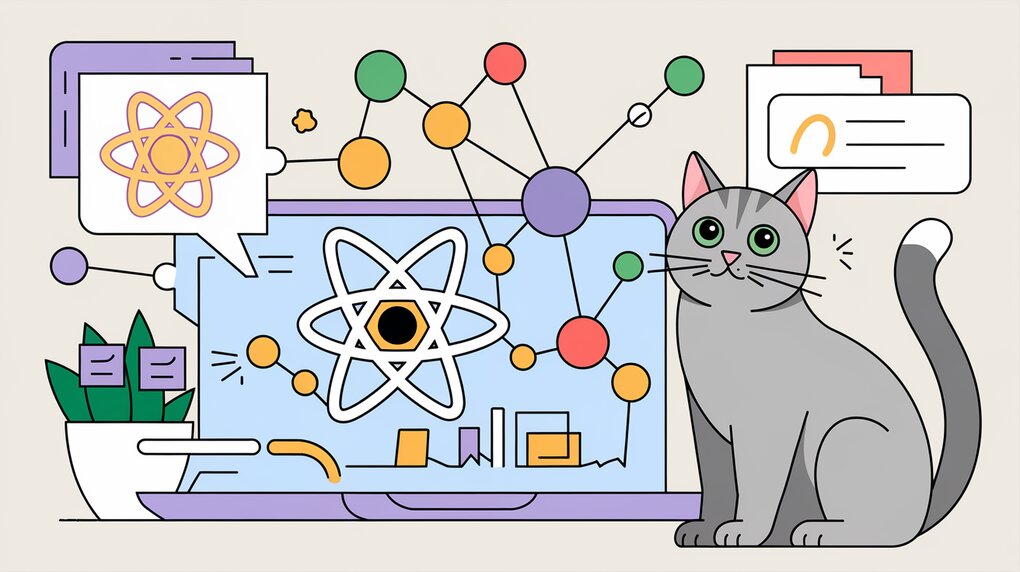
Jotai: Unleashing Atomic State Management in React
Jotai is a state management library for React that introduces an atomic approach to handling global state. Inspired by Recoil, Jotai allows developers to manage state through small, isolated units called atoms. These atoms can be combined to form complex state structures, making Jotai an excellent choice for both simple and enterprise-level applications. By optimizing renders based on atom dependencies, Jotai reduces unnecessary re-renders and eliminates the need for memoization, providing a more efficient way to manage state.
Features
- Minimal Core API: Jotai’s core is lightweight, with a simple API that’s easy to learn and integrate.
- TypeScript Support: Built with TypeScript in mind, Jotai offers strong typing and compatibility.
- Flexible Integration: Works seamlessly with frameworks like Next.js, Gatsby, and React Native.
- Utilities and Extensions: Offers additional tools for advanced use cases, such as localStorage persistence and integration with React Query.
Installation
To get started with Jotai, you need to add it to your project. You can use either npm or yarn:
# Using npm
npm install jotai
# Using yarn
yarn add jotai
Basic Usage
Jotai’s core concept revolves around atoms. An atom represents a piece of state and can be used similarly to React’s useState
hook.
Creating Atoms
Here’s how you can create a simple atom:
import { atom } from 'jotai';
const countAtom = atom(0);
Using Atoms in Components
To use an atom in a component, you can utilize the useAtom
hook:
import { useAtom } from 'jotai';
function Counter() {
const [count, setCount] = useAtom(countAtom);
return (
<div>
<h1>{count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Advanced Usage
Jotai allows you to create derived atoms, which can compute values based on other atoms. This feature is particularly useful for creating dynamic state dependencies.
Derived Atoms
Derived atoms can be read-only or writable. Here’s an example of a read-only derived atom:
const doubledCountAtom = atom((get) => get(countAtom) * 2);
function DoubleCounter() {
const [doubledCount] = useAtom(doubledCountAtom);
return <h2>Doubled Count: {doubledCount}</h2>;
}
Writable Derived Atoms
Writable derived atoms allow you to define both read and write functions:
const decrementCountAtom = atom(
(get) => get(countAtom),
(get, set) => set(countAtom, get(countAtom) - 1)
);
function DecrementCounter() {
const [count, decrement] = useAtom(decrementCountAtom);
return (
<div>
<h1>{count}</h1>
<button onClick={decrement}>Decrement</button>
</div>
);
}
Asynchronous Atoms
Jotai also supports asynchronous operations within atoms. This can be useful for fetching data:
const urlAtom = atom('https://api.example.com/data');
const fetchDataAtom = atom(async (get) => {
const response = await fetch(get(urlAtom));
return await response.json();
});
function DataFetcher() {
const [data] = useAtom(fetchDataAtom);
return <div>Data: {JSON.stringify(data)}</div>;
}
Conclusion
Jotai is a powerful and flexible state management library that simplifies the way you manage state in React applications. By breaking down state into atomic units, Jotai provides a scalable solution that can handle anything from small projects to large-scale applications. Its minimal API, combined with the ability to create derived and asynchronous atoms, makes Jotai a versatile tool for developers looking to optimize their React applications. Whether you’re building a simple counter or a complex data-driven app, Jotai’s atomic approach offers a fresh and efficient way to manage state.