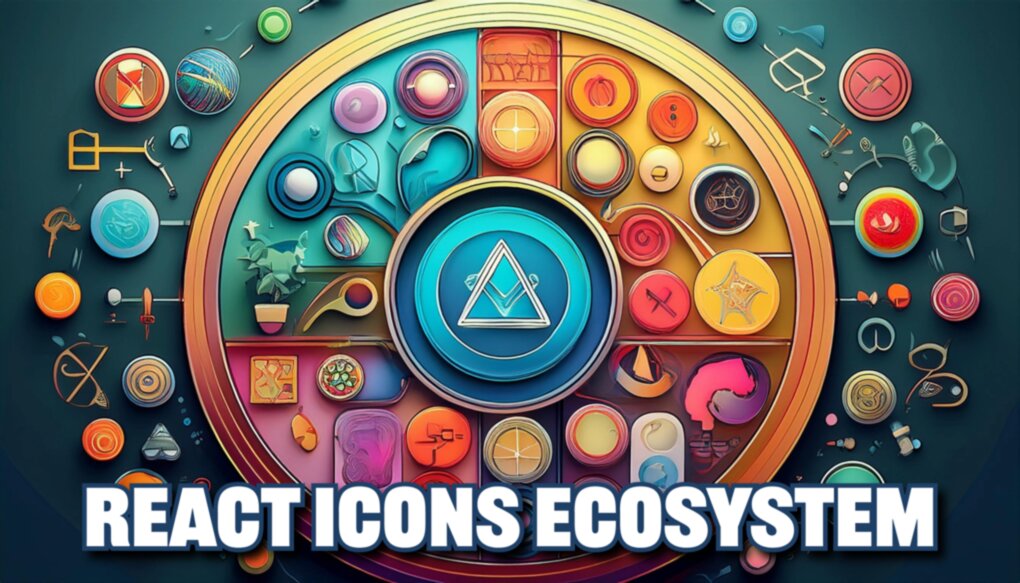
The react-icons library is a powerful tool for React developers, offering a simple way to include popular icon sets in your projects. With support for ES6 imports, it allows you to include only the icons you need, keeping your bundle size optimized. Let’s dive into how you can leverage this library to enhance your React applications.
Installation
To get started with react-icons, you’ll need to install it in your project. Open your terminal and run one of the following commands:
npm install react-icons
# or
yarn add react-icons
Basic Usage
Once installed, you can start using icons from various popular sets. Here’s a simple example:
import React from 'react';
import { FaBeer } from "react-icons/fa";
const BeerQuestion: React.FC = () => {
return (
<h3>
Lets go for a <FaBeer />?
</h3>
);
}
export default BeerQuestion;
In this example, we’re importing the beer icon from the Font Awesome set. The fa
in react-icons/fa
stands for Font Awesome. Each icon set has its own subfolder that you import from.
Advanced Usage
Using Icons from Different Sets
react-icons provides access to multiple icon sets. Here’s how you can use icons from different sets in the same component:
import React from 'react';
import { FaReact } from "react-icons/fa";
import { AiFillAndroid } from "react-icons/ai";
import { IoLogoApple } from "react-icons/io";
const TechIcons: React.FC = () => {
return (
<div>
<FaReact /> React
<AiFillAndroid /> Android
<IoLogoApple /> iOS
</div>
);
}
export default TechIcons;
Customizing Icons
You can easily customize icons by passing props:
import React from 'react';
import { FaHeart } from "react-icons/fa";
const CustomHeart: React.FC = () => {
return (
<FaHeart size={48} color="red" />
);
}
export default CustomHeart;
Using IconContext for Global Styling
If you want to apply consistent styling to all icons in a section of your app, you can use the IconContext
provider:
import React from 'react';
import { IconContext } from "react-icons";
import { FaFolder, FaFile } from "react-icons/fa";
const FileExplorer: React.FC = () => {
return (
<IconContext.Provider value={{ color: "blue", size: "1.5em", className: "global-icon" }}>
<div>
<FaFolder /> Documents
<FaFile /> Report.pdf
</div>
</IconContext.Provider>
);
}
export default FileExplorer;
This will apply the specified styles to all icons within the IconContext.Provider
.
Dynamic Icon Selection
You can also dynamically select icons based on conditions:
import React from 'react';
import { FaSun, FaMoon } from "react-icons/fa";
interface ThemeToggleProps {
isDarkMode: boolean;
}
const ThemeToggle: React.FC<ThemeToggleProps> = ({ isDarkMode }) => {
const Icon = isDarkMode ? FaSun : FaMoon;
return (
<button>
<Icon /> Toggle Theme
</button>
);
}
export default ThemeToggle;
Conclusion
The react-icons library provides a seamless way to incorporate a wide variety of icons into your React projects. Its modular nature allows for efficient bundle sizes, while the straightforward API makes it easy to use and customize icons to fit your design needs.
Remember to explore the different icon sets available and leverage features like IconContext
for consistent styling across your application. With react-icons, you can significantly enhance the visual appeal and user experience of your React applications without the overhead of managing icon fonts or SVG files manually.
Happy coding, and may your React projects be filled with beautiful, scalable icons!