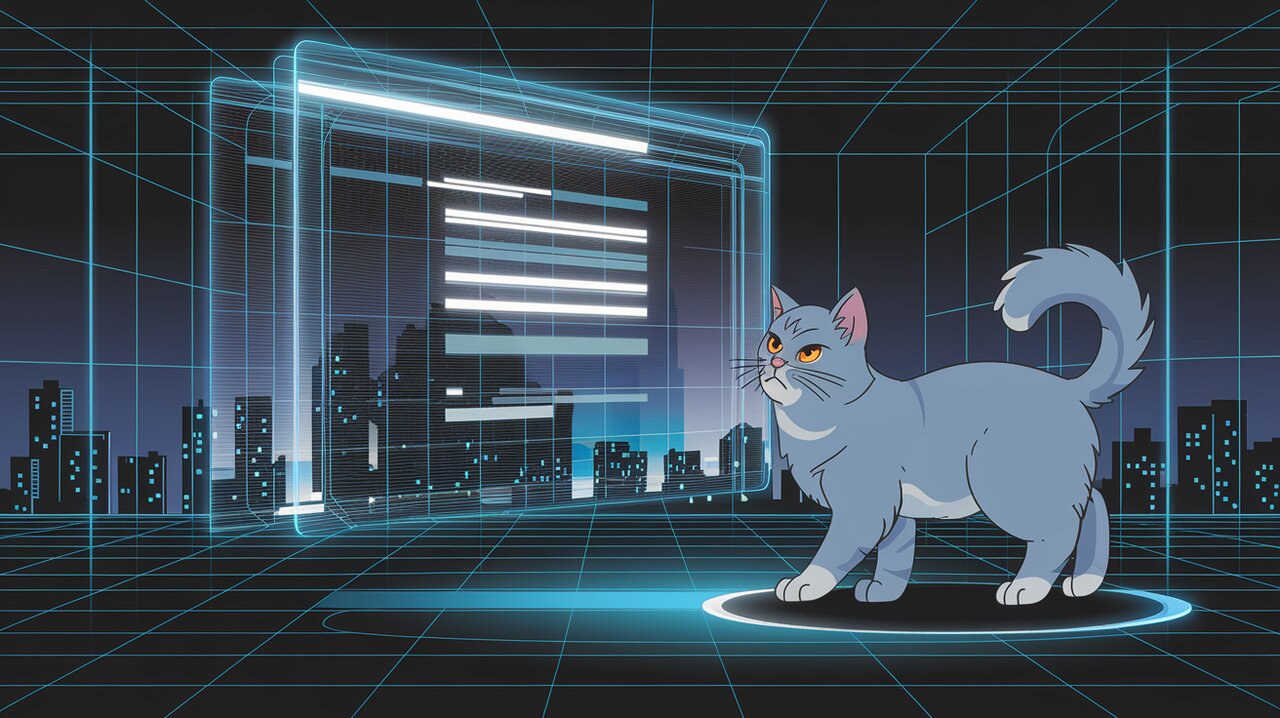
Unleash Infinite Scrolling Power with react-infinite-grid
In the realm of React development, handling large datasets efficiently is a common challenge. Enter react-infinite-grid, a powerful solution that brings infinite scrolling capabilities to your React applications. This ingenious component renders only the elements visible to the user, along with a small buffer, making it an ideal choice for displaying vast numbers of items without compromising performance.
Unveiling the Magic of react-infinite-grid
react-infinite-grid shines in scenarios where you need to present an extensive list or grid of elements. Whether you’re building a photo gallery with thousands of images, a product catalog, or a social media feed, this library ensures smooth scrolling and optimal resource utilization.
Key Features That Set react-infinite-grid Apart
- Efficient Rendering: Only renders visible elements and a small buffer, drastically reducing DOM nodes.
- Seamless Scrolling: Provides a smooth, infinite scrolling experience.
- Flexible Sizing: Customizable item dimensions and padding.
- Lazy Loading Support: Built-in callback for implementing lazy loading of data.
- React-Friendly: Seamlessly integrates with your React components.
Getting Started with react-infinite-grid
Let’s dive into how you can harness the power of react-infinite-grid
in your projects.
Installation
First, let’s add the library to your project. You can install it using npm or yarn:
npm install react-infinite-grid
Or if you prefer yarn:
yarn add react-infinite-grid
Basic Implementation: Creating Your First Infinite Grid
Let’s create a simple infinite grid with 100,000 items to demonstrate the library’s capabilities:
import React from 'react';
import ReactDOM from 'react-dom';
import InfiniteGrid from 'react-infinite-grid';
interface ExampleItemProps {
index: number;
}
const ExampleItem: React.FC<ExampleItemProps> = ({ index }) => {
return (
<div className='example'>
This is item {index}
</div>
);
};
const App: React.FC = () => {
// Create 100,000 Example items
const items = Array.from({ length: 100000 }, (_, i) => (
<ExampleItem key={i} index={i} />
));
return (
<InfiniteGrid
itemClassName="item"
entries={items}
/>
);
};
ReactDOM.render(<App />, document.getElementById('root'));
In this example, we’ve created a grid with 100,000 items. Despite the large number, react-infinite-grid
ensures that only the visible items are rendered, maintaining smooth performance.
Customizing Your Grid
react-infinite-grid
offers several props to tailor the grid to your needs:
import React from 'react';
import InfiniteGrid from 'react-infinite-grid';
const CustomizedGrid: React.FC = () => {
const items = // ... your items array
return (
<InfiniteGrid
entries={items}
height={200}
width={150}
padding={10}
wrapperHeight={600}
lazyCallback={() => console.log('Reached the end!')}
/>
);
};
This setup creates a grid with items of 200px height and 150px width, with 10px padding. The entire grid wrapper has a height of 600px, and it logs a message when the user reaches the end.
Advanced Usage: Implementing Lazy Loading
One of the most powerful features of react-infinite-grid
is its support for lazy loading. Let’s implement a grid that loads more items as the user scrolls:
import React, { useState, useCallback } from 'react';
import InfiniteGrid from 'react-infinite-grid';
const LazyLoadGrid: React.FC = () => {
const [items, setItems] = useState(() =>
Array.from({ length: 50 }, (_, i) => (
<div key={i} className="grid-item">Item {i}</div>
))
);
const loadMore = useCallback(() => {
const newItems = Array.from({ length: 20 }, (_, i) => (
<div key={items.length + i} className="grid-item">
Item {items.length + i}
</div>
));
setItems(prevItems => [...prevItems, ...newItems]);
}, [items.length]);
return (
<InfiniteGrid
entries={items}
lazyCallback={loadMore}
itemClassName="grid-item"
/>
);
};
In this example, we start with 50 items and add 20 more each time the user reaches the end of the grid. The lazyCallback
prop ensures that new items are loaded seamlessly.
Optimizing Performance with Memoization
When dealing with large datasets, memoization can significantly boost performance. Here’s how you can optimize your react-infinite-grid
implementation:
import React, { useMemo, useCallback } from 'react';
import InfiniteGrid from 'react-infinite-grid';
const MemoizedGrid: React.FC = () => {
const generateItems = useCallback((count: number) =>
Array.from({ length: count }, (_, i) => (
<div key={i} className="grid-item">Memoized Item {i}</div>
)),
[]
);
const items = useMemo(() => generateItems(10000), [generateItems]);
return (
<InfiniteGrid
entries={items}
itemClassName="grid-item"
/>
);
};
By using useMemo
and useCallback
, we ensure that our items array and item generation function are only recreated when necessary, reducing unnecessary re-renders and improving overall performance.
Wrapping Up: The Power of Infinite Scrolling
react-infinite-grid opens up a world of possibilities for handling large datasets in React applications. Its efficient rendering approach, combined with the flexibility to customize and implement lazy loading, makes it an invaluable tool for developers dealing with extensive lists or grids.
By leveraging this library, you can create smooth, performant user experiences even when working with thousands of items. Whether you’re building a complex data visualization tool or a simple image gallery, react-infinite-grid
provides the foundation for efficient, scalable grid layouts in React.
Remember, the key to mastering react-infinite-grid
lies in understanding its props and combining them with React’s built-in optimization techniques. With this knowledge, you’re well-equipped to tackle even the most demanding data presentation challenges in your React projects.
For more insights on optimizing your React applications, check out our articles on Infinite Grid Mastery with @egjs/react-infinitegrid and Mastering React Data Tables. Happy coding, and may your grids be ever infinite and performant!