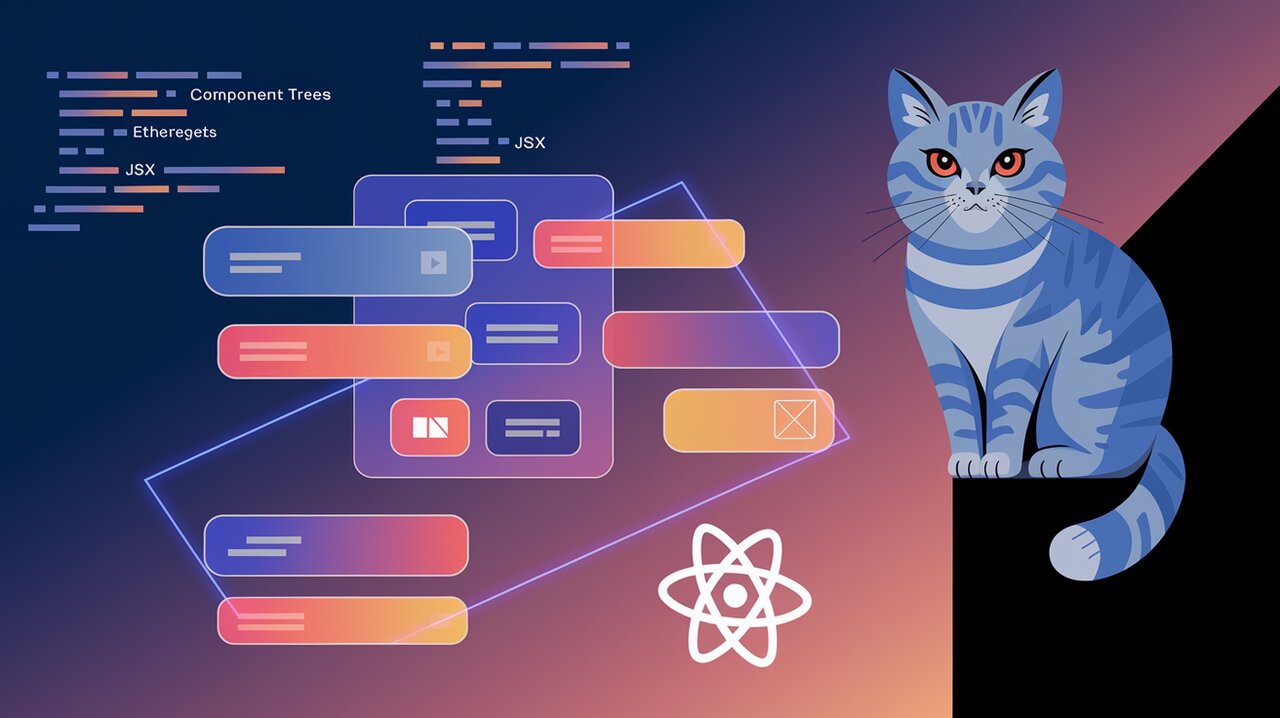
Lasso Your React Elements with react-selectable-fast
React developers often face the challenge of implementing complex user interactions in their applications. One such interaction is the ability to select multiple items by drawing a selection box with the mouse or touch input. This functionality is crucial for applications dealing with lists, grids, or any collection of selectable items. The react-selectable-fast library offers a solution to this challenge, providing a high-performance way to add drag-to-select capabilities to your React components.
What is react-selectable-fast?
react-selectable-fast is a React library that enables components to be selectable via mouse or touch input. It’s an enhanced version of the original react-selectable
library, optimized for performance with large lists of selectable items. The key improvement is that it eliminates unnecessary re-renders during the selection process, ensuring smooth performance even with a large number of items.
Features
- Efficient selection of multiple items using a drag box
- Support for both mouse and touch events
- Customizable selection box appearance
- Ability to scroll while selecting
- Support for selecting and deselecting items
- Callbacks for various stages of the selection process
- Compatible with fixed/absolute positioned containers
Installation
To get started with react-selectable-fast, you can install it via npm:
npm install react-selectable-fast
Or if you prefer using yarn:
yarn add react-selectable-fast
Basic Usage
Let’s dive into how you can use react-selectable-fast in your React application. The library exports several key components and functions that you’ll use to implement the selection functionality.
Setting up the Selectable Group
First, you’ll need to wrap your selectable items with the SelectableGroup
component:
import React from 'react';
import { SelectableGroup } from 'react-selectable-fast';
const App = () => {
const handleSelection = (selectedItems) => {
console.log('Selected items:', selectedItems);
};
return (
<SelectableGroup
className="selectable-group"
onSelectionFinish={handleSelection}
>
{/* Your selectable items go here */}
</SelectableGroup>
);
};
The SelectableGroup
component sets up the area where selection can occur and handles the overall selection logic.
Creating Selectable Items
Next, you’ll need to make your items selectable. You can do this using the createSelectable
higher-order component:
import React from 'react';
import { createSelectable } from 'react-selectable-fast';
const MySelectableComponent = ({ selectableRef, selected, selecting }) => (
<div
ref={selectableRef}
className={`selectable-item ${selected ? 'selected' : ''} ${selecting ? 'selecting' : ''}`}
>
{/* Your item content */}
</div>
);
export default createSelectable(MySelectableComponent);
The createSelectable
HOC adds the necessary props and functionality to make your component selectable.
Putting It All Together
Now you can use your selectable components within the SelectableGroup
:
import React from 'react';
import { SelectableGroup } from 'react-selectable-fast';
import MySelectableComponent from './MySelectableComponent';
const App = () => {
const items = [/* Your list of items */];
return (
<SelectableGroup>
{items.map((item) => (
<MySelectableComponent key={item.id} {...item} />
))}
</SelectableGroup>
);
};
Advanced Usage
react-selectable-fast offers several advanced features to enhance your selection functionality.
Customizing Selection Behavior
You can customize the selection behavior using various props on the SelectableGroup
component:
<SelectableGroup
tolerance={10}
enableDeselect
mixedDeselect
scrollContainer=".scroll-container"
allowClickWithoutSelected={false}
duringSelection={(selectedItems) => console.log('Currently selecting:', selectedItems)}
onSelectionClear={() => console.log('Selection cleared')}
>
{/* Selectable items */}
</SelectableGroup>
These props allow you to fine-tune the selection experience, such as allowing deselection, handling scrolling containers, and responding to various selection events.
Select All and Deselect All Functionality
react-selectable-fast also provides components for selecting or deselecting all items at once:
import React from 'react';
import { SelectableGroup, SelectAll, DeselectAll } from 'react-selectable-fast';
const App = () => (
<SelectableGroup>
<SelectAll>
<button>Select All</button>
</SelectAll>
<DeselectAll>
<button>Deselect All</button>
</DeselectAll>
{/* Selectable items */}
</SelectableGroup>
);
These components make it easy to add bulk selection and deselection functionality to your application.
Performance Considerations
One of the key advantages of react-selectable-fast is its performance optimization. Unlike its predecessor, it minimizes re-renders during the selection process, making it suitable for applications with a large number of selectable items.
To further optimize performance, consider the following tips:
- Use the
tolerance
prop to adjust the precision of selection, potentially reducing the number of calculations. - If your selectable items are in a scrollable container, use the
scrollContainer
prop to ensure accurate selection calculations. - Implement virtualization for very large lists to render only visible items.
Conclusion
react-selectable-fast provides a powerful and efficient solution for implementing drag-to-select functionality in React applications. Its optimized performance and rich feature set make it an excellent choice for developers looking to enhance their user interfaces with intuitive selection capabilities.
By leveraging this library, you can create more interactive and user-friendly interfaces, especially for applications dealing with large datasets or complex item selections. Whether you’re building a file manager, a photo gallery, or any application that requires multi-select functionality, react-selectable-fast offers the tools you need to create a smooth and responsive selection experience.
As you implement selection features in your React applications, you might also be interested in exploring other UI enhancement libraries. For instance, you could look into react-beautiful-dnd for drag-and-drop functionality or react-grid-layout for creating dynamic, resizable layouts. These libraries can complement the selection capabilities of react-selectable-fast, helping you build even more powerful and flexible user interfaces.