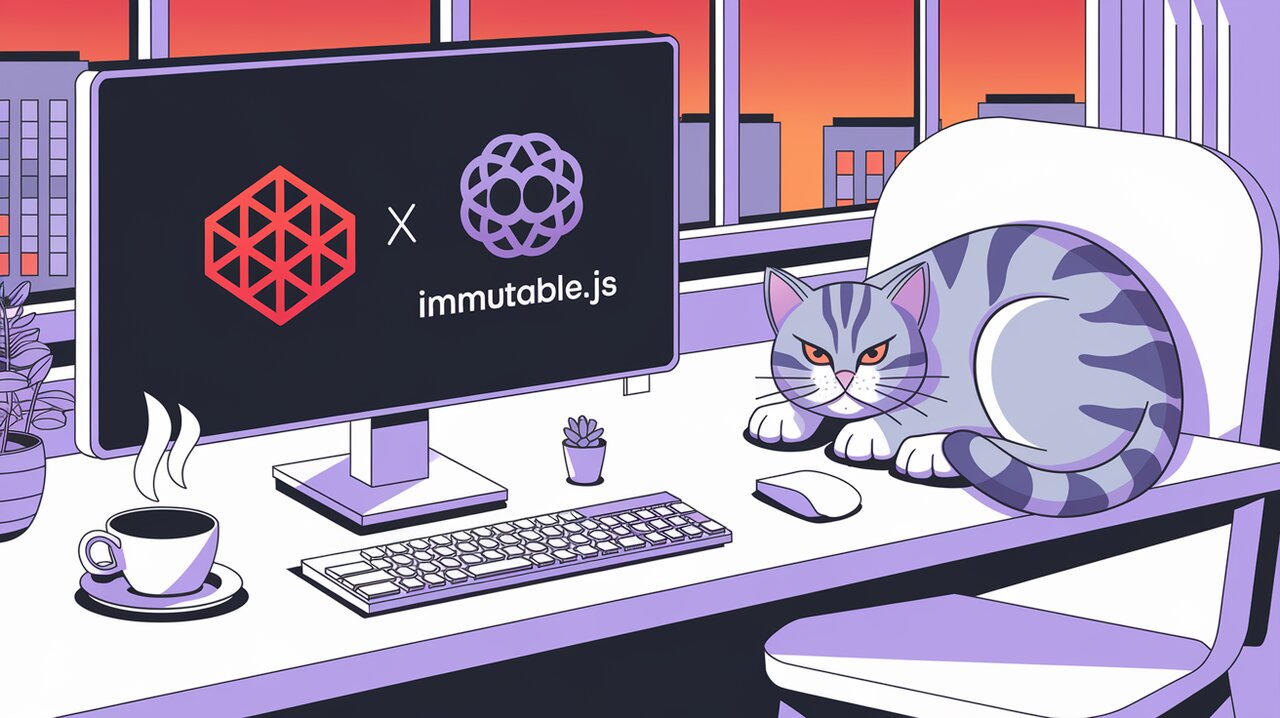
Harmonizing Redux and Immutable.js: The Magic of redux-immutable-utils
In the ever-evolving landscape of React development, managing state efficiently is crucial for building performant and maintainable applications. While Redux has long been a go-to solution for state management, combining it with Immutable.js can lead to even more robust and predictable applications. Enter redux-immutable-utils, a lightweight library that seamlessly integrates these two powerful tools, offering developers a streamlined approach to handling immutable state in Redux applications.
Bridging the Gap: Redux and Immutable.js
redux-immutable-utils is designed to simplify the process of using Immutable.js with Redux. It provides utility functions that allow developers to leverage the benefits of immutable data structures within their Redux workflow, without the need for complex boilerplate code or manual conversions between plain JavaScript objects and Immutable.js data types.
Key Features
- Seamless Integration: Easily combine Redux reducers into an Immutable Map.
- Type Safety: Full TypeScript support for enhanced developer experience.
- Minimal Overhead: Lightweight implementation with no additional dependencies.
- Flexibility: Makes no assumptions about your reducers, allowing for custom implementations.
Getting Started
Installation
To start using redux-immutable-utils in your project, you can install it via npm or yarn:
npm install redux-immutable-utils
Or if you prefer yarn:
yarn add redux-immutable-utils
Basic Usage: Combining Reducers
The core functionality of redux-immutable-utils revolves around its combineReducers
function. This function is similar to Redux’s native combineReducers
, but it creates an Immutable Map instead of a plain JavaScript object.
Here’s a simple example of how to use it:
import { combineReducers } from 'redux-immutable-utils';
import { Map } from 'immutable';
// Define your reducers
const userReducer = (state = Map(), action) => {
switch (action.type) {
case 'SET_USER':
return state.set('name', action.payload);
default:
return state;
}
};
const todoReducer = (state = Map(), action) => {
switch (action.type) {
case 'ADD_TODO':
return state.update('todos', todos => todos.push(action.payload));
default:
return state;
}
};
// Combine reducers
const rootReducer = combineReducers({
user: userReducer,
todos: todoReducer
});
In this example, we define two reducers: userReducer
and todoReducer
. Each reducer operates on its own Immutable Map. The combineReducers
function from redux-immutable-utils then combines these reducers into a single root reducer, where the state is an Immutable Map with user
and todos
as its keys.
Advanced Usage: Custom Reducer Combination
One of the strengths of redux-immutable-utils is its flexibility. You’re not limited to just combining reducers into a Map. You can create custom combinations to suit your application’s needs.
Creating a Record-based State
If you prefer a more structured state, you can use Immutable.js Records:
import { combineReducers } from 'redux-immutable-utils';
import { Record } from 'immutable';
// Define a Record for your state structure
const StateRecord = Record({
user: Map(),
todos: List()
});
// Create reducers that work with specific parts of the state
const userReducer = (state = Map(), action) => {
// ... user reducer logic
};
const todoReducer = (state = List(), action) => {
// ... todo reducer logic
};
// Combine reducers into a Record-based state
const rootReducer = combineReducers({
user: userReducer,
todos: todoReducer
}, StateRecord);
This approach gives you the benefits of type checking and default values provided by Immutable.js Records, while still allowing you to use the convenient combineReducers
function from redux-immutable-utils.
Integrating with Redux Store
To use your Immutable.js-based root reducer with Redux, you’ll need to ensure that your store is configured correctly:
import { createStore } from 'redux';
import { Map } from 'immutable';
const initialState = Map();
const store = createStore(rootReducer, initialState);
Remember that since your state is now an Immutable.js structure, you’ll need to use Immutable.js methods to access and update the state in your components and selectors.
Performance Considerations
Using Immutable.js with Redux can lead to significant performance improvements, especially in large applications. redux-immutable-utils helps you achieve these benefits with minimal overhead:
- Efficient Updates: Immutable.js uses structural sharing, which means that only the parts of the state that actually change are updated, leading to more efficient memory usage.
- Fast Equality Checks: Immutable.js provides fast equality checks for its data structures, which can speed up the process of determining whether a component needs to re-render.
Best Practices
When using redux-immutable-utils, keep these best practices in mind:
- Consistent Usage: Use Immutable.js data structures consistently throughout your application to get the full benefits.
- Selector Functions: Create selector functions that understand your Immutable.js state structure for efficient data retrieval.
- Type Definitions: If using TypeScript, define proper types for your state structure to catch errors early in development.
Conclusion
redux-immutable-utils provides a simple yet powerful way to integrate Immutable.js with Redux in your React applications. By leveraging this library, you can enjoy the benefits of immutable state management without sacrificing the familiarity and ecosystem of Redux. Whether you’re building a small project or a large-scale application, redux-immutable-utils offers the tools you need to create more predictable, performant, and maintainable state management solutions.
As you continue to explore the capabilities of redux-immutable-utils, you’ll discover how it can streamline your development process and help you build more robust React applications. Happy coding!