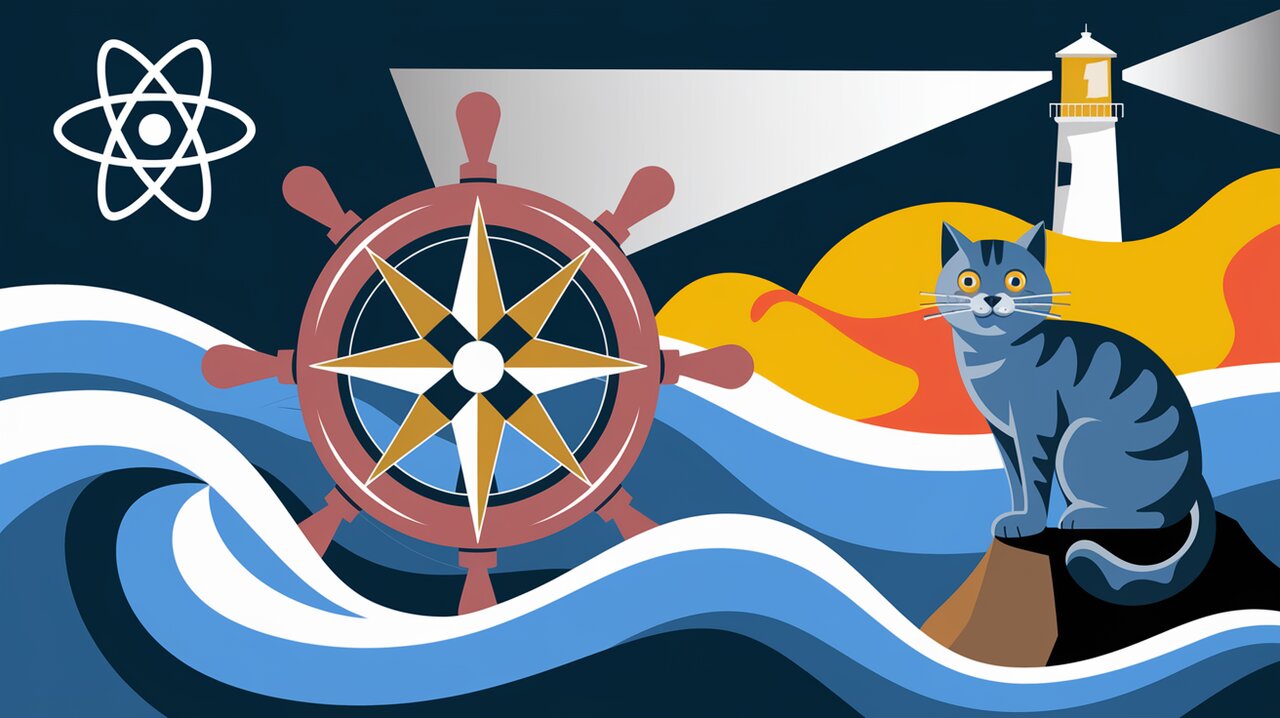
Charting Your Course: Redux-Router5 for Seamless React Navigation
Redux-router5 is a powerful routing solution that combines the strengths of Redux for state management and router5 for flexible routing in React applications. This library provides a seamless way to integrate routing into your Redux-powered React apps, offering a robust and scalable approach to navigation and state handling.
Setting Sail: Getting Started with Redux-Router5
To begin your journey with redux-router5, you’ll need to install the necessary packages:
npm install redux-router5 router5 router5-plugin-browser
Once installed, you can set up your router and integrate it with Redux:
import createRouter from 'router5';
import browserPlugin from 'router5-plugin-browser';
import { reduxPlugin } from 'redux-router5';
import { createStore, combineReducers } from 'redux';
import { router5Reducer } from 'redux-router5';
const routes = [
{ name: 'home', path: '/' },
{ name: 'about', path: '/about' },
{ name: 'contact', path: '/contact' }
];
const router = createRouter(routes);
router.usePlugin(browserPlugin());
router.usePlugin(reduxPlugin());
const store = createStore(combineReducers({
router: router5Reducer
}));
router.start();
This setup creates a router with defined routes, applies the necessary plugins, and integrates it with a Redux store.
Navigating the Waters: Basic Usage
Linking to Routes
To create links in your React components, you can use the Link
component provided by redux-router5:
import React from 'react';
import { Link } from 'redux-router5';
const Navigation = () => (
<nav>
<Link routeName="home">Home</Link>
<Link routeName="about">About</Link>
<Link routeName="contact">Contact</Link>
</nav>
);
The Link
component automatically handles navigation, updating the URL and the Redux store.
Accessing Route Information
You can access the current route information from your Redux store:
import React from 'react';
import { useSelector } from 'react-redux';
const CurrentRoute = () => {
const route = useSelector(state => state.router.route);
return <div>Current route: {route.name}</div>;
};
This component will display the name of the current active route.
Charting Advanced Courses: Advanced Usage
Middleware for Route Changes
Redux-router5 allows you to implement middleware to handle route changes:
import { router5Middleware } from 'redux-router5';
const customMiddleware = router => store => next => action => {
if (action.type === '@@router5/TRANSITION_START') {
console.log('Route transition started');
}
return next(action);
};
const store = createStore(
combineReducers({ router: router5Reducer }),
applyMiddleware(router5Middleware(router), customMiddleware)
);
This middleware logs a message when a route transition starts.
Handling Route Parameters
Router5 supports route parameters, which can be accessed in your components:
const routes = [
{ name: 'user', path: '/user/:id' }
];
// In your component
const UserProfile = () => {
const route = useSelector(state => state.router.route);
return <div>User ID: {route.params.id}</div>;
};
This setup allows you to create dynamic routes with parameters.
Conclusion
Redux-router5 provides a powerful and flexible routing solution for React applications using Redux. By combining the state management capabilities of Redux with the routing prowess of router5, it offers a seamless navigation experience for single-page applications.
As you navigate the seas of React development, redux-router5 serves as a reliable compass, helping you chart a course through complex application states and routes. Whether you’re building a small project or a large-scale application, this library offers the tools you need to create robust and maintainable navigation systems.
For more insights into React routing and state management, you might want to explore our articles on React-Router and Redux. These resources can provide additional context and techniques to enhance your React navigation skills.
Further Reading
For more insights into React routing and state management, you might find these articles helpful:
- Navigating Redux Seas with React Router
- Navigating Redux Waters: Tiny Router
- Navigating the Redux Realm with Universal Redux Router
- Sizzling State Management: Redux Bacon
- Mastering Jotai React State
These resources can provide additional context and techniques to enhance your React navigation and state management skills.