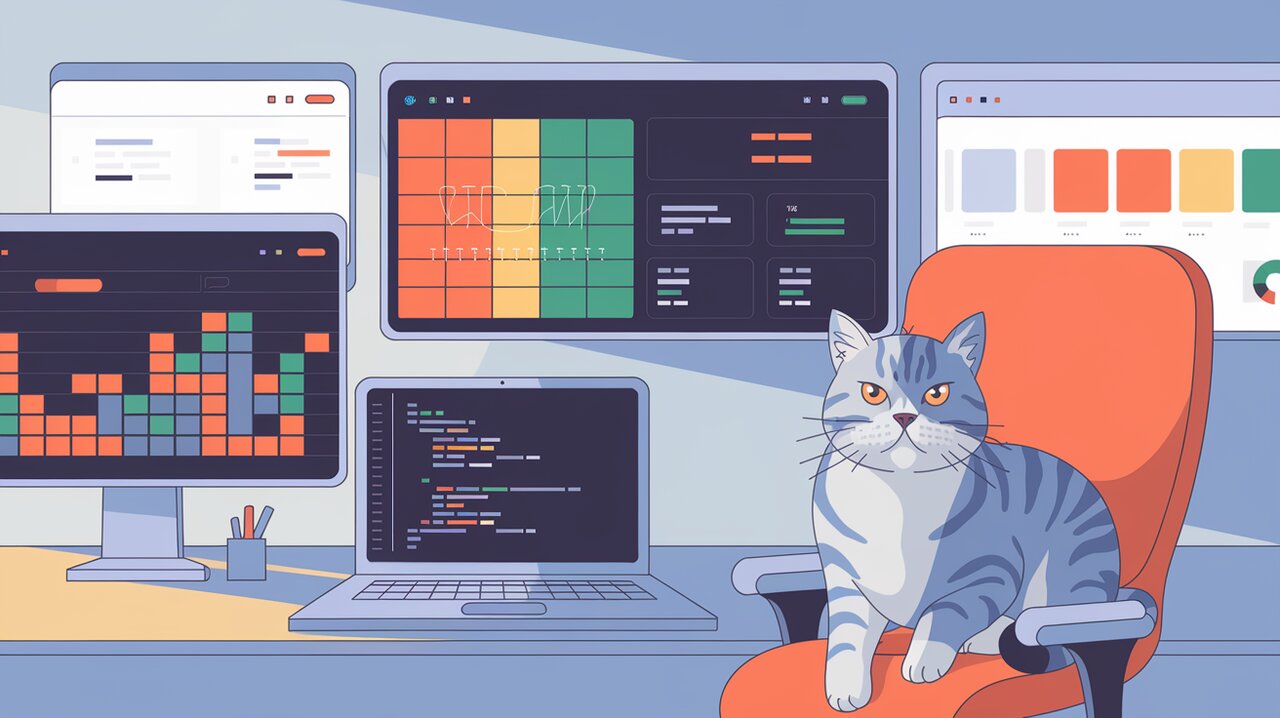
MUI X Data Grid: Supercharging Your React Tables with Style and Functionality
In the realm of React development, managing and displaying large sets of data efficiently can be a daunting task. Enter MUI X Data Grid, a powerful and flexible solution that takes the complexity out of creating feature-rich, performant data tables. Whether you’re building a simple list or a complex data management system, this library offers a comprehensive set of tools to elevate your React applications.
Unleashing the Power of MUI X Data Grid
MUI X Data Grid is more than just a table component. It’s a full-fledged data management solution that brings Material Design aesthetics and functionality to your React projects. With features like virtualization for handling large datasets, built-in sorting and filtering capabilities, and extensive customization options, it’s designed to meet the needs of both simple and complex use cases.
Key Features
- Virtualization: Efficiently render thousands of rows with optimal performance
- Sorting and Filtering: Built-in capabilities for data manipulation
- Pagination: Easy-to-implement page navigation for large datasets
- Column Resizing and Reordering: Customizable grid layout
- Cell Editing: In-place data modification capabilities
- Custom Rendering: Flexibility to define how cells and headers are displayed
- Keyboard Navigation: Enhanced accessibility and user experience
- Localization: Support for multiple languages and formats
- Tree Data: Hierarchical data representation
- Master Detail: Expandable rows for nested data visualization
Getting Started
Installation
To begin using MUI X Data Grid in your project, you’ll need to install it along with its peer dependencies. Here’s how you can do it using npm or yarn:
Using npm:
npm install @mui/x-data-grid @mui/material @emotion/react @emotion/styled
Using yarn:
yarn add @mui/x-data-grid @mui/material @emotion/react @emotion/styled
Basic Usage
Let’s dive into a simple example to get you started with MUI X Data Grid. We’ll create a basic table with a few columns and rows.
import * as React from 'react';
import { DataGrid, GridColDef, GridValueGetterParams } from '@mui/x-data-grid';
const columns: GridColDef[] = [
{ field: 'id', headerName: 'ID', width: 70 },
{ field: 'firstName', headerName: 'First name', width: 130 },
{ field: 'lastName', headerName: 'Last name', width: 130 },
{
field: 'age',
headerName: 'Age',
type: 'number',
width: 90,
},
];
const rows = [
{ id: 1, lastName: 'Snow', firstName: 'Jon', age: 35 },
{ id: 2, lastName: 'Lannister', firstName: 'Cersei', age: 42 },
{ id: 3, lastName: 'Lannister', firstName: 'Jaime', age: 45 },
{ id: 4, lastName: 'Stark', firstName: 'Arya', age: 16 },
{ id: 5, lastName: 'Targaryen', firstName: 'Daenerys', age: null },
];
export default function BasicDataGrid() {
return (
<div style={{ height: 400, width: '100%' }}>
<DataGrid
rows={rows}
columns={columns}
initialState={{
pagination: {
paginationModel: { page: 0, pageSize: 5 },
},
}}
pageSizeOptions={[5, 10]}
checkboxSelection
/>
</div>
);
}
In this example, we define our columns with specific configurations like field names, header labels, and widths. The rows
array contains our data. The DataGrid
component then takes these props to render a fully functional table with pagination and checkbox selection.
Advanced Features
Custom Column Rendering
MUI X Data Grid allows you to customize how cells are rendered. This is particularly useful for formatting dates, adding icons, or creating interactive elements within cells.
import { DataGrid, GridColDef, GridRenderCellParams } from '@mui/x-data-grid';
import { IconButton } from '@mui/material';
import DeleteIcon from '@mui/icons-material/Delete';
const columns: GridColDef[] = [
// ... other columns
{
field: 'actions',
headerName: 'Actions',
width: 100,
renderCell: (params: GridRenderCellParams) => (
<IconButton onClick={() => handleDelete(params.row.id)}>
<DeleteIcon />
</IconButton>
),
},
];
const handleDelete = (id: number) => {
// Delete logic here
};
This example demonstrates how to add a custom delete button to each row, showcasing the flexibility of cell rendering in MUI X Data Grid.
Sorting and Filtering
Implementing sorting and filtering is straightforward with MUI X Data Grid. These features come built-in and can be easily customized to fit your needs.
import { DataGrid, GridColDef, GridToolbar } from '@mui/x-data-grid';
const columns: GridColDef[] = [
// ... column definitions
];
export default function SortFilterGrid() {
return (
<DataGrid
rows={rows}
columns={columns}
initialState={{
sorting: {
sortModel: [{ field: 'lastName', sort: 'asc' }],
},
filter: {
filterModel: {
items: [{ field: 'age', operator: '>', value: '30' }],
},
},
}}
components={{ Toolbar: GridToolbar }}
/>
);
}
This setup enables default sorting on the ‘lastName’ field and filters rows where the age is greater than 30. The GridToolbar
component adds UI controls for sorting and filtering.
Pagination and Row Selection
MUI X Data Grid offers robust pagination and row selection capabilities, enhancing user interaction with large datasets.
import { DataGrid, GridColDef, GridSelectionModel } from '@mui/x-data-grid';
import { useState } from 'react';
export default function PaginationSelectionGrid() {
const [selectionModel, setSelectionModel] = useState<GridSelectionModel>([]);
return (
<DataGrid
rows={rows}
columns={columns}
pagination
pageSize={5}
rowsPerPageOptions={[5, 10, 20]}
checkboxSelection
onSelectionModelChange={(newSelectionModel) => {
setSelectionModel(newSelectionModel);
}}
selectionModel={selectionModel}
/>
);
}
This example sets up pagination with options for different page sizes and enables checkbox selection. The selected rows are managed through React state, allowing for further processing of selected data.
Conclusion: Elevating Data Presentation with MUI X Data Grid
MUI X Data Grid stands out as a powerful tool in the React ecosystem for managing and displaying tabular data. Its rich feature set, from basic sorting and filtering to advanced customization options, makes it suitable for a wide range of applications. By leveraging its capabilities, developers can create sophisticated, performant, and user-friendly data grids with minimal effort.
As you explore the depths of MUI X Data Grid, you’ll discover its potential to significantly enhance the data interaction experience in your React applications. Whether you’re building complex dashboards or simple data views, this library provides the flexibility and functionality to meet your needs, all while maintaining the sleek aesthetics of Material Design.
Embrace the power of MUI X Data Grid and transform the way you handle data in your React projects. With its intuitive API and extensive documentation, you’re well-equipped to create stunning, functional data grids that will elevate your application’s user experience.