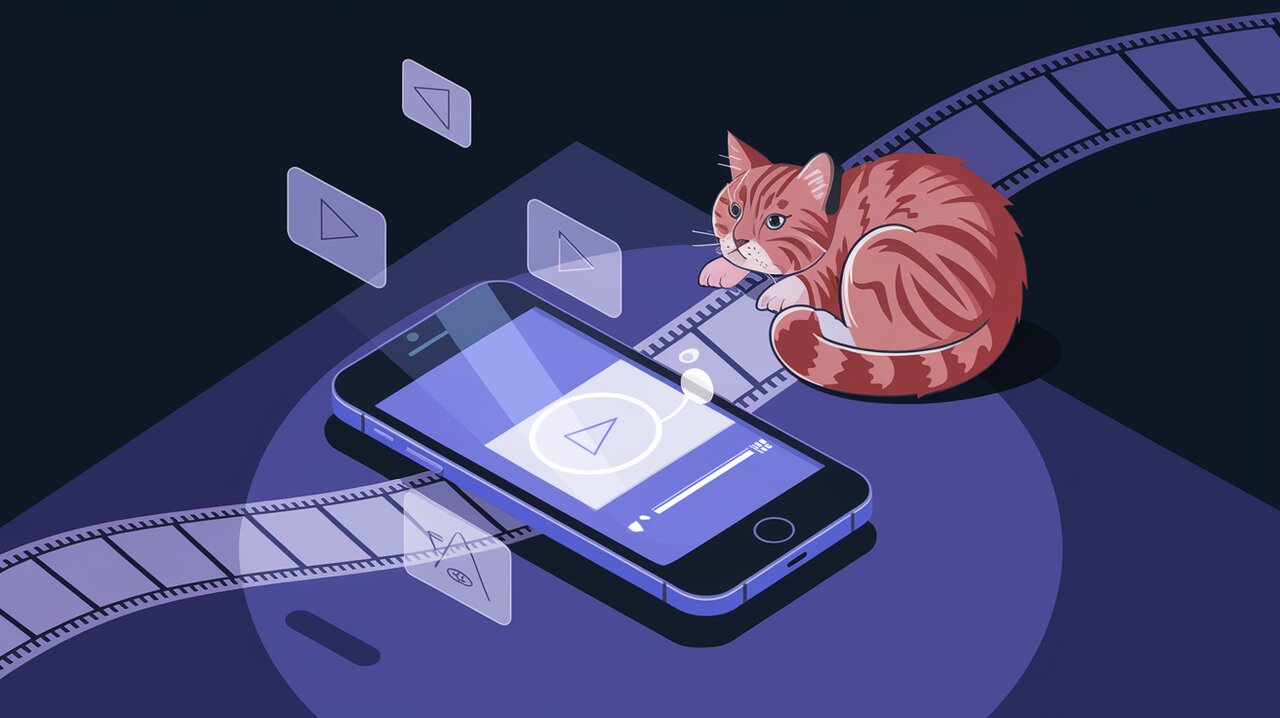
Lights, Camera, Action: Mastering React Native Video Player
React Native has revolutionized the way we build cross-platform mobile applications, and when it comes to incorporating video content, the react-native-video-player library stands out as a powerful ally. This versatile component wraps around the popular react-native-video
package, providing developers with an out-of-the-box solution for embedding video players in their apps with minimal setup and maximum flexibility.
Unleashing Video Playback Power
The react-native-video-player library boasts a range of features that make it a go-to choice for developers:
- Customizable video controls
- Support for both local and remote video sources
- Thumbnail display capabilities
- Error handling for seamless user experience
- Duration display options
- Ref-based imperative API for advanced control
These features combine to create a robust video playback solution that can be tailored to fit the unique needs of any React Native application.
Setting the Stage: Installation
Before we dive into the code, let’s set up our project with the necessary dependencies. You can install react-native-video-player using either yarn or npm:
# Using yarn
yarn add react-native-video-player react-native-video
# Using npm
npm install --save react-native-video-player react-native-video
After installation, iOS developers need to install the pods:
cd ios
pod install
With these steps completed, we’re ready to start implementing video playback in our React Native app.
Lights, Camera, Action: Basic Usage
Let’s jump right into a basic implementation of the video player. Here’s a simple example that demonstrates how to set up a video player with a thumbnail and error handling:
import React, { useRef } from 'react';
import VideoPlayer, { VideoPlayerRef } from 'react-native-video-player';
const VideoComponent: React.FC = () => {
const playerRef = useRef<VideoPlayerRef>(null);
return (
<VideoPlayer
ref={playerRef}
endWithThumbnail
thumbnail={{ uri: 'https://example.com/thumbnail.jpg' }}
source={{ uri: 'https://example.com/video.mp4' }}
onError={(e) => console.log('Video playback error:', e)}
showDuration={true}
/>
);
};
export default VideoComponent;
In this example, we’re creating a video player with a thumbnail that displays at the end of the video. We’re also setting up error handling and enabling the duration display. The ref
allows us to access the player’s methods imperatively if needed.
Advanced Techniques: Customizing the Experience
The react-native-video-player library offers various props to customize the video playback experience. Let’s explore some advanced usage scenarios:
Controlling Playback
You can control the video playback programmatically using the ref:
import React, { useRef } from 'react';
import { Button } from 'react-native';
import VideoPlayer, { VideoPlayerRef } from 'react-native-video-player';
const ControlledVideoPlayer: React.FC = () => {
const playerRef = useRef<VideoPlayerRef>(null);
const handlePlay = () => {
playerRef.current?.resume();
};
const handlePause = () => {
playerRef.current?.pause();
};
return (
<>
<VideoPlayer
ref={playerRef}
source={{ uri: 'https://example.com/video.mp4' }}
/>
<Button title="Play" onPress={handlePlay} />
<Button title="Pause" onPress={handlePause} />
</>
);
};
This setup allows you to control the video playback externally, which can be useful for creating custom control interfaces.
Customizing the Player’s Appearance
You can customize the appearance of the video player by passing style props:
<VideoPlayer
source={{ uri: 'https://example.com/video.mp4' }}
style={{ height: 300, width: '100%' }}
controlsTimeout={5000}
disableControlsAutoHide={false}
resizeMode="contain"
/>
This configuration sets a specific size for the player, adjusts the timeout for control visibility, and ensures the video fits within the player’s bounds.
Handling Video Events
The library provides various callback props to handle different video events:
<VideoPlayer
source={{ uri: 'https://example.com/video.mp4' }}
onEnd={() => console.log('Video playback completed')}
onLoad={(data) => console.log('Video loaded', data)}
onProgress={(data) => console.log('Playback progress', data)}
/>
These callbacks allow you to respond to different stages of video playback, enabling you to create more interactive and responsive video experiences.
Conclusion: A Star in Your React Native Toolkit
The react-native-video-player library simplifies the process of integrating video playback into React Native applications. Its combination of ease of use and flexibility makes it an excellent choice for developers looking to enhance their apps with rich media content.
As you continue to explore the capabilities of this library, you’ll find that it can handle a wide range of video playback scenarios, from simple inline videos to more complex, interactive video experiences. Whether you’re building a social media app, an educational platform, or a content-rich mobile application, react-native-video-player provides the tools you need to create engaging video interfaces.
Remember to check out the library’s documentation for the most up-to-date information on props, methods, and best practices. Happy coding, and may your React Native apps shine bright with seamless video playback!
For more insights into React Native development, you might want to explore our articles on React Native Web: Unifying Mobile and Web Development and Reanimated: React Native Motion Magic. These resources can help you further enhance your mobile app development skills and create truly cross-platform experiences.