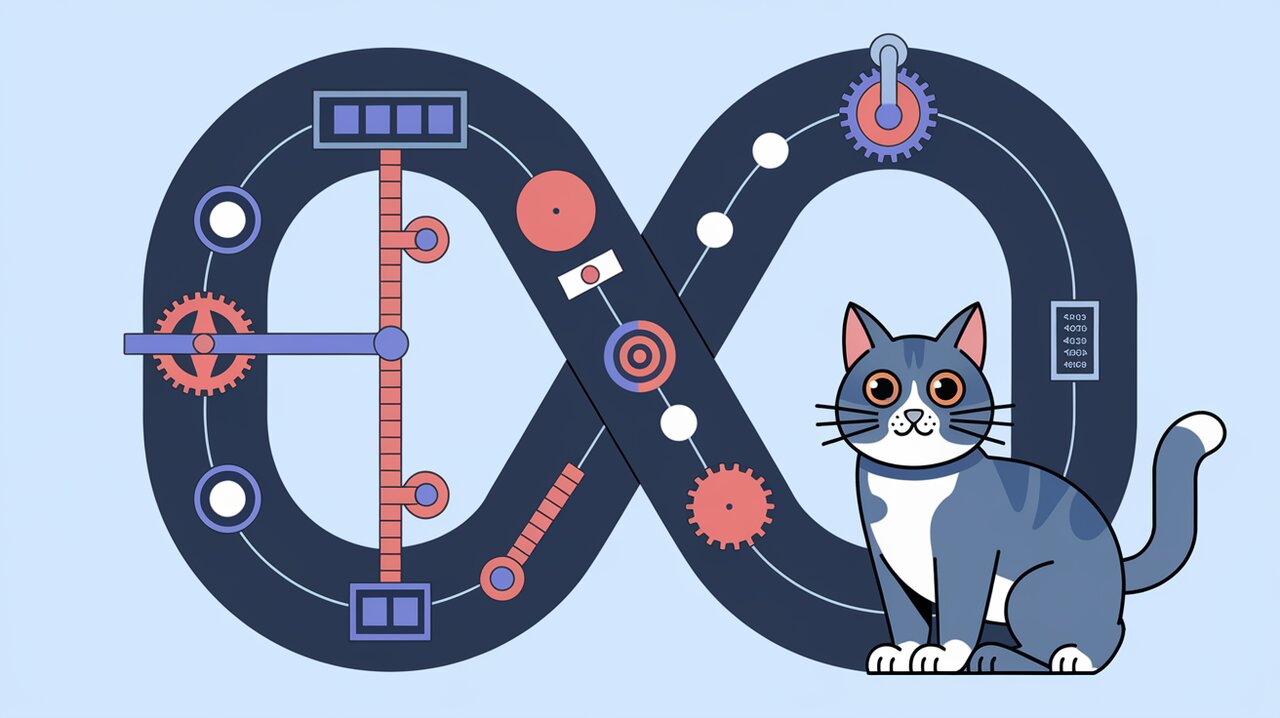
Looping Through Redux Effects: Unleashing the Power of redux-loop
Redux is a powerful state management library for JavaScript applications, but handling side effects can sometimes be challenging. Enter redux-loop, a library that brings the elegance of the Elm Architecture to Redux, allowing developers to sequence effects naturally and purely within their reducers. Let’s dive into how redux-loop can revolutionize your Redux code and make handling side effects a breeze.
Unraveling the Redux Loop
At its core, redux-loop extends Redux’s capabilities by allowing reducers to return both a new state and a description of side effects. This approach keeps your reducers pure while providing a clear and centralized way to manage effects.
Key Features of redux-loop
- Pure Reducers: Maintain the purity of your reducers while still describing side effects.
- Testability: Easily test your reducers without worrying about side effects being executed.
- Elm-inspired: Brings the power of the Elm Architecture to Redux applications.
- Composable Effects: Combine and sequence multiple effects with ease.
- TypeScript Support: Fully typed for improved developer experience.
Getting Started with redux-loop
Let’s begin by installing redux-loop in your project:
npm install redux-loop
Or if you prefer yarn:
yarn add redux-loop
Basic Usage: Looping Through Effects
To start using redux-loop, you need to enhance your Redux store and modify your reducers to return effects when necessary.
Enhancing Your Store
First, let’s set up the Redux store with redux-loop:
import { createStore, applyMiddleware } from 'redux';
import { install, loop, Cmd } from 'redux-loop';
import rootReducer from './reducers';
const store = createStore(rootReducer, undefined, install());
The install()
function enhances your store to work with redux-loop.
Creating Loop-Enabled Reducers
Now, let’s create a reducer that uses redux-loop to handle side effects:
import { loop, Cmd } from 'redux-loop';
import { fetchUserData } from './api';
interface UserState {
loading: boolean;
data: any | null;
error: string | null;
}
const initialState: UserState = {
loading: false,
data: null,
error: null,
};
function userReducer(state = initialState, action: any) {
switch (action.type) {
case 'FETCH_USER_REQUEST':
return loop(
{ ...state, loading: true },
Cmd.run(fetchUserData, {
successActionCreator: (data) => ({ type: 'FETCH_USER_SUCCESS', payload: data }),
failActionCreator: (error) => ({ type: 'FETCH_USER_FAILURE', payload: error.message }),
})
);
case 'FETCH_USER_SUCCESS':
return { ...state, loading: false, data: action.payload, error: null };
case 'FETCH_USER_FAILURE':
return { ...state, loading: false, data: null, error: action.payload };
default:
return state;
}
}
In this example, when the FETCH_USER_REQUEST
action is dispatched, the reducer returns both a new state (setting loading
to true) and a command to run the fetchUserData
function. The Cmd.run
method describes the effect without actually executing it, maintaining the purity of the reducer.
Advanced Usage: Composing Effects
One of the powerful features of redux-loop is the ability to compose multiple effects. Let’s look at a more complex example:
import { loop, Cmd } from 'redux-loop';
import { fetchUserData, fetchUserPosts, logAction } from './api';
function userReducer(state = initialState, action: any) {
switch (action.type) {
case 'FETCH_USER_AND_POSTS':
return loop(
{ ...state, loading: true },
Cmd.list([
Cmd.run(fetchUserData, {
successActionCreator: (data) => ({ type: 'FETCH_USER_SUCCESS', payload: data }),
failActionCreator: (error) => ({ type: 'FETCH_USER_FAILURE', payload: error.message }),
}),
Cmd.run(fetchUserPosts, {
successActionCreator: (posts) => ({ type: 'FETCH_POSTS_SUCCESS', payload: posts }),
failActionCreator: (error) => ({ type: 'FETCH_POSTS_FAILURE', payload: error.message }),
}),
Cmd.run(logAction, { args: ['FETCH_USER_AND_POSTS'] }),
])
);
// ... handle other actions
}
}
Here, we’re using Cmd.list
to compose multiple effects. When the FETCH_USER_AND_POSTS
action is dispatched, it will trigger fetching user data, user posts, and logging the action, all in parallel.
Testing Redux-Loop Reducers
One of the significant advantages of redux-loop is the ease of testing. Since reducers remain pure functions, you can test them without worrying about side effects being executed:
import { getModel, getCmd } from 'redux-loop';
test('FETCH_USER_REQUEST action', () => {
const action = { type: 'FETCH_USER_REQUEST' };
const result = userReducer(initialState, action);
const [model, cmd] = result;
expect(model).toEqual({ ...initialState, loading: true });
expect(getCmd(result)).toBeTruthy(); // Check if a command was returned
});
Conclusion: Embracing the Loop
Redux-loop offers a powerful and elegant solution for handling side effects in Redux applications. By allowing you to describe effects directly in your reducers, it promotes a more centralized and easier-to-reason-about codebase. Whether you’re building a small React application or a large-scale Redux project, redux-loop can help you manage complexity and keep your code clean and testable.
As you continue your journey with Redux and side effect management, you might also be interested in exploring other state management patterns. Check out our article on Buzzing with Redux Bees for an alternative approach to Redux side effects.
By embracing redux-loop, you’re not just managing state; you’re orchestrating a symphony of effects, all while keeping your code pure and your architecture clean. Happy looping!