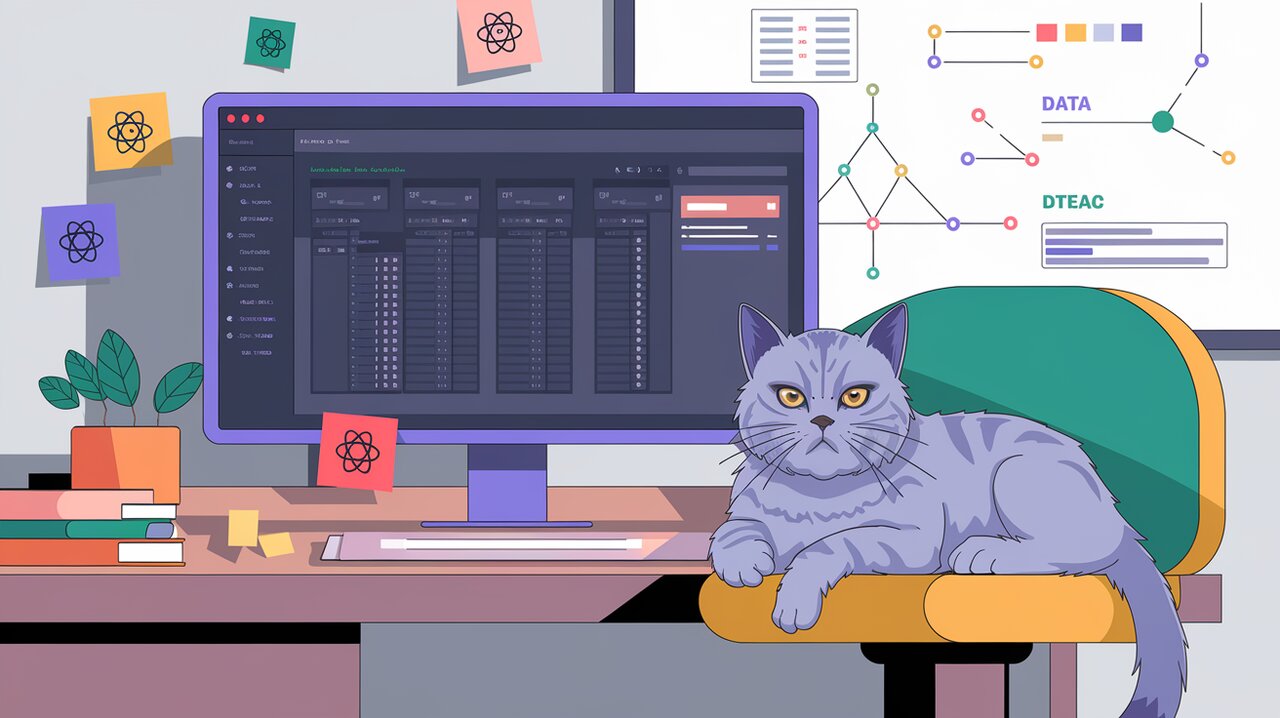
Material React Table: Conjuring Data Grid Magic with React
Material React Table is a powerful and flexible data grid library for React applications that combines the extensive API of TanStack Table with the polished UI components of Material UI. This magical combination allows developers to create feature-rich, customizable, and visually appealing tables with ease.
Unleashing the Power of Material React Table
At its core, Material React Table offers a comprehensive set of features that cater to various data presentation needs:
-
Efficient Bundle Size: With a compact footprint of 37-53 KB (depending on imported components), Material React Table ensures your application remains lightweight.
-
Customization Options: From pre-built components to fully custom implementations, the library provides flexibility to suit your specific requirements.
-
Advanced Features: Beyond standard table functionalities, Material React Table offers advanced capabilities such as virtualization, aggregation, grouping, and full CRUD operations.
-
TypeScript Support: Enjoy the benefits of type safety with Material React Table’s robust TypeScript support.
Conjuring Your First Table
Let’s dive into creating a basic table using Material React Table. First, ensure you have the necessary dependencies installed:
npm install material-react-table @mui/material @emotion/react @emotion/styled
Now, let’s craft our first table:
import { useMemo } from 'react';
import { MaterialReactTable, useMaterialReactTable } from 'material-react-table';
type Person = {
name: string;
age: number;
city: string;
};
const data: Person[] = [
{ name: 'John Doe', age: 30, city: 'New York' },
{ name: 'Jane Smith', age: 25, city: 'London' },
// Add more data...
];
const Example = () => {
const columns = useMemo(
() => [
{
accessorKey: 'name',
header: 'Name',
},
{
accessorKey: 'age',
header: 'Age',
},
{
accessorKey: 'city',
header: 'City',
},
],
[]
);
const table = useMaterialReactTable({
columns,
data,
enableRowSelection: true,
enableColumnOrdering: true,
enableGlobalFilter: true,
});
return <MaterialReactTable table={table} />;
};
export default Example;
This example demonstrates a basic table with sorting, filtering, and row selection capabilities. The useMaterialReactTable
hook allows you to define table options, while the MaterialReactTable
component renders the actual table.
Advanced Incantations
Summoning Detail Panels
To add expandable row details, you can use the renderDetailPanel
prop:
const table = useMaterialReactTable({
// ... other options
renderDetailPanel: ({ row }) => (
<Box>
<Typography>City: {row.original.city}</Typography>
</Box>
),
});
Crafting Custom Cell Renders
For more control over cell rendering, you can define custom cell components:
const columns = useMemo(
() => [
// ... other columns
{
accessorKey: 'status',
header: 'Status',
Cell: ({ cell }) => (
<Chip
label={cell.getValue<string>()}
color={cell.getValue<string>() === 'Active' ? 'success' : 'error'}
/>
),
},
],
[]
);
Invoking Data Export Spells
Material React Table makes it easy to add export functionality to your tables:
import { MRT_ExportData } from 'material-react-table';
const ExportButton = ({ table }) => (
<Button
onClick={() => MRT_ExportData(table.getPrePaginationRowModel().rows, ['name', 'age', 'city'])}
>
Export
</Button>
);
Mastering the Art of Customization
Material React Table offers extensive customization options. You can override styles, create custom toolbars, and even build your own table structure using the useMaterialReactTable
hook.
For instance, to create a custom top toolbar:
const table = useMaterialReactTable({
// ... other options
renderTopToolbar: ({ table }) => (
<Box>
<MRT_GlobalFilterTextField table={table} />
<MRT_ToggleFiltersButton table={table} />
<ExportButton table={table} />
</Box>
),
});
Conclusion: Unleashing Data Grid Magic
Material React Table empowers developers to create sophisticated, performant, and visually appealing data grids with minimal effort. Its combination of TanStack Table’s powerful API and Material UI’s sleek components makes it an excellent choice for React applications that require advanced data presentation capabilities.
As you continue your journey with Material React Table, explore its extensive documentation and examples to unlock even more magical features. Whether you’re building a simple data table or a complex data management system, Material React Table provides the tools you need to conjure impressive and functional user interfaces.
For more React library wizardry, check out our articles on React Beautiful DnD for drag-and-drop magic, and React Big Calendar for powerful date and event management. Happy coding, and may your tables be ever data-rich and user-friendly!