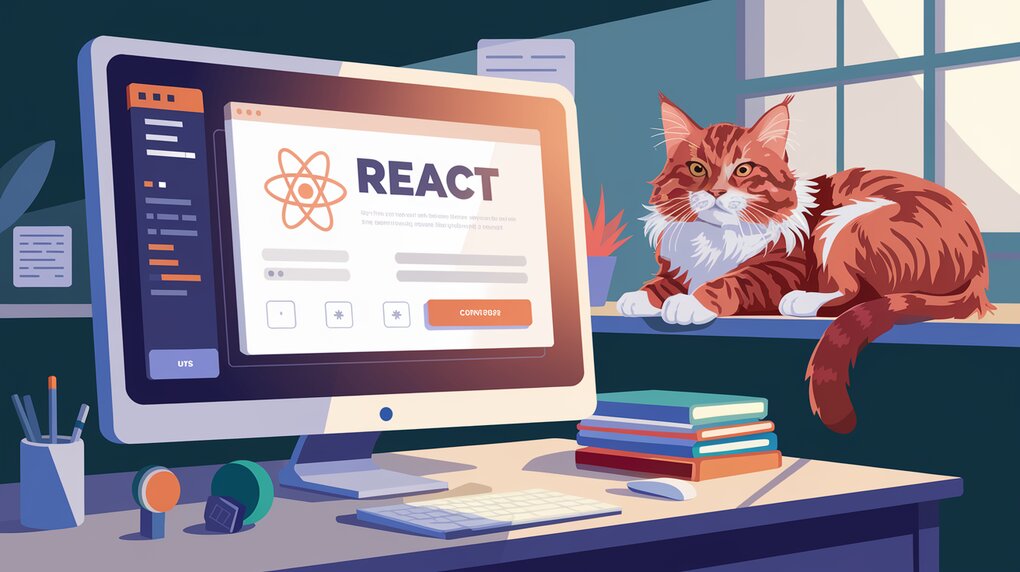
Mastering React Modals: Unleash the Power of react-modal
React-modal is a powerful and flexible library that allows developers to create accessible modal dialogs in React applications. It provides a simple yet customizable way to display important information, gather user input, or present interactive content without navigating away from the current page. With react-modal, you can easily create modals that enhance user experience and maintain accessibility standards.
Features
React-modal comes packed with a variety of features that make it a go-to choice for developers:
- Accessibility: Built-in support for screen readers and keyboard navigation.
- Customization: Extensive styling options to match your application’s design.
- Flexibility: Supports various content types, including forms, images, and complex layouts.
- Ease of use: Simple API for quick implementation and management of modal states.
- Portal rendering: Modals are rendered outside the main React component tree for better z-index management.
Installation
To get started with react-modal, you can install it using npm or yarn:
npm install react-modal
or
yarn add react-modal
For React CDN applications, you can include the library via a script tag:
<script src="https://cdnjs.cloudflare.com/ajax/libs/react-modal/3.14.3/react-modal.min.js" integrity="sha512-MY2jfK3DBnVzdS2V8MXo5lRtr0mNRroUI9hoLVv2/yL3vrJTam3VzASuKQ96fLEpyYIT4a8o7YgtUs5lPjiLVQ==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
Basic Usage
Let’s start with a simple example to demonstrate how to use react-modal in your React application:
import React, { useState } from 'react';
import Modal from 'react-modal';
// Set the app element for accessibility
Modal.setAppElement('#root');
const App: React.FC = () => {
const [modalIsOpen, setModalIsOpen] = useState(false);
const openModal = () => setModalIsOpen(true);
const closeModal = () => setModalIsOpen(false);
return (
<div>
<button onClick={openModal}>Open Modal</button>
<Modal
isOpen={modalIsOpen}
onRequestClose={closeModal}
contentLabel="Example Modal"
>
<h2>Hello, I'm a modal!</h2>
<button onClick={closeModal}>Close</button>
<div>This is the modal content</div>
</Modal>
</div>
);
};
export default App;
In this example, we import the Modal component and use the useState
hook to manage the modal’s open/close state. The isOpen
prop controls the visibility of the modal, while onRequestClose
handles the closing action.
Advanced Usage
React-modal offers various advanced features for more complex scenarios. Let’s explore some of them:
Custom Styling
You can customize the appearance of your modal using inline styles or CSS classes:
import React, { useState } from 'react';
import Modal from 'react-modal';
const customStyles = {
content: {
top: '50%',
left: '50%',
right: 'auto',
bottom: 'auto',
marginRight: '-50%',
transform: 'translate(-50%, -50%)',
backgroundColor: '#f0f0f0',
borderRadius: '8px',
padding: '20px',
},
overlay: {
backgroundColor: 'rgba(0, 0, 0, 0.75)'
}
};
const App: React.FC = () => {
const [modalIsOpen, setModalIsOpen] = useState(false);
return (
<div>
<button onClick={() => setModalIsOpen(true)}>Open Styled Modal</button>
<Modal
isOpen={modalIsOpen}
onRequestClose={() => setModalIsOpen(false)}
style={customStyles}
contentLabel="Styled Modal Example"
>
<h2>Custom Styled Modal</h2>
<p>This modal has custom styles applied to it.</p>
<button onClick={() => setModalIsOpen(false)}>Close</button>
</Modal>
</div>
);
};
export default App;
Handling Modal Actions
You can add custom logic to handle actions within the modal:
import React, { useState } from 'react';
import Modal from 'react-modal';
const App: React.FC = () => {
const [modalIsOpen, setModalIsOpen] = useState(false);
const [formData, setFormData] = useState({ name: '', email: '' });
const handleSubmit = (e: React.FormEvent) => {
e.preventDefault();
console.log('Form submitted:', formData);
setModalIsOpen(false);
};
return (
<div>
<button onClick={() => setModalIsOpen(true)}>Open Form Modal</button>
<Modal
isOpen={modalIsOpen}
onRequestClose={() => setModalIsOpen(false)}
contentLabel="Form Modal Example"
>
<h2>Submit Your Information</h2>
<form onSubmit={handleSubmit}>
<input
type="text"
placeholder="Name"
value={formData.name}
onChange={(e) => setFormData({ ...formData, name: e.target.value })}
/>
<input
type="email"
placeholder="Email"
value={formData.email}
onChange={(e) => setFormData({ ...formData, email: e.target.value })}
/>
<button type="submit">Submit</button>
</form>
</Modal>
</div>
);
};
export default App;
Accessibility Features
React-modal provides built-in accessibility features. You can enhance them further:
import React, { useState } from 'react';
import Modal from 'react-modal';
Modal.setAppElement('#root');
const App: React.FC = () => {
const [modalIsOpen, setModalIsOpen] = useState(false);
return (
<div>
<button onClick={() => setModalIsOpen(true)}>Open Accessible Modal</button>
<Modal
isOpen={modalIsOpen}
onRequestClose={() => setModalIsOpen(false)}
contentLabel="Accessible Modal Example"
aria={{
labelledby: "heading",
describedby: "full_description"
}}
>
<h2 id="heading">Accessible Modal</h2>
<div id="full_description">
<p>This modal is fully accessible, with proper ARIA attributes.</p>
<button onClick={() => setModalIsOpen(false)}>Close Modal</button>
</div>
</Modal>
</div>
);
};
export default App;
Conclusion
React-modal is a versatile and powerful library for creating modal dialogs in React applications. Its focus on accessibility, customization options, and ease of use make it an excellent choice for developers looking to enhance their user interfaces. By mastering react-modal, you can create engaging and interactive experiences that improve user engagement and application functionality. Whether you’re building a simple notification system or complex multi-step forms, react-modal provides the tools you need to create polished and professional modal dialogs.