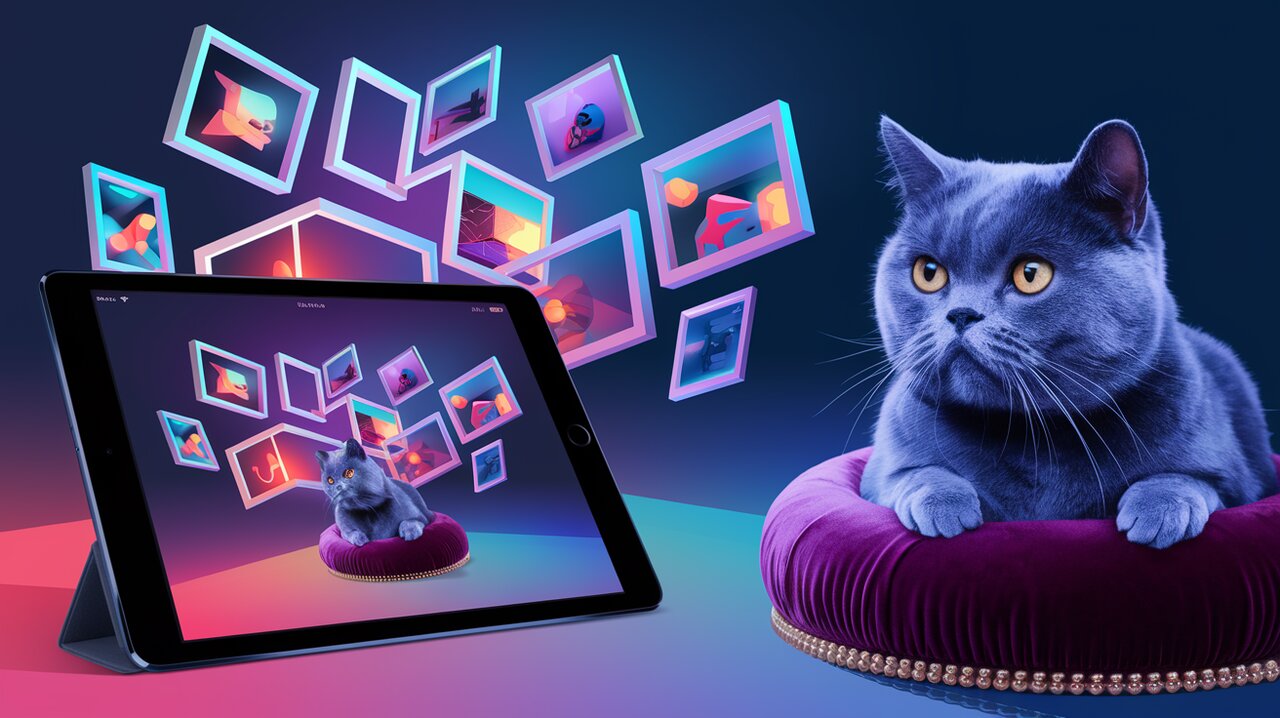
Illuminating React Galleries: Mastering lightGallery for Stunning Visual Experiences
Illuminating Your React Projects with lightGallery
In the world of web development, creating engaging and interactive image and video galleries is crucial for delivering a captivating user experience. lightGallery emerges as a powerful solution for React developers, offering a feature-rich, customizable, and responsive gallery component that brings your visual content to life.
Key Features of lightGallery
lightGallery boasts an impressive array of features that set it apart:
- Fully responsive design
- Touch-enabled for mobile devices
- Support for images, videos, and iframes
- Customizable animations and transitions
- Social sharing capabilities
- Thumbnail navigation
- Zoom functionality
- Fullscreen mode
- Keyboard navigation
- Accessibility support
Getting Started with lightGallery in React
Installation
To begin using lightGallery in your React project, you’ll need to install the package and its plugins. Open your terminal and run:
npm install lightgallery lg-zoom lg-thumbnail
Or if you prefer using Yarn:
yarn add lightgallery lg-zoom lg-thumbnail
Basic Usage
Let’s create a simple image gallery using lightGallery. First, import the necessary components and styles:
import React from 'react';
import LightGallery from 'lightgallery/react';
// import styles
import 'lightgallery/css/lightgallery.css';
import 'lightgallery/css/lg-zoom.css';
import 'lightgallery/css/lg-thumbnail.css';
// import plugins if you need
import lgThumbnail from 'lightgallery/plugins/thumbnail';
import lgZoom from 'lightgallery/plugins/zoom';
Now, let’s create a functional component that renders our gallery:
const Gallery = () => {
const onInit = () => {
console.log('lightGallery has been initialized');
};
return (
<div className="App">
<LightGallery
onInit={onInit}
speed={500}
plugins={[lgThumbnail, lgZoom]}
>
<a href="https://picsum.photos/1024/768?image=1">
<img alt="img1" src="https://picsum.photos/200/300?image=1" />
</a>
<a href="https://picsum.photos/1024/768?image=2">
<img alt="img2" src="https://picsum.photos/200/300?image=2" />
</a>
<a href="https://picsum.photos/1024/768?image=3">
<img alt="img3" src="https://picsum.photos/200/300?image=3" />
</a>
</LightGallery>
</div>
);
};
This basic setup creates a gallery with three images, utilizing the zoom and thumbnail plugins.
Advanced Usage and Customization
Dynamic Content Loading
lightGallery supports dynamic content loading, which is perfect for scenarios where you need to load gallery items from an API or other dynamic sources:
const DynamicGallery = () => {
const [items, setItems] = useState([]);
useEffect(() => {
// Simulating an API call
const fetchItems = async () => {
const response = await fetch('https://api.example.com/gallery-items');
const data = await response.json();
setItems(data);
};
fetchItems();
}, []);
return (
<LightGallery mode="lg-fade" plugins={[lgZoom, lgThumbnail]}>
{items.map((item, index) => (
<a key={index} href={item.src}>
<img alt={item.alt} src={item.thumb} />
</a>
))}
</LightGallery>
);
};
Custom Thumbnails
You can create custom thumbnails for your gallery items, which is particularly useful for video content:
const VideoGallery = () => {
return (
<LightGallery plugins={[lgThumbnail, lgZoom]}>
<a
data-lg-size="1280-720"
data-src="https://www.youtube.com/watch?v=dQw4w9WgXcQ"
data-poster="https://img.youtube.com/vi/dQw4w9WgXcQ/maxresdefault.jpg"
data-sub-html="<h4>Never Gonna Give You Up - Rick Astley</h4><p>The classic music video</p>"
>
<img
alt="Video thumbnail"
src="https://img.youtube.com/vi/dQw4w9WgXcQ/maxresdefault.jpg"
style={{ maxWidth: '400px' }}
/>
</a>
</LightGallery>
);
};
Customizing Animations and Transitions
lightGallery offers various animation options. You can easily customize the transition effects:
const AnimatedGallery = () => {
return (
<LightGallery
mode="lg-fade"
cssEasing="cubic-bezier(0.25, 0, 0.25, 1)"
speed={500}
plugins={[lgZoom, lgThumbnail]}
>
{/* Gallery items */}
</LightGallery>
);
};
Optimizing Performance
To ensure your gallery performs well, especially with a large number of items, consider the following tips:
- Use appropriate image sizes and formats (WebP for modern browsers).
- Implement lazy loading for thumbnails.
- Utilize the
preload
plugin to preload adjacent slides.
Here’s an example of implementing lazy loading:
const LazyLoadGallery = () => {
return (
<LightGallery plugins={[lgZoom, lgThumbnail]}>
{galleryItems.map((item, index) => (
<a key={index} href={item.src}>
<img
alt={item.alt}
data-src={item.thumb}
className="lazyload"
/>
</a>
))}
</LightGallery>
);
};
Accessibility Considerations
lightGallery provides built-in accessibility features, but it’s important to enhance them for the best user experience:
- Use descriptive
alt
texts for images. - Implement keyboard navigation.
- Ensure sufficient color contrast for UI elements.
Conclusion
lightGallery offers React developers a powerful toolkit for creating stunning, interactive galleries. From basic image displays to complex video galleries with custom thumbnails, this library provides the flexibility and features needed to enhance any project’s visual appeal. By leveraging its extensive customization options and keeping performance and accessibility in mind, you can create engaging gallery experiences that captivate your users and elevate your React applications.