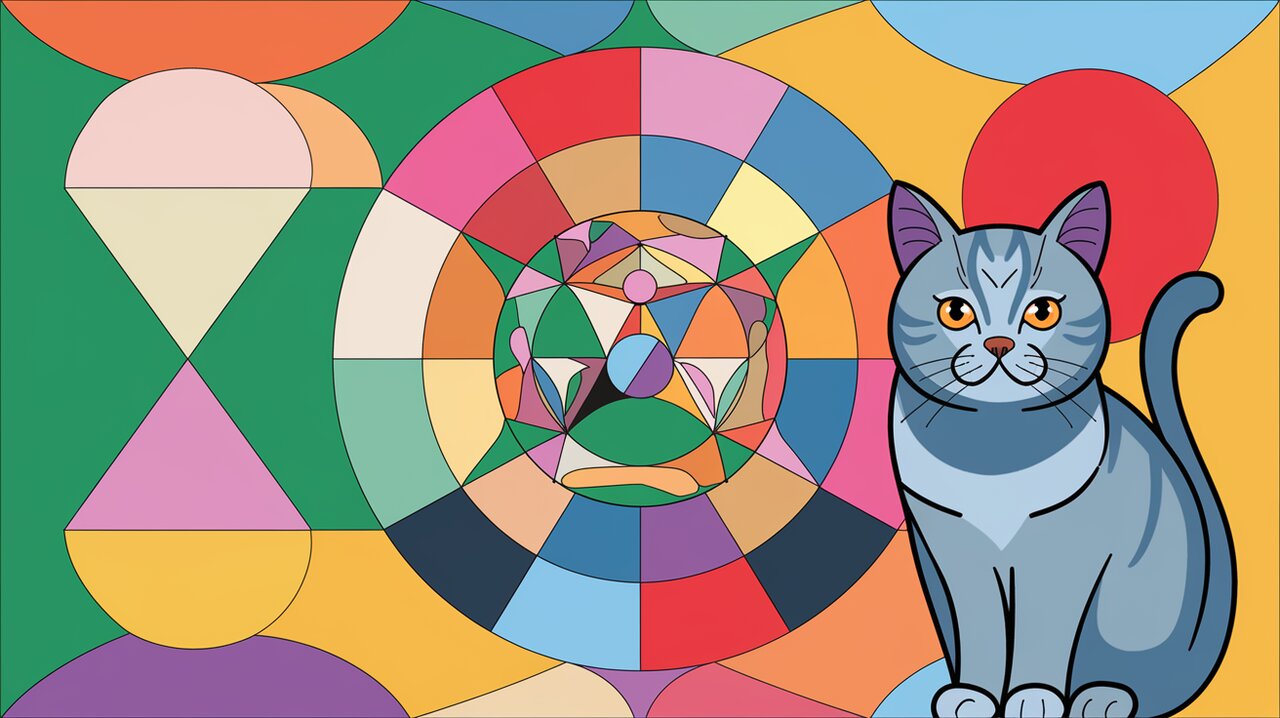
Kaleidoscopic Avatars: Unleashing React Avatar Generator's Magic
React developers are constantly seeking ways to enhance user interfaces and create memorable experiences. Enter react-avatar-generator, a library that brings the mesmerizing beauty of kaleidoscopes to user avatars. This powerful tool allows you to generate unique, visually striking avatars that can set your application apart from the crowd.
Unveiling the Kaleidoscope
The react-avatar-generator library draws inspiration from the LayerVault website, offering a fresh take on user avatars. Instead of traditional static images or initials, this library creates dynamic, kaleidoscope-like patterns that are both visually appealing and unique to each user.
Getting Started with react-avatar-generator
To begin your journey into the world of kaleidoscopic avatars, you’ll need to install the library. You can do this using either npm or yarn:
npm install react-avatar-generator
or
yarn add react-avatar-generator
Once installed, you can import the AvatarGenerator
component into your React application:
import AvatarGenerator from 'react-avatar-generator';
Basic Implementation
Let’s start with a simple implementation to see the AvatarGenerator
in action:
import React from 'react';
import AvatarGenerator from 'react-avatar-generator';
const UserProfile: React.FC = () => {
return (
<div>
<h1>User Profile</h1>
<AvatarGenerator
colors={['#333', '#222', '#ccc']}
backgroundColor="#000"
/>
</div>
);
};
export default UserProfile;
This basic setup creates a kaleidoscopic avatar using the specified colors against a black background. The result is a unique, eye-catching avatar that adds a touch of personality to your user profiles.
Customizing Your Kaleidoscope
The AvatarGenerator
component offers a wide range of props to customize the appearance of your avatars. Let’s explore some of these options:
Adjusting Dimensions
You can control the size of your avatar by setting the width
and height
props:
<AvatarGenerator
width={200}
height={200}
colors={['#FF5733', '#33FF57', '#3357FF']}
backgroundColor="#F0F0F0"
/>
Shaping Your Avatar
The shape
prop allows you to choose between different avatar shapes:
<AvatarGenerator
shape="circle"
width={150}
height={150}
colors={['#FFD700', '#4B0082', '#00CED1']}
/>
You can set shape
to 'circle'
, 'triangle'
, or 'square'
depending on your design preferences.
Fine-tuning the Kaleidoscope Effect
Several props allow you to adjust the intricacy of the kaleidoscope pattern:
<AvatarGenerator
mirrors={5}
zoom={0.3}
rotation={0.5}
fade={0.8}
opacity={0.4}
amount={20}
spacing={25}
wavelength={3}
sizing={5}
colors={['#E6E6FA', '#FFA07A', '#98FB98']}
/>
These props control various aspects of the kaleidoscope effect, from the number of mirrors to the spacing and opacity of the patterns.
Advanced Usage: Randomization and Image Data
The AvatarGenerator
component also provides methods for more advanced functionality:
Randomizing Avatars
You can create a button to generate new random avatars:
import React, { useRef } from 'react';
import AvatarGenerator from 'react-avatar-generator';
const RandomAvatar: React.FC = () => {
const avatarRef = useRef<AvatarGenerator>(null);
const handleRandomize = () => {
if (avatarRef.current) {
avatarRef.current.randomize();
}
};
return (
<div>
<AvatarGenerator
ref={avatarRef}
colors={['#FF6B6B', '#4ECDC4', '#45B7D1']}
/>
<button onClick={handleRandomize}>Generate New Avatar</button>
</div>
);
};
export default RandomAvatar;
This setup allows users to generate new avatar designs with a single click.
Saving Avatar Images
To enable users to save their generated avatars, you can use the getImageData
method:
import React, { useRef } from 'react';
import AvatarGenerator from 'react-avatar-generator';
const SaveableAvatar: React.FC = () => {
const avatarRef = useRef<AvatarGenerator>(null);
const handleSave = () => {
if (avatarRef.current) {
const imageData = avatarRef.current.getImageData();
const link = document.createElement('a');
link.href = imageData;
link.download = 'my-avatar.png';
link.click();
}
};
return (
<div>
<AvatarGenerator
ref={avatarRef}
colors={['#6B5B95', '#FFA07A', '#88B04B']}
/>
<button onClick={handleSave}>Save Avatar</button>
</div>
);
};
export default SaveableAvatar;
This code allows users to download their generated avatar as a PNG file.
Integrating with Other React Libraries
While react-avatar-generator offers a unique approach to avatars, you might want to use it in conjunction with other avatar libraries for a more comprehensive solution. For instance, you could combine it with a library like react-avatar
for fallback options.
To learn more about implementing versatile avatar solutions, check out our article on React Avatar: From Installation to Advanced Customization.
Conclusion
The react-avatar-generator library opens up a world of creative possibilities for user avatars in React applications. By leveraging its kaleidoscopic patterns and extensive customization options, you can create visually stunning and unique avatars that enhance user engagement and personalization in your projects.
Whether you’re building a social platform, a user management system, or any application that requires user representation, react-avatar-generator provides an innovative solution that stands out from traditional avatar implementations.
For more insights on working with avatars in React, don’t miss our guide on How to Create an Avatar Feature with React, which explores additional techniques and libraries to further enhance your avatar implementation.
Embrace the kaleidoscopic revolution and give your users an avatar experience they won’t forget!