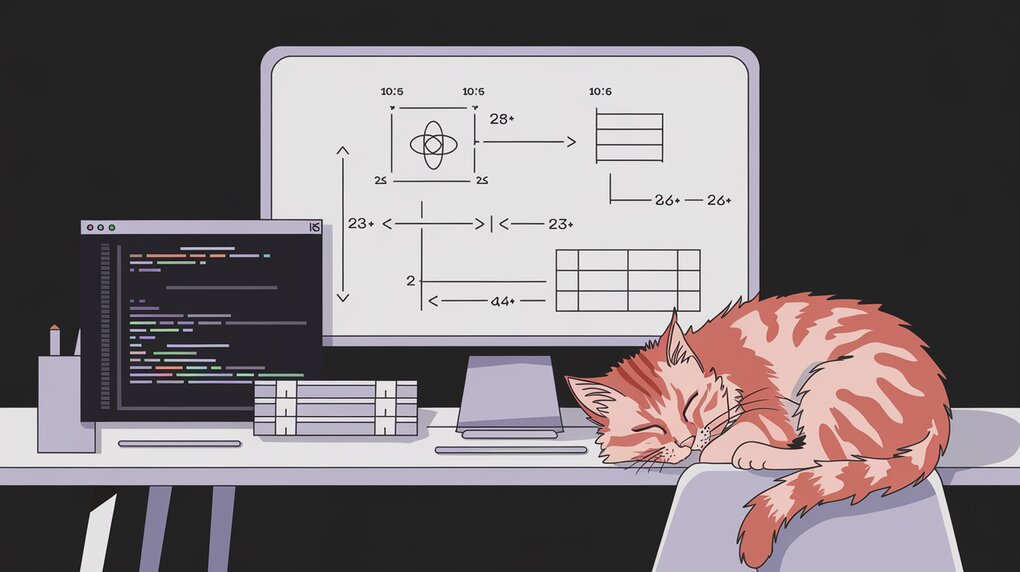
Measure Up Your React Components with react-measure
React applications often require precise knowledge of component dimensions for optimal layout and responsiveness. Enter react-measure, a powerful library that simplifies the process of computing and tracking measurements of React components. By leveraging the ResizeObserver API, react-measure provides a robust solution for detecting changes in element dimensions, making it an invaluable tool for developers seeking to create highly responsive and adaptive user interfaces.
Features
react-measure
offers several key features that make it stand out:
- Utilizes ResizeObserver for efficient dimension tracking
- Provides a polyfill for browsers lacking ResizeObserver support
- Offers flexible measurement options including client, offset, scroll, bounds, and margin
- Supports both component-based and higher-order component (HOC) usage patterns
- Allows for programmatic measurements
Installation
To get started with react-measure, you can install it using npm or yarn:
npm install react-measure --save
or
yarn add react-measure
Basic Usage
Measuring a Component
Let’s dive into a basic example of how to use react-measure to track the dimensions of a component:
import React, { useState } from 'react';
import Measure from 'react-measure';
const MeasuredComponent: React.FC = () => {
const [dimensions, setDimensions] = useState({ width: -1, height: -1 });
return (
<Measure
bounds
onResize={(contentRect) => {
setDimensions(contentRect.bounds || { width: -1, height: -1 });
}}
>
{({ measureRef }) => (
<div ref={measureRef} style={{ border: '1px solid #ccc', padding: '20px' }}>
<p>Width: {dimensions.width}px</p>
<p>Height: {dimensions.height}px</p>
</div>
)}
</Measure>
);
};
In this example, we wrap our component with the Measure
component from react-measure. The bounds
prop tells the component to measure the bounding rectangle of the element. The onResize
callback is called whenever the dimensions change, allowing us to update our state with the new measurements.
Responsive Design with Measurements
react-measure can be particularly useful for creating responsive layouts. Here’s an example of how you might use it to adjust a component’s appearance based on its width:
import React, { useState } from 'react';
import Measure from 'react-measure';
import classNames from 'classnames';
const ResponsiveComponent: React.FC = () => {
const [dimensions, setDimensions] = useState({ width: -1 });
return (
<Measure
bounds
onResize={(contentRect) => {
setDimensions(contentRect.bounds || { width: -1 });
}}
>
{({ measureRef }) => (
<div
ref={measureRef}
className={classNames({
'large-layout': dimensions.width >= 600,
'small-layout': dimensions.width < 600,
})}
>
{dimensions.width >= 600 ? (
<h2>Large Layout Content</h2>
) : (
<h3>Small Layout Content</h3>
)}
<p>Current width: {dimensions.width}px</p>
</div>
)}
</Measure>
);
};
This component will change its layout and content based on whether its width is above or below 600 pixels, demonstrating how react-measure can be used to create adaptive user interfaces.
Advanced Usage
Using the withContentRect HOC
For more complex scenarios, react-measure provides a higher-order component called withContentRect
. This can be useful when you want to measure multiple aspects of an element or when you prefer a more declarative approach:
import React from 'react';
import { withContentRect } from 'react-measure';
interface Props {
measureRef: (element: HTMLElement | null) => void;
contentRect: {
bounds?: DOMRectReadOnly;
client?: DOMRectReadOnly;
offset?: DOMRectReadOnly;
};
}
const MyComponent: React.FC<Props> = ({ measureRef, contentRect }) => (
<div ref={measureRef} style={{ border: '1px solid #ccc', padding: '20px' }}>
<h2>Component Measurements</h2>
<p>Width: {contentRect.bounds?.width || 0}px</p>
<p>Height: {contentRect.bounds?.height || 0}px</p>
<p>Top: {contentRect.bounds?.top || 0}px</p>
<p>Left: {contentRect.bounds?.left || 0}px</p>
</div>
);
export default withContentRect('bounds')(MyComponent);
This approach allows you to access the measurement data directly as props, which can be particularly useful when working with TypeScript or when you need to measure multiple aspects of an element.
Measuring Specific Aspects
react-measure allows you to specify which aspects of an element you want to measure. You can choose from client
, offset
, scroll
, bounds
, and margin
:
import React from 'react';
import Measure from 'react-measure';
const DetailedMeasureComponent: React.FC = () => (
<Measure
client
offset
scroll
bounds
margin
onResize={(contentRect) => {
console.log('Client:', contentRect.client);
console.log('Offset:', contentRect.offset);
console.log('Scroll:', contentRect.scroll);
console.log('Bounds:', contentRect.bounds);
console.log('Margin:', contentRect.margin);
}}
>
{({ measureRef }) => (
<div ref={measureRef} style={{ border: '1px solid #ccc', padding: '20px', margin: '10px' }}>
<h2>Detailed Measurements</h2>
<p>Check the console for all measurement details</p>
</div>
)}
</Measure>
);
This example demonstrates how to measure all available aspects of an element, logging the results to the console. This can be particularly useful for debugging or when you need very specific information about an element’s dimensions and position.
Conclusion
react-measure
provides a powerful and flexible solution for measuring and tracking the dimensions of React components. Whether you’re creating responsive layouts, optimizing performance, or simply need to know the exact size of your components, react-measure offers an easy-to-use API that integrates seamlessly with your React applications.
By leveraging the ResizeObserver API and providing a polyfill for unsupported browsers, react-measure ensures broad compatibility while offering efficient and accurate measurements. Its support for both component-based usage and higher-order components allows developers to choose the approach that best fits their coding style and project requirements.
As web applications continue to demand more responsive and adaptive user interfaces, tools like react-measure become increasingly valuable. By incorporating react-measure into your development toolkit, you can create more dynamic, responsive, and user-friendly React applications with ease.