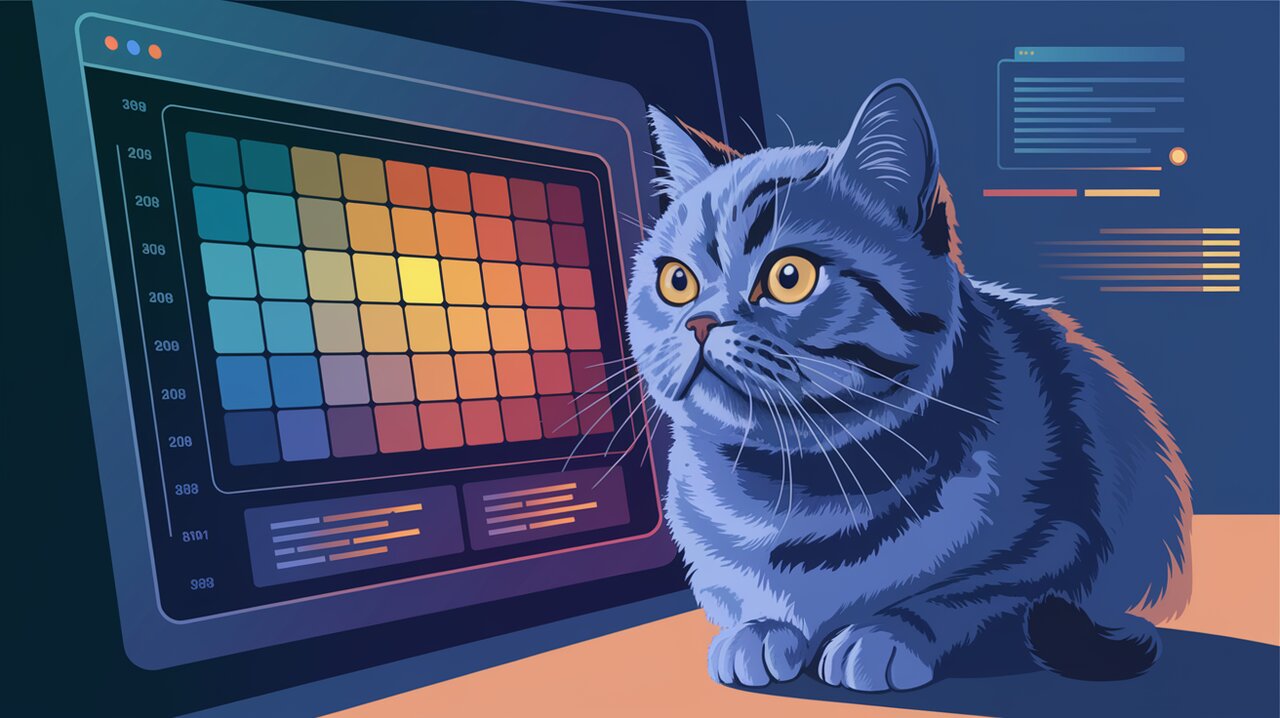
Sizzling Data Displays: Unleashing the Power of react-heatmap-grid
In the realm of data visualization, heatmaps stand out as a powerful tool for representing complex datasets in an easily digestible format. Enter react-heatmap-grid
, a React component that brings the magic of heatmaps to your web applications with ease and flexibility. Whether you’re tracking user engagement, analyzing sales patterns, or visualizing any other multi-dimensional data, this library has got you covered.
Igniting Your Data Visualization
react-heatmap-grid
offers a straightforward way to create heatmaps using simple div
elements. This approach ensures broad compatibility and performance across different browsers and devices. Let’s dive into the features that make this library a hot choice for React developers.
Key Features
- Easy Integration: Seamlessly integrates with your React projects.
- Customizable Appearance: Tailor the heatmap’s look to match your application’s design.
- Flexible Data Handling: Works with 2D arrays, making it versatile for various data structures.
- Interactive Elements: Add click handlers to cells for enhanced user interaction.
- Responsive Design: Adapts to different screen sizes and orientations.
Installing the Heat
Getting started with react-heatmap-grid
is as simple as installing any other npm package. You have two options:
Using yarn:
yarn add react-heatmap-grid
Or if you prefer npm:
npm install react-heatmap-grid --save
Firing Up Your First Heatmap
Let’s create a basic heatmap to showcase how easy it is to get started. Here’s a simple example:
import React from 'react';
import HeatMap from 'react-heatmap-grid';
const MyHeatmap: React.FC = () => {
const xLabels = new Array(24).fill(0).map((_, i) => `${i}`);
const yLabels = ['Sun', 'Mon', 'Tue'];
const data = new Array(yLabels.length)
.fill(0)
.map(() => new Array(xLabels.length).fill(0).map(() => Math.floor(Math.random() * 100)));
return (
<HeatMap
xLabels={xLabels}
yLabels={yLabels}
data={data}
/>
);
};
export default MyHeatmap;
This code creates a heatmap representing random data across three days and 24 hours. The xLabels
represent hours, yLabels
represent days, and data
is a 2D array of random values between 0 and 99.
Customizing Your Heatmap
One of the strengths of react-heatmap-grid
is its customizability. Let’s explore some advanced usage scenarios to make your heatmap truly shine.
Styling Cells
You can customize the appearance of each cell using the cellStyle
prop:
<HeatMap
xLabels={xLabels}
yLabels={yLabels}
data={data}
cellStyle={(background, value, min, max, data, x, y) => ({
background: `rgb(0, 151, 230, ${1 - (max - value) / (max - min)})`,
fontSize: '11px',
color: '#444',
})}
/>
This example changes the cell color based on its value relative to the minimum and maximum values in the dataset.
Adding Interactivity
Make your heatmap interactive by adding an onClick
handler:
<HeatMap
xLabels={xLabels}
yLabels={yLabels}
data={data}
onClick={(x, y) => alert(`Clicked ${x}, ${y}`)}
/>
Now, when a user clicks on a cell, they’ll see an alert with the cell’s coordinates.
Custom Cell Rendering
For more complex cell content, use the cellRender
prop:
<HeatMap
xLabels={xLabels}
yLabels={yLabels}
data={data}
cellRender={(value) => value && `${value}%`}
/>
This will display the cell value as a percentage when it’s non-zero.
Advanced Configurations
react-heatmap-grid
offers several configuration options to fine-tune your heatmap’s appearance and behavior:
- background: Set the base color for the heatmap (default: “#329fff”).
- height: Specify the height of each cell in pixels (default: 30).
- squares: Set to
true
to render cells as squares instead of rectangles. - xLabelWidth and yLabelWidth: Control the width of label areas.
- xLabelsLocation: Place x-axis labels at the “top” or “bottom” of the grid.
- xLabelsVisibility: An array of booleans to control which x-labels are displayed.
Here’s an example incorporating some of these advanced features:
<HeatMap
xLabels={xLabels}
yLabels={yLabels}
xLabelsLocation="bottom"
xLabelsVisibility={xLabels.map((_, i) => i % 2 === 0)}
xLabelWidth={50}
data={data}
squares
onClick={(x, y) => alert(`Clicked ${x}, ${y}`)}
cellStyle={(background, value, min, max, data, x, y) => ({
background: `rgba(66, 86, 244, ${1 - (max - value) / (max - min)})`,
fontSize: '11px',
})}
cellRender={(value) => value && `${value}%`}
title={(value, unit) => `${value} ${unit}`}
/>
This configuration creates a square-celled heatmap with bottom-aligned x-labels, showing only even-numbered labels, and custom styling and rendering for cells.
Conclusion
react-heatmap-grid
offers a powerful yet easy-to-use solution for creating heatmaps in React applications. Its flexibility allows for simple implementations as well as complex, customized visualizations. By leveraging this library, you can transform your data into insightful, visually appealing heatmaps that enhance user understanding and engagement.
Whether you’re building dashboards, analyzing trends, or simply want to add a touch of data visualization to your React project, react-heatmap-grid
provides the tools you need to create stunning heatmaps with ease.
For more React visualization adventures, check out our articles on Chart.js Symphony with react-chartjs-2 and Charting Adventures with React Google Charts. These complementary libraries can further enhance your data visualization toolkit, allowing you to create a wide range of charts and graphs alongside your heatmaps.
Happy heatmapping!