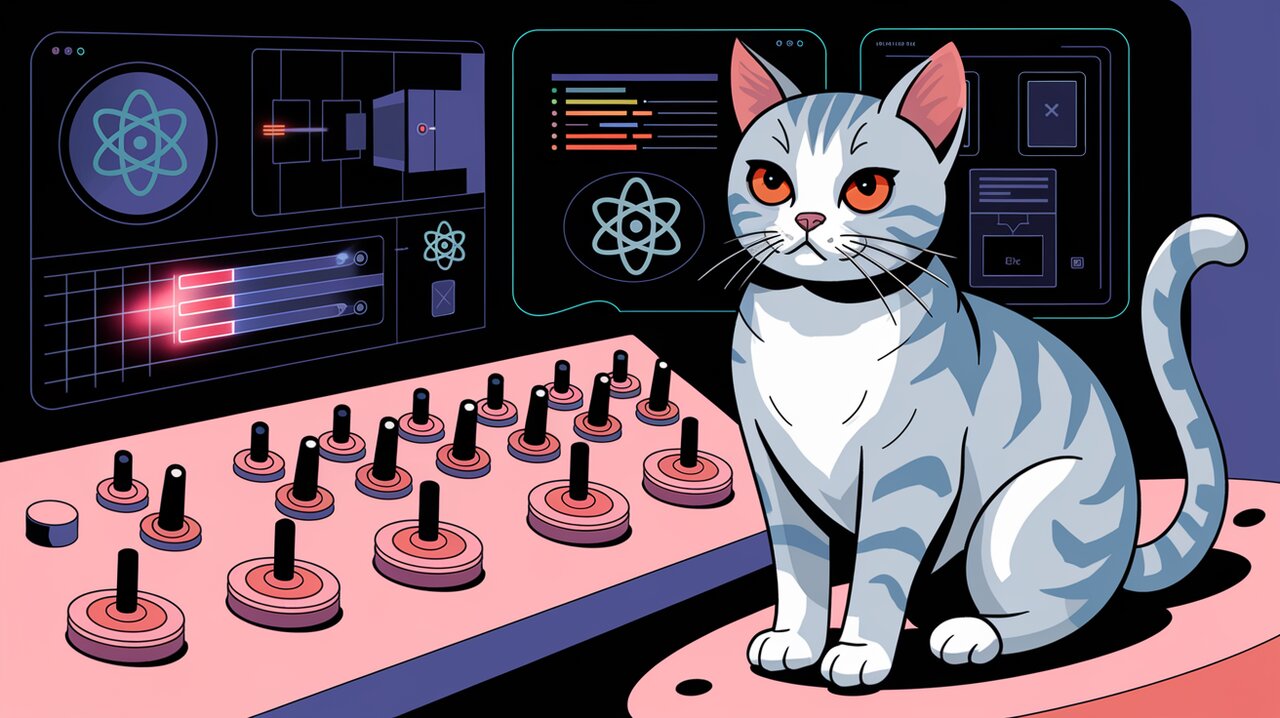
Multireducer Magic: Multiply Your Redux State with Ease
In the realm of React and Redux development, managing complex state structures can often feel like juggling multiple balls while riding a unicycle. Enter multireducer, a magical utility that allows you to effortlessly multiply your Redux reducers, bringing simplicity and scalability to your state management. Let’s embark on a journey to explore this powerful tool and see how it can transform your React applications.
Unraveling the Multireducer Mystery
At its core, multireducer
is a utility that wraps multiple copies of a single Redux reducer into one key-based reducer. This seemingly simple concept opens up a world of possibilities for React developers, especially when dealing with scenarios that require multiple instances of the same type of data or component.
Key Features of Multireducer
- Reducer Reusability: Use the same reducer logic for multiple instances in your state tree.
- Simplified State Structure: Avoid complex nested state objects for similar data types.
- Easy Integration: Seamlessly works with existing Redux setups.
- Performance Optimization: Reduces boilerplate and potentially improves app performance.
Setting Up Your Multireducer Magic Show
Before we dive into the nitty-gritty, let’s get multireducer set up in your project. It’s as simple as waving a wand – or in this case, running a command in your terminal.
npm install multireducer
# or if you prefer yarn
yarn add multireducer
Basic Incantations: Getting Started with Multireducer
Let’s start with a basic example to see multireducer in action. Imagine you’re building an app that needs to manage multiple counters.
The Counter Reducer
First, let’s create a simple counter reducer:
const counterReducer = (state = 0, action: { type: string }) => {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
};
Multiplying Your Reducer
Now, let’s use multireducer
to create multiple instances of this counter:
import { combineReducers } from 'redux';
import multireducer from 'multireducer';
const rootReducer = combineReducers({
counters: multireducer({
counterA: counterReducer,
counterB: counterReducer,
counterC: counterReducer
})
});
In this magical transformation, we’ve created three separate counters, all using the same counterReducer
logic. Each counter will maintain its own state independently.
Advanced Spells: Mastering Multireducer
Now that we’ve got the basics down, let’s explore some more advanced techniques to truly harness the power of multireducer.
Connecting Components to Multireducer State
When connecting your React components to the Redux store, you’ll need to specify which instance of the reducer you’re interested in. Here’s how you might do that:
import { connect } from 'react-redux';
const mapStateToProps = (state, ownProps) => ({
count: state.counters[ownProps.counterId]
});
const mapDispatchToProps = (dispatch, ownProps) => ({
increment: () => dispatch({ type: 'INCREMENT', payload: {}, meta: { key: ownProps.counterId } }),
decrement: () => dispatch({ type: 'DECREMENT', payload: {}, meta: { key: ownProps.counterId } })
});
const ConnectedCounter = connect(mapStateToProps, mapDispatchToProps)(Counter);
Notice the meta: { key: ownProps.counterId }
in the action creators. This is the magic that tells multireducer which instance of the reducer should handle the action.
Dynamic Instance Creation
One of the most powerful features of multireducer is the ability to dynamically create instances of your reducers. This is particularly useful for scenarios where you don’t know in advance how many instances you’ll need.
import { wrapReducer } from 'multireducer';
const dynamicCounterReducer = wrapReducer(counterReducer);
// Later, in your component...
const newCounterId = 'counter' + Date.now();
dispatch(dynamicCounterReducer.createInstance(newCounterId));
This spell allows you to create new counter instances on the fly, perfect for dynamic user interfaces.
Multireducer in the Wild: Real-World Applications
To truly appreciate the power of multireducer, let’s look at some scenarios where it shines:
- Multi-user Dashboards: Manage state for multiple user profiles simultaneously.
- Form Management: Handle multiple instances of the same form type (e.g., multiple address forms).
- Game Development: Manage state for multiple game entities with similar properties.
- E-commerce Platforms: Handle multiple product listings or shopping carts.
The Multireducer Ecosystem
While multireducer is powerful on its own, it plays well with other Redux utilities. For instance, you might want to explore how it interacts with libraries like redux-saga
for managing side effects, or reselect
for optimizing state derivation.
If you’re interested in diving deeper into Redux ecosystem, you might find our articles on Redux Rhapsody: Orchestrating React State and Reselect: Memoization Magic enlightening.
Wrapping Up: The Magic of Multireducer
Multireducer is like a magic wand for Redux state management. It allows you to write cleaner, more modular code by reusing reducer logic across multiple instances. By simplifying your state structure and reducing boilerplate, it paves the way for more scalable and maintainable React applications.
As you continue your journey with multireducer, remember that like any powerful tool, it’s most effective when used judiciously. Consider your application’s needs and architecture to determine where multireducer can bring the most value.
So go forth, wave your multireducer wand, and watch as your Redux state management transforms from a tangled web into a beautifully orchestrated symphony of simplicity and power. Happy coding, and may your states always be manageable!