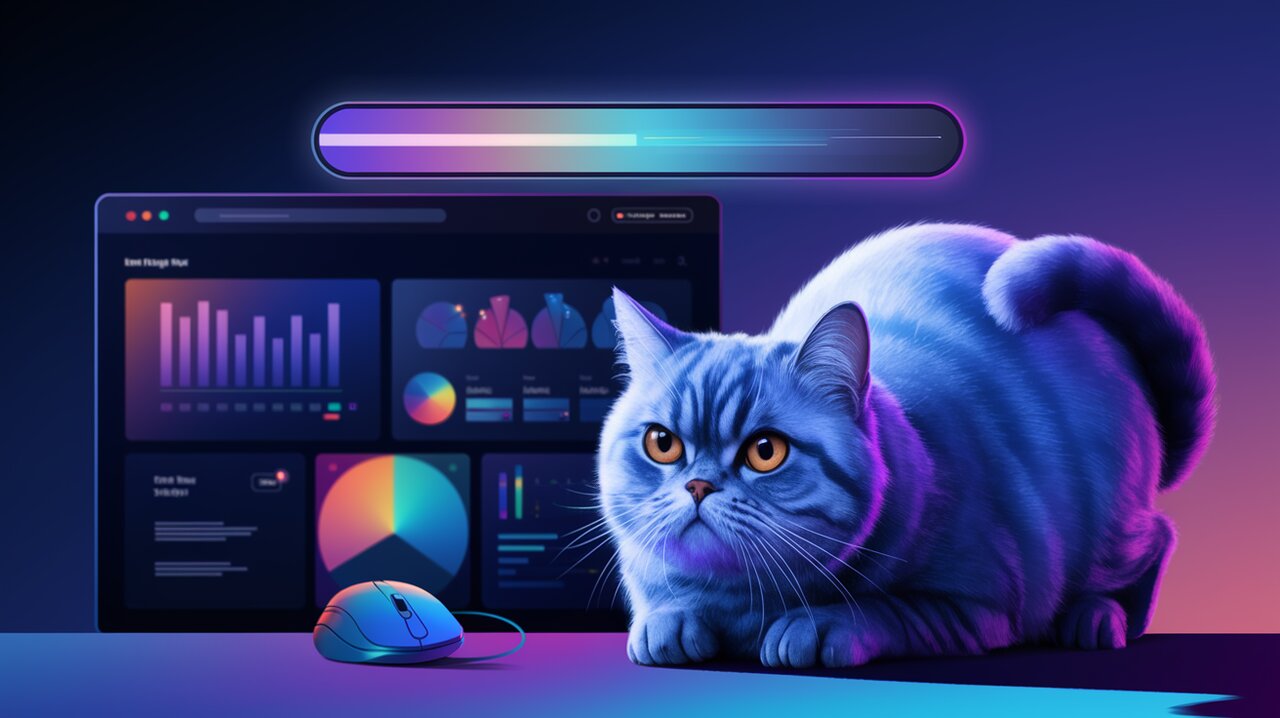
Loading Bar Symphony: Orchestrating Progress with React Redux Loading Bar
React Redux Loading Bar is a powerful library that brings harmony to the often chaotic world of asynchronous operations in web applications. By providing a simple yet effective way to display loading progress, this library enhances user experience and gives developers fine-grained control over loading indicators. Let’s dive into the melodious world of React Redux Loading Bar and see how it can orchestrate loading states in your React applications.
Setting the Stage: Installation and Setup
Before we can start our loading bar symphony, we need to set up our instruments. Install the library using npm or yarn:
npm install --save react-redux-loading-bar
# or
yarn add react-redux-loading-bar
With the library installed, it’s time to integrate it into your Redux setup. First, add the loading bar reducer to your root reducer:
import { combineReducers } from 'redux';
import { loadingBarReducer } from 'react-redux-loading-bar';
const rootReducer = combineReducers({
// Your other reducers
loadingBar: loadingBarReducer,
});
Next, mount the LoadingBar component in your application, typically in your main layout or header component:
import React from 'react';
import LoadingBar from 'react-redux-loading-bar';
const Header: React.FC = () => {
return (
<header>
<LoadingBar />
{/* Other header content */}
</header>
);
};
export default Header;
The First Movement: Basic Usage
With our setup complete, let’s start with a simple demonstration of how to show and hide the loading bar. The library provides action creators that you can dispatch to control the loading bar’s visibility:
import { showLoading, hideLoading } from 'react-redux-loading-bar';
// In your component or thunk
dispatch(showLoading());
// Perform some asynchronous operation
// ...
dispatch(hideLoading());
This basic usage allows you to manually control the loading bar’s visibility. It’s particularly useful for operations where you need fine-grained control over when the loading indicator appears and disappears.
The Second Movement: Middleware Magic
For a more automated approach, especially when dealing with promise-based actions, you can use the provided middleware. This middleware will automatically show the loading bar when a promise-based action is dispatched and hide it when the promise resolves or rejects:
import { createStore, applyMiddleware } from 'redux';
import { loadingBarMiddleware } from 'react-redux-loading-bar';
import rootReducer from './reducers';
const store = createStore(
rootReducer,
applyMiddleware(loadingBarMiddleware())
);
With this middleware in place, any action that returns a promise will automatically trigger the loading bar. This is particularly useful when working with libraries like redux-thunk or redux-promise-middleware.
The Third Movement: Customization and Styling
React Redux Loading Bar offers various props to customize its behavior and appearance. Here are some key customization options:
<LoadingBar
style={{ backgroundColor: 'blue', height: '5px' }}
updateTime={100}
maxProgress={95}
progressIncrease={5}
showFastActions
/>
In this example:
- We’ve changed the color and height of the loading bar.
updateTime
sets how often the loading bar updates (in milliseconds).maxProgress
defines the maximum width the bar can reach before completion.progressIncrease
determines how much the progress increases with each update.showFastActions
allows the bar to show even for quick actions.
The Fourth Movement: Multiple Loading Bars
For complex applications, you might need multiple loading bars for different sections. React Redux Loading Bar supports this through scopes:
// In your reducer
import { combineReducers } from 'redux';
import { loadingBarReducer } from 'react-redux-loading-bar';
const rootReducer = combineReducers({
// ...other reducers
loadingBar: loadingBarReducer,
sectionLoadingBar: loadingBarReducer,
});
// In your component
<LoadingBar scope="sectionLoadingBar" />
// In your actions
import { showLoading, hideLoading } from 'react-redux-loading-bar';
dispatch(showLoading('sectionLoadingBar'));
// ...async operation
dispatch(hideLoading('sectionLoadingBar'));
This approach allows you to have independent loading bars for different parts of your application, providing more granular feedback to users.
The Grand Finale: Integration with Async Workflows
To bring it all together, let’s look at how React Redux Loading Bar can be integrated into a typical async workflow using redux-thunk:
import { showLoading, hideLoading } from 'react-redux-loading-bar';
const fetchData = () => async (dispatch) => {
dispatch(showLoading());
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
dispatch({ type: 'FETCH_DATA_SUCCESS', payload: data });
} catch (error) {
dispatch({ type: 'FETCH_DATA_FAILURE', error });
} finally {
dispatch(hideLoading());
}
};
In this example, the loading bar will appear as soon as the fetch operation starts and disappear once it’s completed, regardless of success or failure.
Encore: Best Practices and Considerations
- Consistent Usage: Use the loading bar consistently across your application for a unified user experience.
- Error Handling: Always ensure that
hideLoading()
is called, even in error scenarios, to prevent the loading bar from getting stuck. - Performance: For very frequent updates, consider adjusting the
updateTime
to balance smoothness and performance. - Accessibility: While visual indicators are helpful, also consider non-visual ways to communicate loading states for accessibility.
React Redux Loading Bar orchestrates a beautiful symphony of user feedback in your React applications. By providing visual cues for asynchronous operations, it enhances user experience and gives developers powerful tools to manage loading states.
As you implement loading indicators in your React applications, you might also be interested in exploring other UI enhancement libraries. Check out our articles on React Toastify for dynamic notifications and React Modal for creating modals to further elevate your user interface.
With React Redux Loading Bar, you’re well-equipped to create responsive, user-friendly applications that keep your audience informed and engaged throughout their journey in your web application. Happy coding, and may your loading bars always progress smoothly!