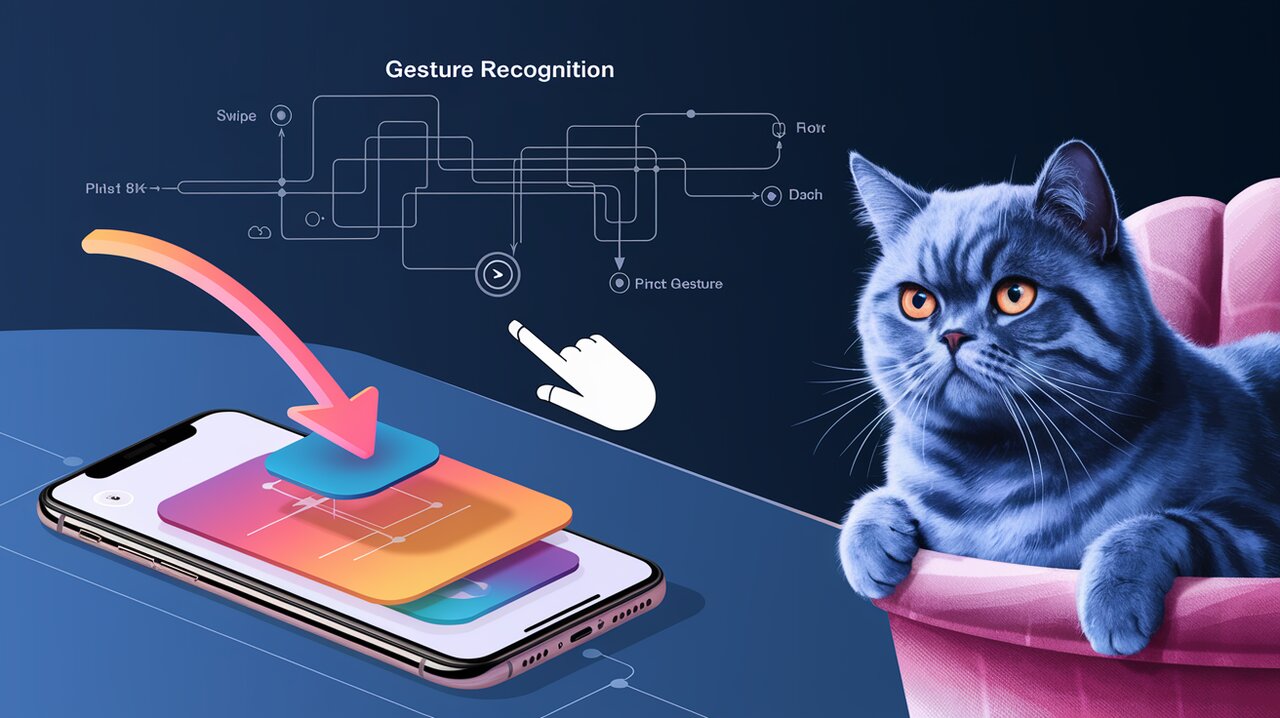
Unleashing Smooth Gestures with React Native Gesture Handler
React Native Gesture Handler is a powerful library that brings native-driven gesture management to React Native applications. By leveraging platform-specific touch handling systems, it offers a more responsive and fluid user experience compared to React Native’s built-in touch system. Let’s dive into how you can use this library to create smooth, performant gesture interactions in your apps.
Features
React Native Gesture Handler provides several key features that make it an essential tool for mobile developers:
- Native-driven gestures: Gestures are recognized and tracked in the UI thread, resulting in smoother interactions.
- Declarative API: Easily define complex gesture handling logic using a clear and intuitive API.
- Platform consistency: Ensures consistent behavior across iOS and Android platforms.
- Performance optimizations: Designed to work efficiently, even with complex gesture combinations.
- Reanimated integration: Seamlessly works with Reanimated for high-performance animations.
Installation
To get started with React Native Gesture Handler, you’ll need to install it in your project. Open your terminal and run one of the following commands:
npm install react-native-gesture-handler
or if you prefer using Yarn:
yarn add react-native-gesture-handler
After installation, you’ll need to wrap your app’s root component with GestureHandlerRootView
. This step is crucial for the library to function correctly:
import { GestureHandlerRootView } from 'react-native-gesture-handler';
export default function App() {
return (
<GestureHandlerRootView style={{ flex: 1 }}>
{/* Your app content */}
</GestureHandlerRootView>
);
}
Basic Usage
Simple Tap Gesture
Let’s start with a basic tap gesture. We’ll create a simple button that responds to taps:
import React from 'react';
import { Text, View } from 'react-native';
import { Gesture, GestureDetector } from 'react-native-gesture-handler';
export default function TapExample() {
const tap = Gesture.Tap()
.onStart(() => {
console.log('Tap detected');
});
return (
<GestureDetector gesture={tap}>
<View style={{ padding: 20, backgroundColor: 'lightblue' }}>
<Text>Tap me!</Text>
</View>
</GestureDetector>
);
}
This code creates a simple tappable area. When tapped, it logs a message to the console. The Gesture.Tap()
method creates a tap gesture recognizer, and GestureDetector
wraps the view that should respond to this gesture.
Pan Gesture
Now, let’s implement a more complex gesture - panning (dragging):
import React from 'react';
import { StyleSheet, View } from 'react-native';
import { Gesture, GestureDetector } from 'react-native-gesture-handler';
import Animated, { useAnimatedStyle, useSharedValue } from 'react-native-reanimated';
export default function PanExample() {
const translateX = useSharedValue(0);
const translateY = useSharedValue(0);
const pan = Gesture.Pan()
.onUpdate((event) => {
translateX.value += event.changeX;
translateY.value += event.changeY;
});
const animatedStyle = useAnimatedStyle(() => {
return {
transform: [
{ translateX: translateX.value },
{ translateY: translateY.value },
],
};
});
return (
<GestureDetector gesture={pan}>
<Animated.View style={[styles.box, animatedStyle]} />
</GestureDetector>
);
}
const styles = StyleSheet.create({
box: {
width: 100,
height: 100,
backgroundColor: 'blue',
borderRadius: 20,
},
});
This example creates a blue box that can be dragged around the screen. We use Gesture.Pan()
to create a pan gesture recognizer and update the position of the box using Reanimated’s useSharedValue
and useAnimatedStyle
.
Advanced Usage
Composing Gestures
React Native Gesture Handler allows you to compose multiple gestures for more complex interactions:
import React from 'react';
import { StyleSheet, View } from 'react-native';
import { Gesture, GestureDetector } from 'react-native-gesture-handler';
import Animated, { useAnimatedStyle, useSharedValue } from 'react-native-reanimated';
export default function ComposedGestureExample() {
const scale = useSharedValue(1);
const savedScale = useSharedValue(1);
const rotation = useSharedValue(0);
const savedRotation = useSharedValue(0);
const pinch = Gesture.Pinch()
.onUpdate((event) => {
scale.value = savedScale.value * event.scale;
})
.onEnd(() => {
savedScale.value = scale.value;
});
const rotate = Gesture.Rotation()
.onUpdate((event) => {
rotation.value = savedRotation.value + event.rotation;
})
.onEnd(() => {
savedRotation.value = rotation.value;
});
const composed = Gesture.Simultaneous(pinch, rotate);
const animatedStyle = useAnimatedStyle(() => {
return {
transform: [
{ scale: scale.value },
{ rotateZ: `${rotation.value}rad` },
],
};
});
return (
<GestureDetector gesture={composed}>
<Animated.View style={[styles.box, animatedStyle]} />
</GestureDetector>
);
}
const styles = StyleSheet.create({
box: {
width: 200,
height: 200,
backgroundColor: 'green',
borderRadius: 20,
},
});
This example combines pinch and rotation gestures, allowing the user to simultaneously scale and rotate the green box.
Manual Gesture Handling
For cases where you need more control over gesture recognition, you can use the Manual gesture:
import React from 'react';
import { StyleSheet, View } from 'react-native';
import { Gesture, GestureDetector } from 'react-native-gesture-handler';
export default function ManualGestureExample() {
const manual = Gesture.Manual()
.onTouchesDown((event, manager) => {
if (event.numberOfTouches === 2) {
manager.activate();
}
})
.onTouchesMove((event, manager) => {
if (event.numberOfTouches !== 2) {
manager.fail();
}
})
.onTouchesUp((event, manager) => {
manager.end();
});
return (
<GestureDetector gesture={manual}>
<View style={styles.container}>
{/* Your content here */}
</View>
</GestureDetector>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
This example demonstrates how to use the Manual gesture to recognize a custom two-finger gesture. The gesture activates when two fingers touch the screen and fails if the number of touches changes.
Conclusion
React Native Gesture Handler provides a powerful and flexible system for implementing complex gesture interactions in your React Native applications. By leveraging native gesture recognition, it offers superior performance and a more natural feel compared to JavaScript-based solutions.
As you’ve seen, the library’s declarative API makes it straightforward to implement common gestures like taps and pans, while also providing the flexibility to compose gestures and create custom interactions. When combined with libraries like Reanimated, you can create fluid, high-performance animations that respond to user gestures.
By mastering React Native Gesture Handler, you’ll be able to create more engaging and interactive mobile experiences that feel truly native. As you continue to explore its capabilities, you’ll discover even more ways to enhance your app’s user interface and interactions.