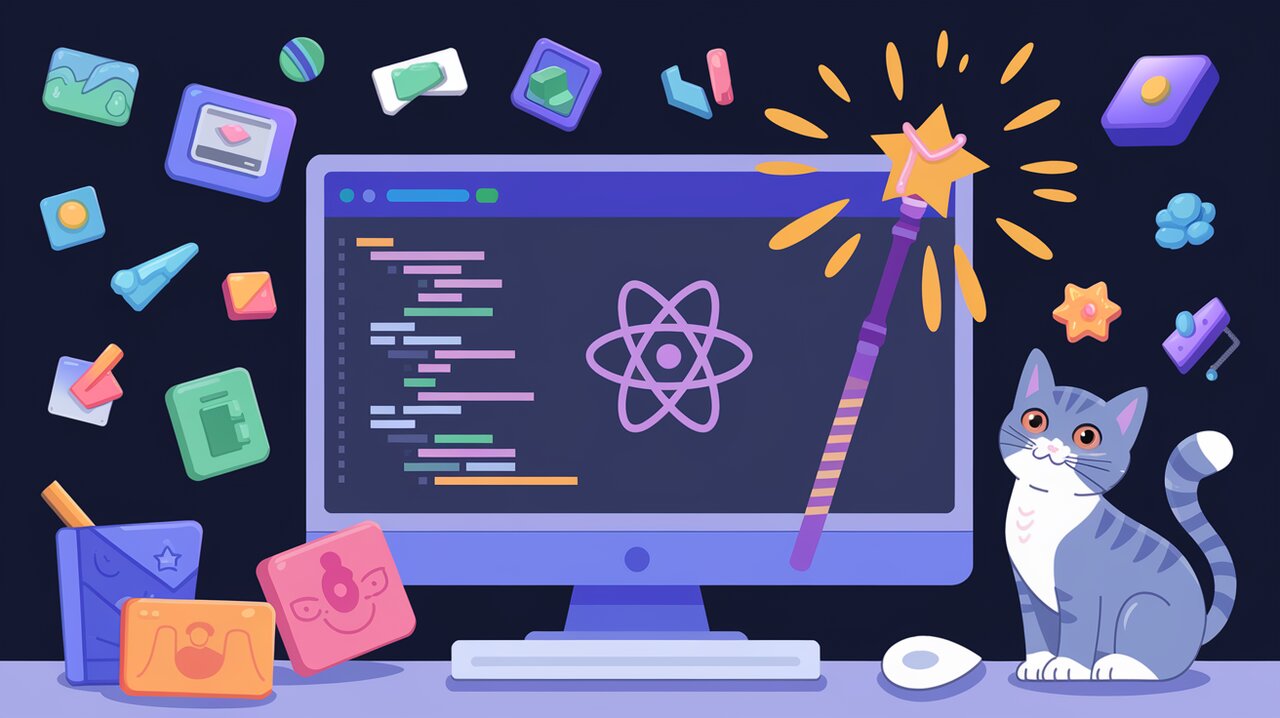
React-Icomoon is a powerful library that simplifies the process of integrating and customizing SVG icons in React and React Native projects. By leveraging the flexibility of SVG graphics and the component-based architecture of React, this library offers developers a seamless way to enhance their user interfaces with scalable, customizable icons.
Features
React-Icomoon comes packed with a set of features that make it a go-to solution for icon management in React applications:
- Easy Integration: Seamlessly incorporate SVG icons into your React and React Native projects.
- Customization: Easily modify icon properties such as size, color, and style.
- Scalability: Utilize vector graphics that maintain quality at any size.
- Performance: Optimize your application’s performance with efficient SVG rendering.
- Flexibility: Use with various icon sets, including custom-made icons.
Getting Started
To begin your journey with React-Icomoon, you’ll first need to install the library in your project.
Installation
You can install React-Icomoon using npm or yarn:
npm install react-icomoon
Or if you prefer yarn:
yarn add react-icomoon
Basic Usage
Once installed, you can start using React-Icomoon in your project. Here’s a simple example of how to set up and use the library:
import IcoMoon from "react-icomoon";
import iconSet from "./selection.json";
const Icon = (props) => <IcoMoon iconSet={iconSet} {...props} />;
export default Icon;
In this example, we’re importing the IcoMoon
component from the react-icomoon
package and our icon set (typically named selection.json
). We then create a custom Icon
component that wraps the IcoMoon
component, passing in our icon set and any additional props.
To use this Icon
component in your application, you can simply import it and use it like any other React component:
import Icon from "./Icon";
const MyComponent = () => (
<div>
<Icon icon="pencil" size={20} color="orange" />
</div>
);
This will render a pencil icon with a size of 20 pixels and an orange color.
Advanced Usage
React-Icomoon offers several advanced features that allow for greater customization and flexibility in your icon usage.
Customizing Icon Styles
You can easily customize the appearance of your icons by passing additional props:
<Icon
icon="star"
size={32}
color="#FFD700"
style={{ marginRight: "10px" }}
/>
This will render a gold star icon with a size of 32 pixels and a right margin of 10 pixels.
Using with React Native
React-Icomoon also supports React Native projects. To use it in a React Native environment, you’ll need to make a few adjustments:
import IcoMoon from "react-icomoon";
import { Svg, Path } from "react-native-svg";
import iconSet from "./selection.json";
const Icon = (props) => (
<IcoMoon
native
SvgComponent={Svg}
PathComponent={Path}
iconSet={iconSet}
{...props}
/>
);
export default Icon;
In this setup, we’re using the native
prop and providing Svg
and Path
components from the react-native-svg
library.
Retrieving Icon List
React-Icomoon provides a utility function to retrieve a list of all available icons in your icon set:
import { iconList } from "react-icomoon";
import iconSet from "./selection.json";
const availableIcons = iconList(iconSet);
console.log(availableIcons);
This can be useful for dynamically generating icon pickers or validating icon names in your application.
Conclusion
React-Icomoon offers a powerful and flexible solution for integrating SVG icons into React and React Native projects. Its ease of use, customization options, and support for both web and mobile platforms make it an excellent choice for developers looking to enhance their user interfaces with high-quality, scalable icons.
By leveraging React-Icomoon, you can significantly streamline your icon management process, allowing you to focus on creating beautiful and intuitive user interfaces. Whether you’re working on a small project or a large-scale application, React-Icomoon provides the tools you need to bring your designs to life with ease and efficiency.
For more insights on enhancing your React applications, check out our articles on Iconify React: Icon Extravaganza and Mastering React Icons Library. These resources will further expand your toolkit for creating visually appealing and user-friendly interfaces in React.