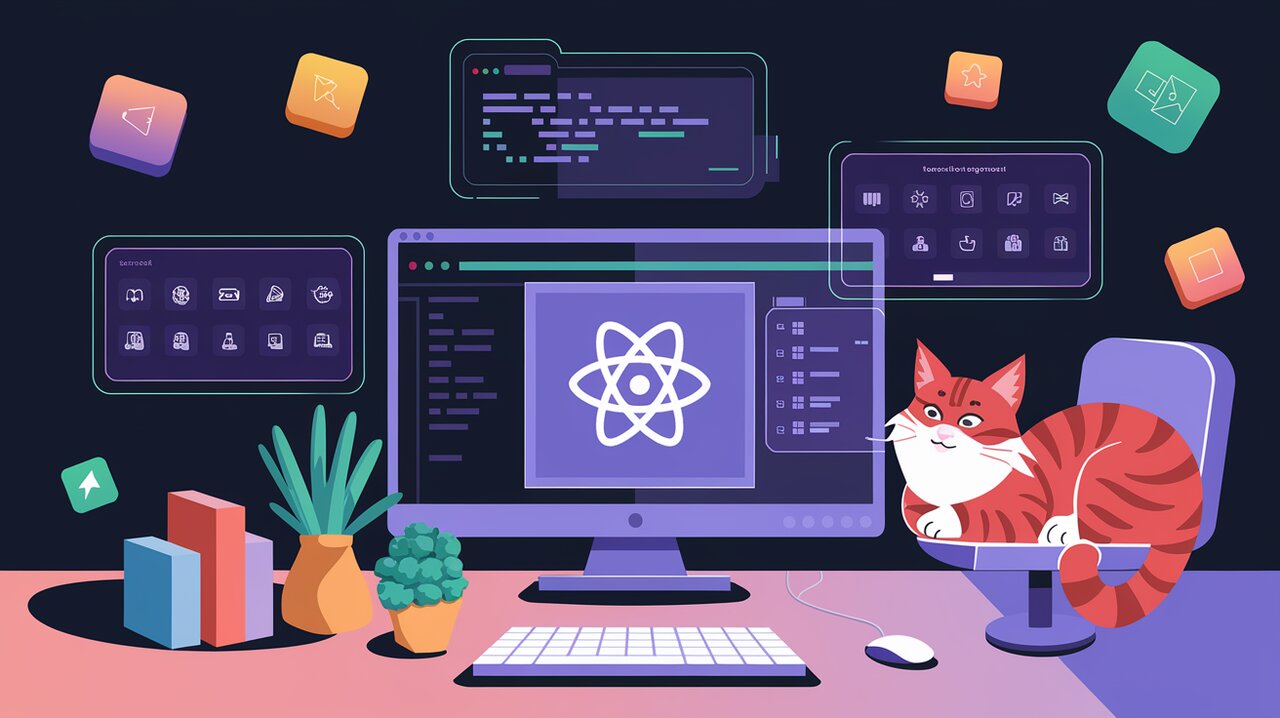
Unleash the Icon Extravaganza with @iconify/react
In the ever-evolving landscape of web development, icons play a crucial role in enhancing user interfaces and improving user experience. Enter @iconify/react, a game-changing library that brings an extensive universe of icons to your React applications. With its vast collection and dynamic loading capabilities, @iconify/react is revolutionizing how developers integrate and manage icons in their projects.
Unveiling the Icon Treasure Trove
@iconify/react is not just another icon library; it’s a comprehensive icon framework that offers unparalleled flexibility and choice. Let’s explore the key features that make this library stand out:
-
Massive Icon Collection: With over 200,000 open-source icons from more than 150 icon sets, you’ll never run out of options.
-
On-Demand Loading: Icons are loaded dynamically from the Iconify API, ensuring optimal performance and reducing bundle size.
-
Framework Agnostic: While we focus on React, Iconify supports various frameworks and can be used as a web component.
-
Customization: Easily customize icons with props for color, size, and more.
-
TypeScript Support: Enjoy full TypeScript support for a better development experience.
Getting Started with @iconify/react
Let’s dive into how you can start using @iconify/react in your React projects.
Installation
First, you need to install the package. You can do this using npm or yarn:
npm install @iconify/react
# or
yarn add @iconify/react
Basic Usage
Once installed, you can start using icons in your React components. Here’s a simple example:
import React from 'react';
import { Icon } from '@iconify/react';
const MyComponent: React.FC = () => {
return (
<div>
<h1>Welcome to My App</h1>
<Icon icon="mdi:home" />
</div>
);
};
export default MyComponent;
In this example, we’re using the ‘home’ icon from the Material Design Icons set. The Icon
component automatically loads the icon data from the Iconify API when it’s needed.
Customizing Icons
One of the strengths of @iconify/react is its customization capabilities. Let’s explore some ways to tailor icons to your needs.
Changing Size and Color
You can easily adjust the size and color of icons using props:
<Icon icon="mdi:star" color="gold" width="32" height="32" />
This will render a gold star icon with a width and height of 32 pixels.
Applying CSS Styles
For more advanced styling, you can use the style
prop or external CSS:
<Icon
icon="mdi:heart"
style={{ color: 'red', fontSize: '24px' }}
/>
Advanced Usage
Let’s delve into some more advanced features of @iconify/react.
Using Icon Sets
While you can use individual icons, @iconify/react also allows you to use entire icon sets. This can be particularly useful for theming or maintaining consistency across your application.
import { Icon, IconifyProvider } from '@iconify/react';
import mdiSet from '@iconify/icons-mdi';
const App: React.FC = () => {
return (
<IconifyProvider defaultSet="mdi" icons={mdiSet}>
<div>
<Icon icon="home" />
<Icon icon="star" />
<Icon icon="settings" />
</div>
</IconifyProvider>
);
};
In this example, we’re using the Material Design Icons set as our default, allowing us to reference icons without specifying the set each time.
Offline Usage
While @iconify/react typically loads icons from the API, you can also use it offline by bundling the icons you need:
import { Icon } from '@iconify/react';
import homeIcon from '@iconify-icons/mdi/home';
const OfflineComponent: React.FC = () => {
return <Icon icon={homeIcon} />;
};
This approach is useful when you want to ensure specific icons are always available, even without an internet connection.
Performance Considerations
One of the key advantages of @iconify/react is its performance optimization. By loading icons on-demand, it significantly reduces the initial bundle size of your application. However, there are a few tips to further optimize performance:
-
Preload Frequently Used Icons: For icons that appear on every page, consider preloading them to avoid any delay in rendering.
-
Use Icon Components: For frequently used icons, create dedicated components to improve reusability and potentially optimize rendering.
-
Lazy Loading: For pages with many icons, consider lazy loading icons that are not immediately visible.
Conclusion
@iconify/react offers a powerful and flexible solution for integrating icons into your React applications. Its vast library, coupled with dynamic loading and customization options, makes it an invaluable tool for modern web development. By leveraging its features, you can enhance your UI design process and create more engaging user experiences.
As you explore the world of icons with @iconify/react, you might also be interested in other React UI enhancements. Check out our articles on Animate with Framer Motion for adding smooth animations to your React components, or CoreUI React UI Symphony for a comprehensive UI component library that complements your icon usage.
Remember, the key to great UI design is not just in the individual elements, but in how they come together to create a cohesive and intuitive user experience. With @iconify/react, you’re well-equipped to bring your interface designs to life with a touch of iconographic magic.