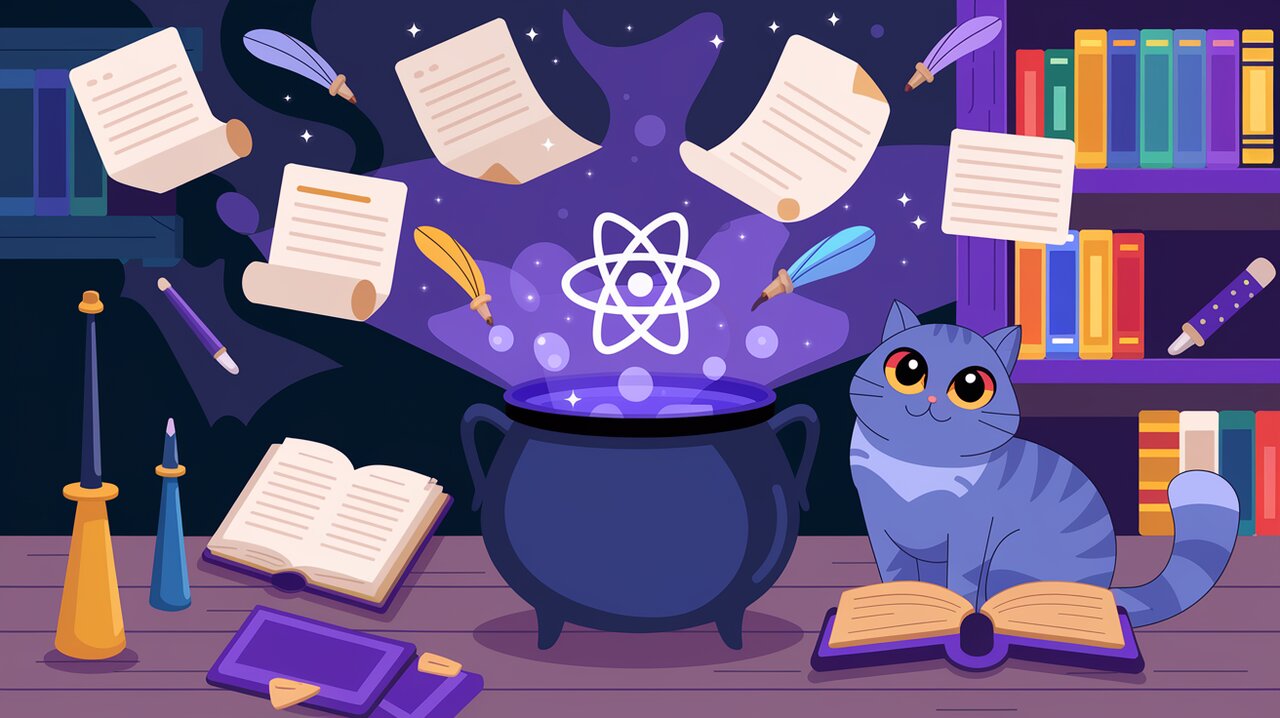
Markdown Wizardry with @uiw/react-md-editor: Conjure Powerful Content in React
In the realm of web development, crafting rich, interactive content has become a crucial aspect of modern applications. Enter @uiw/react-md-editor, a powerful React component that brings the magic of Markdown editing to your fingertips. This versatile library empowers developers to seamlessly integrate a feature-rich Markdown editor into their React applications, complete with live preview, syntax highlighting, and a host of customization options.
Unveiling the Enchanted Features
Before we dive into the incantations of code, let’s explore the magical features that make @uiw/react-md-editor a must-have tool in your React spellbook:
- 📝 Live Preview: Witness your Markdown transform in real-time as you type.
- 🎨 Syntax Highlighting: Illuminate your code blocks with vibrant colors.
- 🌙 Dark Mode Support: Adapt to the preferences of night owls and daylight dwellers alike.
- 🧰 Customizable Toolbar: Craft your perfect editing environment with ease.
- 📏 Adjustable Height: Flex your editor to fit any space in your UI.
- 🔒 XSS Protection: Keep your content safe from malicious incantations.
- 🖼️ Image Support: Conjure visuals directly into your Markdown.
- 📊 Math and Diagram Rendering: Bring complex formulas and flowcharts to life.
Summoning the Library
To begin your journey with @uiw/react-md-editor, you’ll need to summon it into your project. Open your terminal and chant one of these incantations:
npm install @uiw/react-md-editor
Or if you prefer the yarn familiar:
yarn add @uiw/react-md-editor
Casting Your First Spell
Now that you’ve acquired this powerful artifact, let’s cast our first spell to create a basic Markdown editor. Prepare your React component and follow along:
The Simple Incantation
import React, { useState } from 'react';
import MDEditor from '@uiw/react-md-editor';
const SimpleEditor: React.FC = () => {
const [value, setValue] = useState("# Welcome to the magical realm of Markdown!");
return (
<div className="container">
<MDEditor
value={value}
onChange={setValue}
/>
</div>
);
};
export default SimpleEditor;
In this enchanted snippet, we’ve conjured a basic Markdown editor. The useState
hook manages our editor’s content, while the MDEditor
component brings the editing magic to life. As you type, the onChange
function updates the state, creating a seamless editing experience.
Revealing the Preview
To gaze upon your formatted Markdown without entering the editing realm, you can use the Markdown
subcomponent:
import React from 'react';
import MDEditor from '@uiw/react-md-editor';
const MarkdownPreview: React.FC = () => {
const markdownContent = "## Behold, the power of Markdown!\n\nWith great formatting comes great responsibility.";
return (
<div className="container">
<MDEditor.Markdown source={markdownContent} />
</div>
);
};
export default MarkdownPreview;
This spell renders your Markdown content in its fully formatted glory, perfect for displaying user-generated content or documentation.
Advanced Enchantments
As you grow more comfortable with the basic spells, it’s time to delve into the advanced tomes of @uiw/react-md-editor. These powerful incantations will allow you to bend the editor to your will and create truly magical user experiences.
Customizing the Toolbar
The toolbar is your wand for Markdown manipulation. Let’s customize it to fit your specific needs:
import React, { useState } from 'react';
import MDEditor, { commands } from '@uiw/react-md-editor';
const CustomToolbarEditor: React.FC = () => {
const [value, setValue] = useState("# Customize your magical toolbar");
return (
<MDEditor
value={value}
onChange={setValue}
commands={[
commands.bold, commands.italic, commands.strikethrough,
commands.hr, commands.title, commands.divider,
commands.link, commands.quote, commands.code
]}
extraCommands={[
commands.codeEdit,
commands.codeLive,
commands.codePreview
]}
/>
);
};
export default CustomToolbarEditor;
This incantation crafts a toolbar with a specific set of formatting options. The commands
prop determines the main toolbar items, while extraCommands
adds additional tools to the right side of the toolbar.
Summoning the Dark Mode
For those who prefer to work under the cover of darkness, @uiw/react-md-editor offers a built-in dark mode. To invoke this shadowy realm, you need but whisper to the document’s root:
import React, { useEffect } from 'react';
import MDEditor from '@uiw/react-md-editor';
const DarkModeEditor: React.FC = () => {
useEffect(() => {
document.documentElement.setAttribute('data-color-mode', 'dark');
}, []);
return (
<MDEditor
value="# Welcome to the dark side of Markdown"
onChange={(val) => console.log(val)}
/>
);
};
export default DarkModeEditor;
This spell envelops your editor in a soothing dark theme, perfect for late-night coding sessions or those who simply prefer a darker aesthetic.
Conjuring Custom Previews
For those seeking to push the boundaries of Markdown rendering, @uiw/react-md-editor allows you to infuse your previews with custom magic. Behold, a spell that brings mathematical formulas to life:
import React, { useState } from 'react';
import MDEditor from '@uiw/react-md-editor';
import katex from 'katex';
import 'katex/dist/katex.css';
const MathEditor: React.FC = () => {
const [value, setValue] = useState("# Math Magic\n\n$$ E = mc^2 $$");
return (
<MDEditor
value={value}
onChange={setValue}
previewOptions={{
components: {
code: ({ inline, children = [], className, ...props }) => {
const txt = children[0] || '';
if (inline) {
if (typeof txt === 'string' && /^\$\$(.*)\$\$/.test(txt)) {
const html = katex.renderToString(txt.replace(/^\$\$(.*)\$\$/, '$1'), {
throwOnError: false,
});
return <code dangerouslySetInnerHTML={{ __html: html }} />;
}
return <code>{txt}</code>;
}
return null;
},
},
}}
/>
);
};
export default MathEditor;
This powerful incantation integrates KaTeX, allowing you to render complex mathematical equations within your Markdown. The custom code
component in previewOptions
intercepts LaTeX-style math expressions and transforms them into beautifully rendered formulas.
Conclusion: Mastering the Markdown Arts
As we conclude our magical journey through the realms of @uiw/react-md-editor, you now possess the knowledge to weave powerful Markdown editing experiences into your React applications. From basic editing to advanced customizations, this library offers a versatile set of tools to enhance your content creation process.
Remember, like any powerful artifact, @uiw/react-md-editor reveals its true potential with practice and experimentation. Don’t hesitate to explore its depths, combine different features, and create unique editing experiences tailored to your project’s needs.
As you continue to master the Markdown arts, may your code be bug-free and your content richly formatted. Happy editing, fellow React sorcerers!