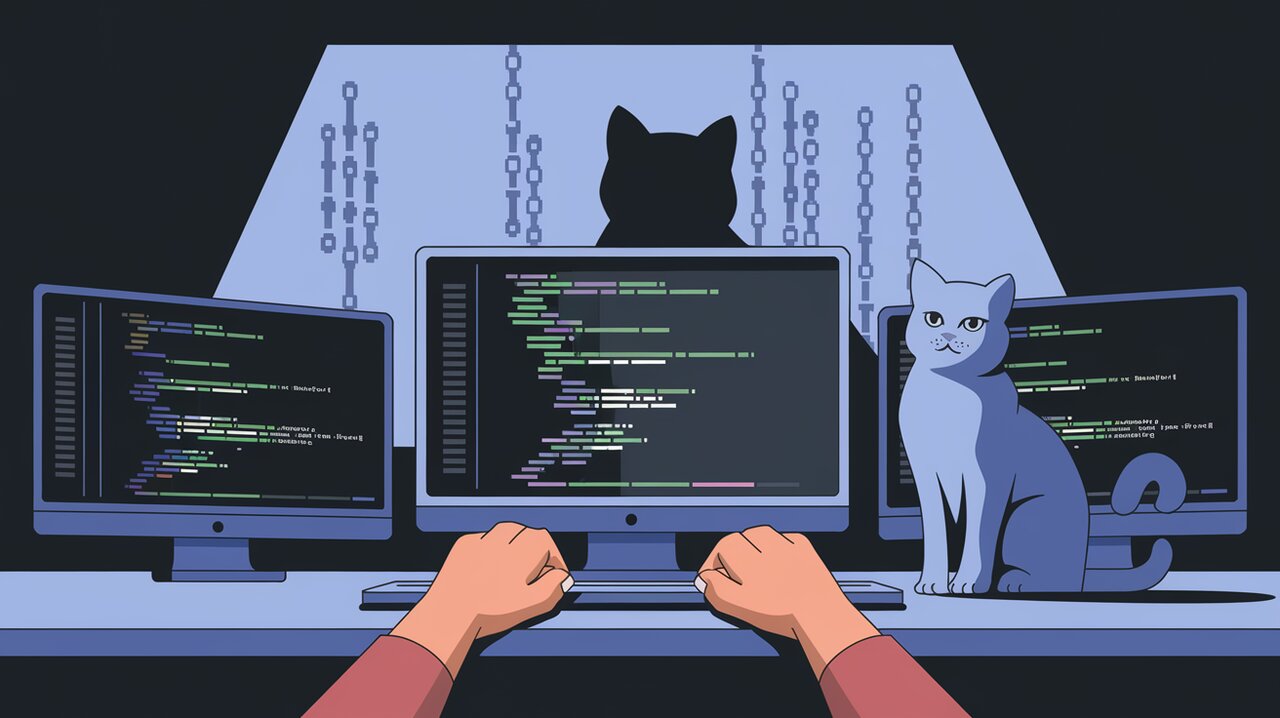
Illuminating the Shadows: Mastering React-Shadow for Style Encapsulation
Introduction
In the ever-evolving landscape of web development, the need for modular, encapsulated components has become increasingly crucial. React developers often face challenges when it comes to style isolation and preventing CSS conflicts across different parts of their applications. Enter react-shadow
, a powerful library that brings the capabilities of Shadow DOM to the React ecosystem, offering a solution to these common pain points.
Features
react-shadow
boasts several key features that make it an invaluable tool for React developers:
- Shadow DOM Integration: Seamlessly create shadow roots for React components.
- Style Encapsulation: Isolate styles within components, preventing CSS conflicts.
- Flexible Usage: Support for various styling methods, including CSS imports and styled-components.
- SSR Compatibility: Experimental support for server-side rendering with shadow DOM.
- Customizable Shadow Root: Fine-grained control over shadow root properties.
Installation
To get started with react-shadow
, you can install it using npm or yarn:
npm install react-shadow
or
yarn add react-shadow
Basic Usage
Creating a Shadow Root Component
The most fundamental use of react-shadow
involves creating a shadow root for your React component. Here’s a simple example:
import root from 'react-shadow';
import styles from './styles.css';
export default function Quote() {
return (
<root.div className="quote">
<q>There is strong shadow where there is much light.</q>
<span className="author">― Johann Wolfgang von Goethe.</span>
<style type="text/css">{styles}</style>
</root.div>
);
}
In this example, we create a shadow root using root.div
. All child elements are placed within the shadow boundary, and styles are encapsulated.
Applying Styles
react-shadow
offers flexibility in how you can apply styles to your shadow DOM components. You can either include styles directly as a string or import CSS files as strings. For the latter approach, you might need to configure your build process. For instance, with Webpack, you could use:
{
test: /\.css$/,
loader: ['to-string-loader', 'css-loader']
}
This configuration allows you to import CSS files as strings and apply them within your shadow DOM.
Advanced Usage
Using Styled-Components
react-shadow
seamlessly integrates with popular CSS-in-JS libraries like styled-components. To use styled-components with react-shadow
, you can import from a special entry point:
import root from 'react-shadow/styled-components';
// Use styled-components as usual within your shadow root
This integration creates a new StyleSheetManager
context for each shadow boundary, ensuring that styles remain encapsulated.
Customizing Shadow Root Properties
react-shadow
allows you to customize the properties of the shadow root. You can control aspects such as the mode and focus delegation:
<root.div mode="closed" delegatesFocus={true}>
{/* Your component content */}
</root.div>
These props correspond to the options you would pass to attachShadow
in vanilla JavaScript.
Accessing the Shadow Root
In some cases, you might need to access the shadow root directly. You can do this using a ref:
const node = useRef(null);
// ...
<root.section ref={node} />
// Later, you can access the shadow root with:
// node.current.shadowRoot
This can be useful for advanced manipulations or when you need to interact with the shadow DOM programmatically.
Experimental SSR Support
react-shadow
offers experimental support for server-side rendering with shadow DOM. While this feature is still in development and may have limitations, you can enable it using the ssr
prop:
<root.section ssr>
{/* Your component content */}
</root.section>
This feature aims to provide a solution for applications that require server-side rendering while still benefiting from shadow DOM encapsulation.
Conclusion
react-shadow
is a powerful tool that brings the benefits of Shadow DOM to the React ecosystem. By providing robust style encapsulation and component isolation, it addresses common challenges in large-scale React applications. Whether you’re building a complex application with numerous components or simply want to ensure style isolation in specific parts of your app, react-shadow
offers a flexible and efficient solution.
As web development continues to evolve, tools like react-shadow
play a crucial role in enabling developers to create more modular, maintainable, and performant applications. By mastering react-shadow
, you can take your React development skills to the next level, creating truly encapsulated and reusable components.
Remember to keep an eye on the project’s documentation and updates, as the Shadow DOM specification and its implementation in browsers continue to evolve. With react-shadow
in your toolkit, you’re well-equipped to tackle the challenges of modern web development and create robust, scalable React applications.