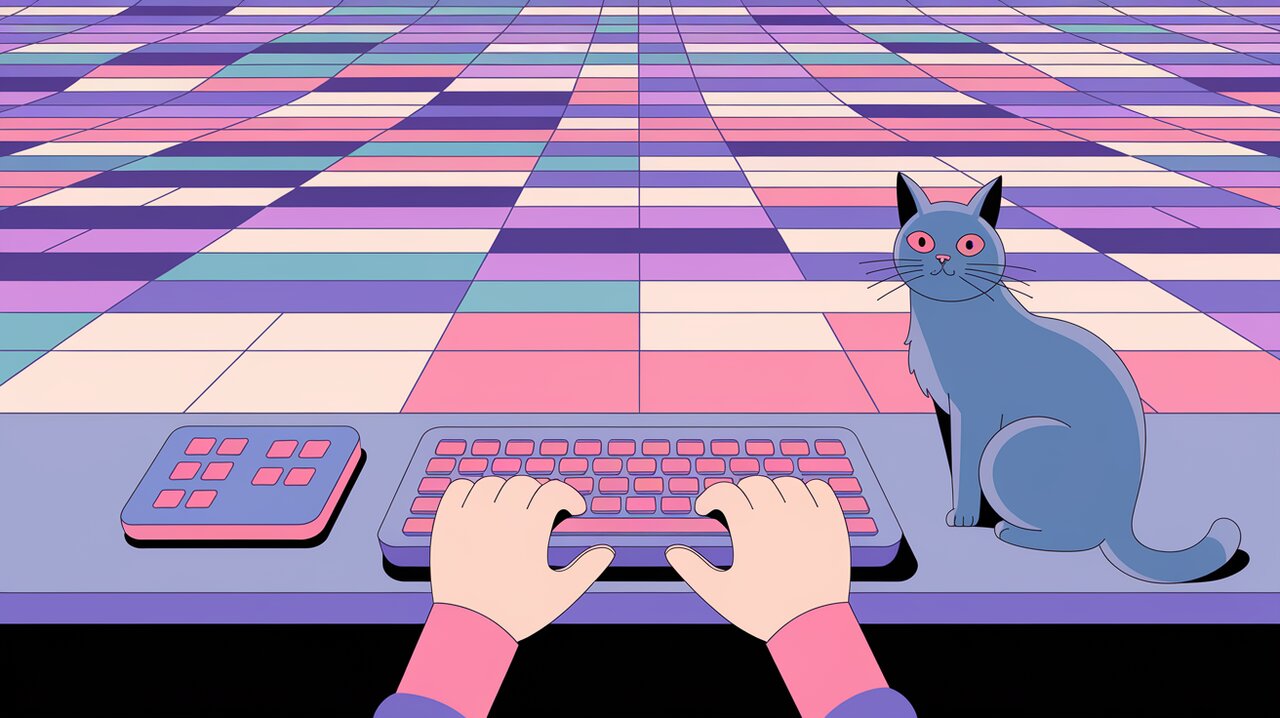
Mastering Infinite Grids with @egjs/react-infinitegrid
@egjs/react-infinitegrid is a powerful React library that enables developers to create dynamic, infinitely scrollable grid layouts with ease. This versatile tool is perfect for building responsive and performant user interfaces that can handle large amounts of data without compromising on speed or user experience.
Features of @egjs/react-infinitegrid
Infinite Scrolling: The library’s core feature allows for seamless loading of new content as the user scrolls, providing a smooth and engaging browsing experience[1].
Multiple Grid Types: @egjs/react-infinitegrid supports various grid layouts, including Masonry, Justified, Frame, Packing, and more, giving developers flexibility in design[1].
Performance Optimization: By maintaining a limited number of DOM elements and efficiently managing them, the library ensures high performance even with large datasets[1].
Responsive Design: Grids automatically adjust to different screen sizes, making it easy to create responsive layouts[1].
TypeScript Support: Fully written in TypeScript, the library provides excellent type safety and autocompletion in modern IDEs[3].
Framework Compatibility: While we’re focusing on React, it’s worth noting that the library also supports Angular, Vue, and Svelte[3].
Installation and Basic Setup
To get started with @egjs/react-infinitegrid, first install it via npm:
npm install @egjs/react-infinitegrid
Now, let’s create a basic infinite grid:
import React from 'react';
import { MasonryInfiniteGrid } from "@egjs/react-infinitegrid";
const MyGrid = () => {
const [items, setItems] = React.useState([]);
const getItems = (nextGroupKey: number, count: number) => {
const nextItems = [];
for (let i = 0; i < count; ++i) {
const nextKey = nextGroupKey * count + i;
nextItems.push({ key: nextKey, data: `Item ${nextKey}` });
}
return nextItems;
};
React.useEffect(() => {
setItems(getItems(0, 10));
}, []);
return (
<MasonryInfiniteGrid
className="container"
gap={5}
onRequestAppend={(e) => {
const nextGroupKey = (+e.groupKey || 0) + 1;
setItems([...items, ...getItems(nextGroupKey, 10)]);
}}
>
{items.map((item) => (
<div className="item" key={item.key}>
{item.data}
</div>
))}
</MasonryInfiniteGrid>
);
};
export default MyGrid;
This example creates a Masonry-style infinite grid that loads more items as the user scrolls[1].
Advanced Usage: Optimizing Performance
@egjs/react-infinitegrid offers several ways to optimize performance, especially when dealing with images or complex layouts.
Lazy Loading
Implement lazy loading for images to improve initial load time:
<MasonryInfiniteGrid
className="container"
gap={5}
>
{items.map((item) => (
<div className="item" key={item.key}>
<img src={item.imageUrl} loading="lazy" alt={item.description} />
</div>
))}
</MasonryInfiniteGrid>
The loading="lazy"
attribute tells the browser to load images only when they’re about to enter the viewport[1].
Pre-calculating Sizes
For even better performance, especially with varying content sizes, you can pre-calculate and specify item dimensions:
<MasonryInfiniteGrid
className="container"
gap={5}
>
{items.map((item) => (
<div
className="item"
key={item.key}
data-grid-width={item.width}
data-grid-height={item.height}
>
<img src={item.imageUrl} alt={item.description} />
</div>
))}
</MasonryInfiniteGrid>
By providing data-grid-width
and data-grid-height
attributes, you help the grid calculate layouts more efficiently[1].
Implementing Different Grid Types
@egjs/react-infinitegrid offers various grid types to suit different design needs. Let’s explore a couple:
Justified Grid
import { JustifiedInfiniteGrid } from "@egjs/react-infinitegrid";
// ... (similar setup as before)
return (
<JustifiedInfiniteGrid
className="container"
gap={5}
onRequestAppend={(e) => {
// ... (append logic)
}}
>
{items.map((item) => (
<div className="item" key={item.key}>
<img src={item.imageUrl} alt={item.description} />
</div>
))}
</JustifiedInfiniteGrid>
);
This creates a justified grid where items are aligned to have equal heights in each row[1].
Packing Grid
import { PackingInfiniteGrid } from "@egjs/react-infinitegrid";
// ... (similar setup as before)
return (
<PackingInfiniteGrid
className="container"
gap={5}
onRequestAppend={(e) => {
// ... (append logic)
}}
>
{items.map((item) => (
<div className="item" key={item.key}>
{item.data}
</div>
))}
</PackingInfiniteGrid>
);
The Packing grid type creates a compact layout by fitting items into available spaces[1].
Conclusion
@egjs/react-infinitegrid is a versatile and powerful library for creating dynamic, infinite-scroll grid layouts in React applications. Its support for various grid types, performance optimizations, and responsive design make it an excellent choice for developers looking to implement complex, data-heavy UIs.
By leveraging features like lazy loading, size pre-calculation, and different grid types, you can create highly performant and visually appealing layouts that enhance user experience and engagement.
As you continue to explore @egjs/react-infinitegrid, you might also find these related articles helpful:
These resources can provide additional insights into creating dynamic layouts and implementing infinite scrolling in React applications, complementing your knowledge of @egjs/react-infinitegrid.