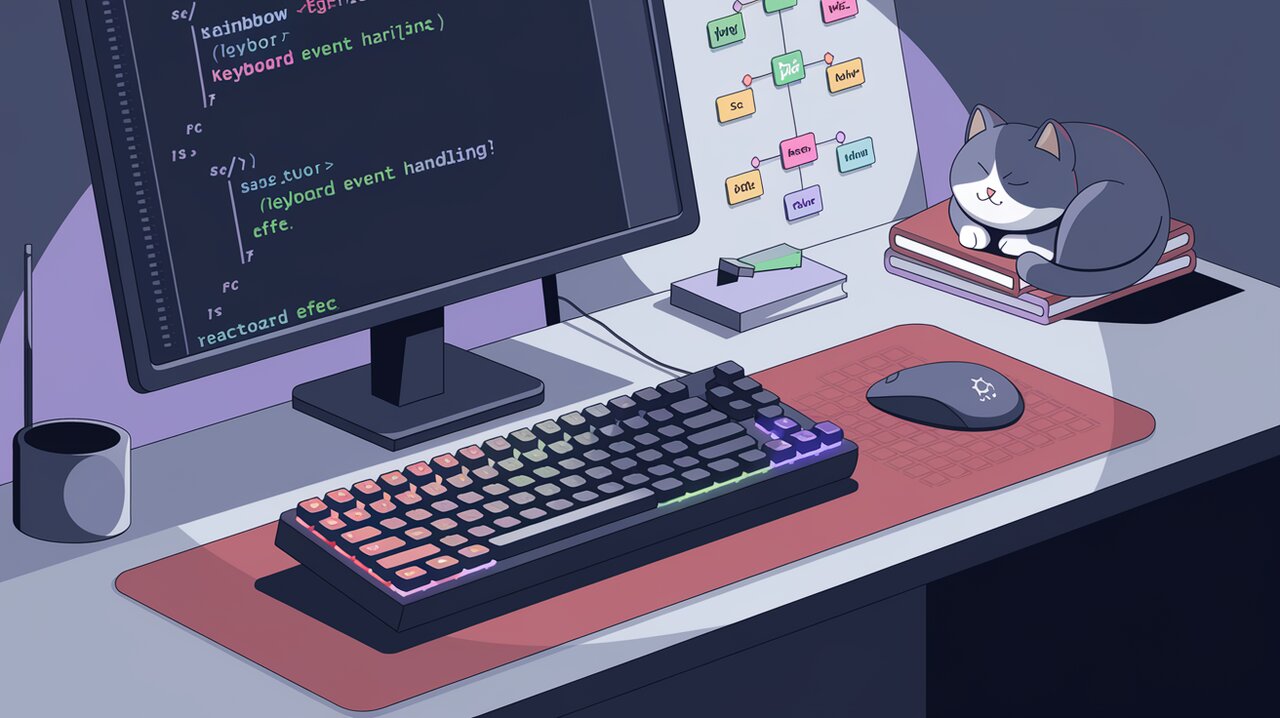
Keyboard Wizardry: Unleashing the Power of react-keyboard-event-handler
Introduction
In the fast-paced world of web applications, keyboard shortcuts are the secret weapon of power users. They provide a quick and efficient way to navigate and interact with an application, significantly enhancing productivity. The react-keyboard-event-handler
library brings this power to React developers, offering a robust and flexible solution for implementing keyboard event handling in React applications.
Features
react-keyboard-event-handler
comes packed with an impressive array of features that set it apart:
- Support for combined key presses (e.g., CTRL + S or CTRL + SHIFT + S)
- Ability to handle modifier keys independently (e.g., detecting a standalone ‘ctrl’ key press)
- Compatibility with a wide range of keys, including function keys
- Consistent and intuitive key naming conventions
- Key aliases like ‘alphanumeric’ and ‘all’ for efficient multiple key handling
- Support for multiple handler instances with granular control over enable/disable states
Installation
Getting started with react-keyboard-event-handler
is straightforward. You can install it using npm or yarn:
npm install react-keyboard-event-handler
or
yarn add react-keyboard-event-handler
Basic Usage
Let’s explore how to integrate react-keyboard-event-handler
into your React components.
Handling Global Key Events
By default, KeyboardEventHandler
captures global key events from document.body
. This means it can respond to key presses anywhere on the page, regardless of focus.
import React from 'react';
import KeyboardEventHandler from 'react-keyboard-event-handler';
const GlobalKeyHandler = () => {
return (
<div>
<KeyboardEventHandler
handleKeys={['a', 'b', 'c']}
onKeyEvent={(key, e) => {
console.log(`You pressed: ${key}`);
}}
/>
<p>Press A, B, or C to see the magic happen!</p>
</div>
);
};
In this example, the component will log a message to the console whenever the user presses A, B, or C, regardless of where the focus is on the page.
Handling Specific Element Events
You can also use KeyboardEventHandler
to capture events from specific child elements:
import React from 'react';
import KeyboardEventHandler from 'react-keyboard-event-handler';
const InputKeyHandler = () => {
return (
<KeyboardEventHandler
handleKeys={['enter']}
onKeyEvent={(key, e) => {
console.log('Enter key pressed in the input field');
}}
>
<input type="text" placeholder="Press Enter here" />
</KeyboardEventHandler>
);
};
This setup will only trigger the handler when the Enter key is pressed while the input field is focused.
Advanced Usage
Handling Combined Keys
One of the standout features of react-keyboard-event-handler
is its ability to handle combined key presses:
import React from 'react';
import KeyboardEventHandler from 'react-keyboard-event-handler';
const SaveShortcutHandler = () => {
return (
<KeyboardEventHandler
handleKeys={['ctrl+s', 'cmd+s']}
onKeyEvent={(key, e) => {
e.preventDefault();
console.log('Save action triggered');
// Implement your save logic here
}}
>
<div>Press Ctrl+S (or Cmd+S on Mac) to save</div>
</KeyboardEventHandler>
);
};
This example demonstrates how to implement a save shortcut that works across different operating systems.
Using Key Aliases
The library provides convenient aliases for groups of keys:
import React from 'react';
import KeyboardEventHandler from 'react-keyboard-event-handler';
const AlphanumericHandler = () => {
return (
<KeyboardEventHandler
handleKeys={['alphanumeric']}
onKeyEvent={(key, e) => {
console.log(`Alphanumeric key pressed: ${key}`);
}}
>
<div>Type any letter or number</div>
</KeyboardEventHandler>
);
};
This handler will respond to any alphanumeric key press, making it easier to handle a range of inputs without listing each key individually.
Dynamic Handler Control
You can dynamically enable or disable the keyboard event handler:
import React, { useState } from 'react';
import KeyboardEventHandler from 'react-keyboard-event-handler';
const ToggleableHandler = () => {
const [isEnabled, setIsEnabled] = useState(true);
return (
<div>
<KeyboardEventHandler
handleKeys={['all']}
isDisabled={!isEnabled}
onKeyEvent={(key) => {
console.log(`Key pressed: ${key}`);
}}
/>
<button onClick={() => setIsEnabled(!isEnabled)}>
{isEnabled ? 'Disable' : 'Enable'} Handler
</button>
</div>
);
};
This example shows how to toggle the handler on and off, which can be useful for creating modal dialogs or temporary overlays.
Conclusion
The react-keyboard-event-handler
library opens up a world of possibilities for creating rich, interactive user interfaces in React applications. By leveraging its powerful features, developers can implement sophisticated keyboard shortcuts and events that cater to power users and improve overall application usability.
Remember that with great power comes great responsibility. When implementing keyboard shortcuts, always consider accessibility and ensure that all users, regardless of their input methods, can navigate and interact with your application effectively. With react-keyboard-event-handler
in your toolkit, you’re well-equipped to create intuitive and efficient keyboard interactions that will delight your users and set your React applications apart.