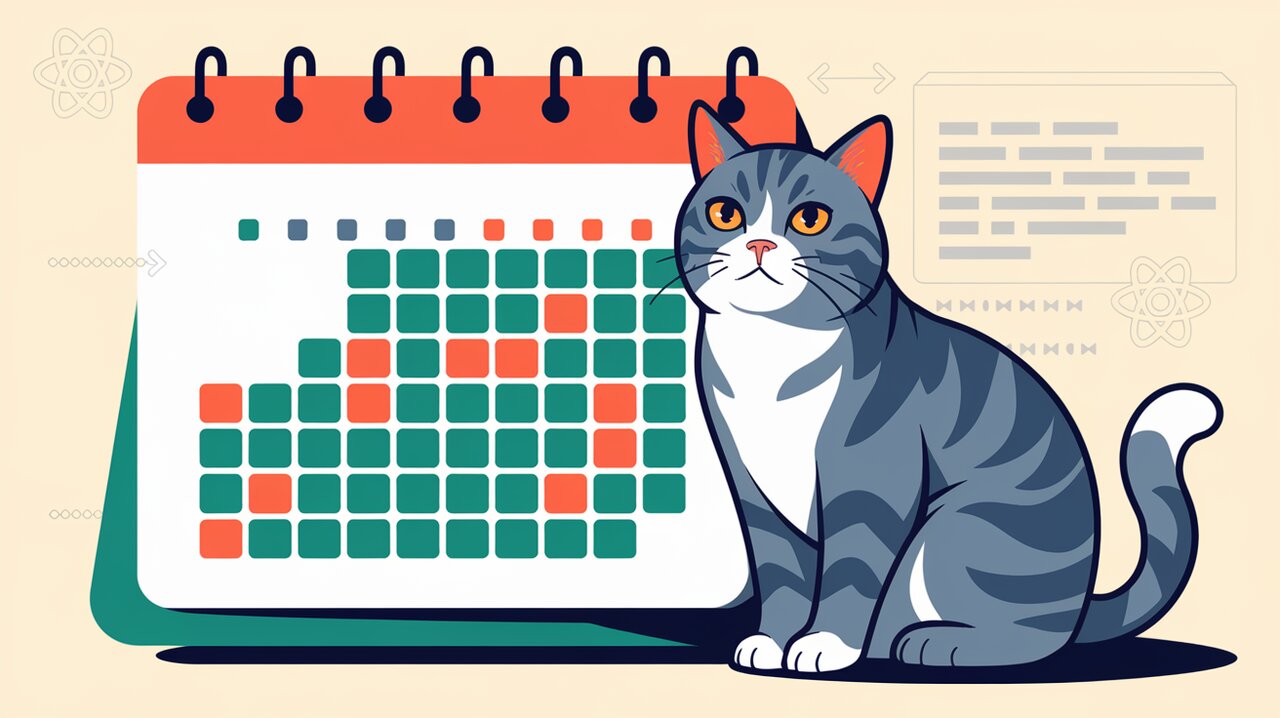
Ignite Your React Apps with @uiw/react-heat-map: A Fiery Calendar Visualization
In the realm of data visualization, few tools capture the essence of time-based trends as elegantly as a calendar heatmap. Enter @uiw/react-heat-map, a lightweight and customizable React component that brings the power of GitHub-style contribution graphs to your applications. Whether you’re tracking user activity, monitoring task completion, or visualizing any time-series data, this library offers a visually appealing and intuitive way to present information.
Illuminating Features
@uiw/react-heat-map comes packed with a range of features that make it a standout choice for developers:
- SVG-based rendering for crisp, scalable graphics
- Customizable colors, sizes, and styles
- Flexible date range support
- Tooltip integration for detailed information display
- Legend customization options
- Week and month label localization
Igniting Your Project
To start using @uiw/react-heat-map in your React application, you’ll need to install it first. You can do this using either npm or yarn:
npm install @uiw/react-heat-map --save
Or if you prefer yarn:
yarn add @uiw/react-heat-map
Sparking Your First Heatmap
Let’s dive into creating your first calendar heatmap with @uiw/react-heat-map. Here’s a basic example to get you started:
import React from 'react';
import HeatMap from '@uiw/react-heat-map';
const value = [
{ date: '2023/01/11', count: 2 },
{ date: '2023/01/12', count: 20 },
{ date: '2023/01/13', count: 10 },
// Add more data points as needed
];
const MyHeatMap = () => {
return (
<HeatMap
value={value}
weekLabels={['', 'Mon', '', 'Wed', '', 'Fri', '']}
startDate={new Date('2023/01/01')}
/>
);
};
export default MyHeatMap;
In this example, we import the HeatMap
component and provide it with an array of data points. Each data point consists of a date and a count. The weekLabels
prop allows us to customize the day labels, while startDate
sets the beginning of our heatmap.
Customizing the Flame
Igniting with Color
One of the most powerful features of @uiw/react-heat-map is its color customization. Let’s see how we can adjust the color scheme to match our app’s theme:
import React from 'react';
import HeatMap from '@uiw/react-heat-map';
const value = [
{ date: '2023/01/11', count: 2 },
{ date: '2023/05/01', count: 5 },
{ date: '2023/05/02', count: 5 },
{ date: '2023/05/03', count: 1 },
{ date: '2023/05/04', count: 11 },
{ date: '2023/05/08', count: 32 },
];
const CustomColorHeatMap = () => {
return (
<HeatMap
value={value}
width={600}
style={{ color: '#ad001d', '--rhm-rect-active': 'red' }}
startDate={new Date('2023/01/01')}
panelColors={{
0: '#f4decd',
7: '#e4b293',
14: '#d48462',
21: '#c2533a',
28: '#ad001d',
35: '#6c0012'
}}
/>
);
};
export default CustomColorHeatMap;
Here, we’ve used the style
prop to set the base color and active rectangle color. The panelColors
prop allows us to define a custom color scale based on the count values.
Shaping the Flames
@uiw/react-heat-map also allows you to customize the shape of each day’s rectangle. Let’s create a heatmap with rounded corners:
import React, { useState } from 'react';
import HeatMap from '@uiw/react-heat-map';
const value = [
{ date: '2023/01/11', count: 2 },
// ... more data points
];
const RoundedHeatMap = () => {
const [range, setRange] = useState(5);
return (
<div>
<input
type="range"
min="0"
max="5"
step="0.1"
value={range}
onChange={(e) => setRange(parseFloat(e.target.value))}
/>
{range}
<HeatMap
value={value}
width={600}
style={{ '--rhm-rect': '#b9b9b9' }}
startDate={new Date('2023/01/01')}
legendRender={(props) => <rect {...props} y={props.y + 10} rx={range} />}
rectProps={{ rx: range }}
/>
</div>
);
};
export default RoundedHeatMap;
In this example, we’ve added an interactive slider that allows users to adjust the corner radius of the rectangles in real-time.
Advanced Techniques
Tooltip Integration
To provide more detailed information on hover, we can integrate tooltips with our heatmap:
import React from 'react';
import Tooltip from '@uiw/react-tooltip';
import HeatMap from '@uiw/react-heat-map';
const value = [
{ date: '2023/01/11', count: 2 },
// ... more data points
];
const TooltipHeatMap = () => {
return (
<HeatMap
value={value}
width={600}
startDate={new Date('2023/01/01')}
rectRender={(props, data) => {
return (
<Tooltip placement="top" content={`count: ${data.count || 0}`}>
<rect {...props} />
</Tooltip>
);
}}
/>
);
};
export default TooltipHeatMap;
This code uses the rectRender
prop to wrap each day’s rectangle in a Tooltip
component, displaying the count value on hover.
Selectable Heatmap
We can also create an interactive heatmap where users can select specific dates:
import React, { useState } from 'react';
import HeatMap from '@uiw/react-heat-map';
const value = [
{ date: '2023/01/11', count: 2 },
// ... more data points
];
const SelectableHeatMap = () => {
const [selected, setSelected] = useState('');
return (
<HeatMap
width={600}
value={value}
startDate={new Date('2023/01/01')}
rectRender={(props, data) => {
if (selected !== '') {
props.opacity = data.date === selected ? 1 : 0.45;
}
return (
<rect
{...props}
onClick={() => {
setSelected(data.date === selected ? '' : data.date);
}}
/>
);
}}
/>
);
};
export default SelectableHeatMap;
This implementation allows users to click on a date to highlight it, dimming all other dates in the process.
Fanning the Flames of Creativity
@uiw/react-heat-map provides a powerful and flexible foundation for creating stunning calendar heatmaps in your React applications. From basic usage to advanced customizations, this library offers a wide range of possibilities for visualizing time-based data.
Whether you’re building a personal productivity dashboard, a team activity tracker, or any application that benefits from temporal data visualization, @uiw/react-heat-map is an excellent choice. Its lightweight nature, customizable features, and ease of use make it a valuable addition to any React developer’s toolkit.
As you continue to explore and experiment with @uiw/react-heat-map, you’ll discover even more ways to tailor the component to your specific needs. The flame of creativity is in your hands – use it to ignite your React applications with compelling and informative calendar heatmaps!