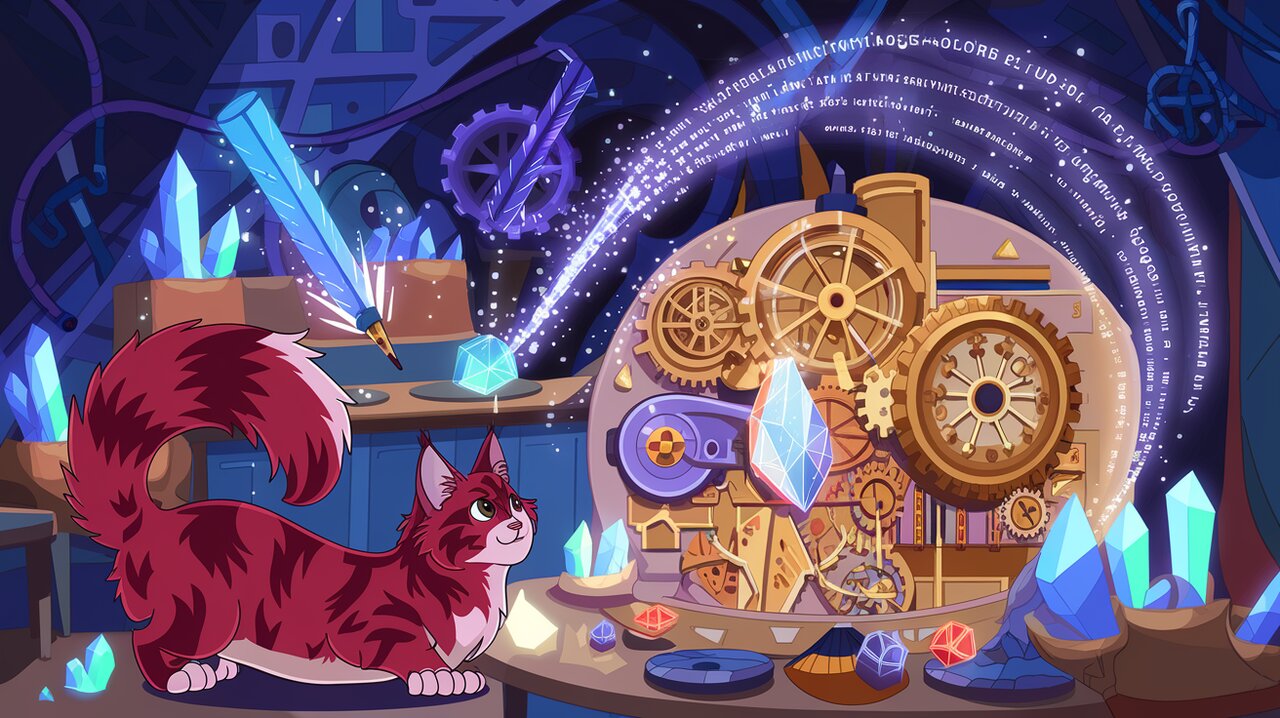
Immutable State Magic with react-copy-write: Simplify Your React State Management
In the ever-evolving landscape of React state management, developers are constantly seeking solutions that offer both simplicity and performance. Enter react-copy-write, a library that brings the best of both worlds: the benefits of immutable state with the ease of a mutable API. This innovative approach to state management in React applications promises to simplify your code while maintaining the performance advantages of immutability.
The Magic Behind the Curtain
At its core, react-copy-write leverages the power of Immer, a library that implements the copy-on-write technique. This allows developers to write state updates as if they were directly mutating the state, while behind the scenes, an immutable update is performed. The result? Clean, intuitive code that doesn’t sacrifice the benefits of immutability.
Conjuring the State
To begin your journey with react-copy-write, you’ll first need to install it in your project. Open your terminal and cast one of these spells:
npm install react-copy-write
# or
yarn add react-copy-write
Once installed, you’re ready to create your first state container. Let’s dive into the basic usage and see how react-copy-write can transform your state management approach.
Crafting Your First Spell
Creating the State
To get started, import createState
from react-copy-write and define your initial state:
import createState from 'react-copy-write';
const { Provider, Consumer, createSelector, mutate } = createState({
user: { name: 'Merlin', spells: [] }
});
This incantation creates a state container with a Provider
, Consumer
, createSelector
function, and a mutate
function. These tools will be the cornerstone of your state management magic.
Providing the State
To make your state available throughout your application, wrap your main component with the Provider
:
const App = () => (
<Provider>
<WizardrySchool />
</Provider>
);
If you need to initialize state from props, you can use the initialState
prop:
const App = ({ initialWizard }) => (
<Provider initialState={{ user: initialWizard }}>
<WizardrySchool />
</Provider>
);
Consuming the State
Now, let’s see how we can access our state within components:
const WizardProfile = () => (
<Consumer>
{state => (
<div>
<h1>{state.user.name}</h1>
<p>Spells known: {state.user.spells.length}</p>
</div>
)}
</Consumer>
);
The Consumer
component uses a render prop to provide access to the state. By default, it gives you the entire state tree, but we can optimize this further.
Advanced Incantations
Selective State Consumption
To prevent unnecessary re-renders, use the select
prop to specify which parts of the state your component needs:
const SpellCounter = () => (
<Consumer select={[state => state.user.spells.length]}>
{spellCount => <p>Spells known: {spellCount}</p>}
</Consumer>
);
This ensures that SpellCounter
only re-renders when the number of spells changes, not on every state update.
Mutating State with Elegance
One of the most powerful features of react-copy-write is its ability to update state using simple mutations. Here’s how you can add a new spell to our wizard’s repertoire:
const learnSpell = (spellName) => {
mutate(draft => {
draft.user.spells.push(spellName);
});
};
Despite appearances, this doesn’t actually mutate your original state. Instead, react-copy-write uses Immer to create a new immutable state based on these “mutations”.
Crafting Optimized Selectors
For frequently used state selections, you can create optimized selectors:
const selectUserName = createSelector(state => state.user.name);
const selectSpells = createSelector(state => state.user.spells);
const WizardSummary = () => (
<Consumer select={[selectUserName, selectSpells]}>
{(name, spells) => (
<div>
<h2>{name}</h2>
<ul>
{spells.map(spell => <li key={spell}>{spell}</li>)}
</ul>
</div>
)}
</Consumer>
);
By defining selectors outside of the render function and using them consistently, you can significantly optimize your application’s performance.
Mastering Complex State Updates
As your application grows, you might need to perform more complex state updates. react-copy-write shines in these scenarios as well:
Updating Nested State
Let’s say we want to add a level property to each spell and update it:
const levelUpSpell = (spellName) => {
mutate(draft => {
const spell = draft.user.spells.find(s => s.name === spellName);
if (spell) {
spell.level = (spell.level || 0) + 1;
}
});
};
Conditional Updates
You can even include complex logic within your mutations:
const attemptToLearnSpell = (spellName, difficultyLevel) => {
mutate(draft => {
if (draft.user.intelligence > difficultyLevel) {
draft.user.spells.push({ name: spellName, level: 1 });
draft.user.experience += 10;
} else {
draft.user.failedAttempts = (draft.user.failedAttempts || 0) + 1;
}
});
};
The Grand Finale
react-copy-write offers a unique blend of simplicity and power in React state management. By providing a mutable API for immutable updates, it allows developers to write intuitive code while reaping the benefits of immutability. The library’s use of structural sharing and memoization ensures that your application remains performant, even as it grows in complexity.
Whether you’re building a small wizard’s apprentice tracker or a grand magical realm management system, react-copy-write equips you with the tools to manage your state effectively. Its seamless integration with React’s context API, combined with the power of optimized selectors, makes it a formidable choice for modern React applications.
As you continue your journey in the realm of React development, consider adding react-copy-write to your spellbook of state management solutions. Its ability to simplify complex state logic while maintaining performance might just be the magic touch your next project needs.
For those looking to dive deeper into React state management, you might also be interested in exploring other powerful libraries like Zustand for simplified React state management or XState for orchestrating state machines in React. Each offers its own unique approach to taming the complexities of state in React applications.