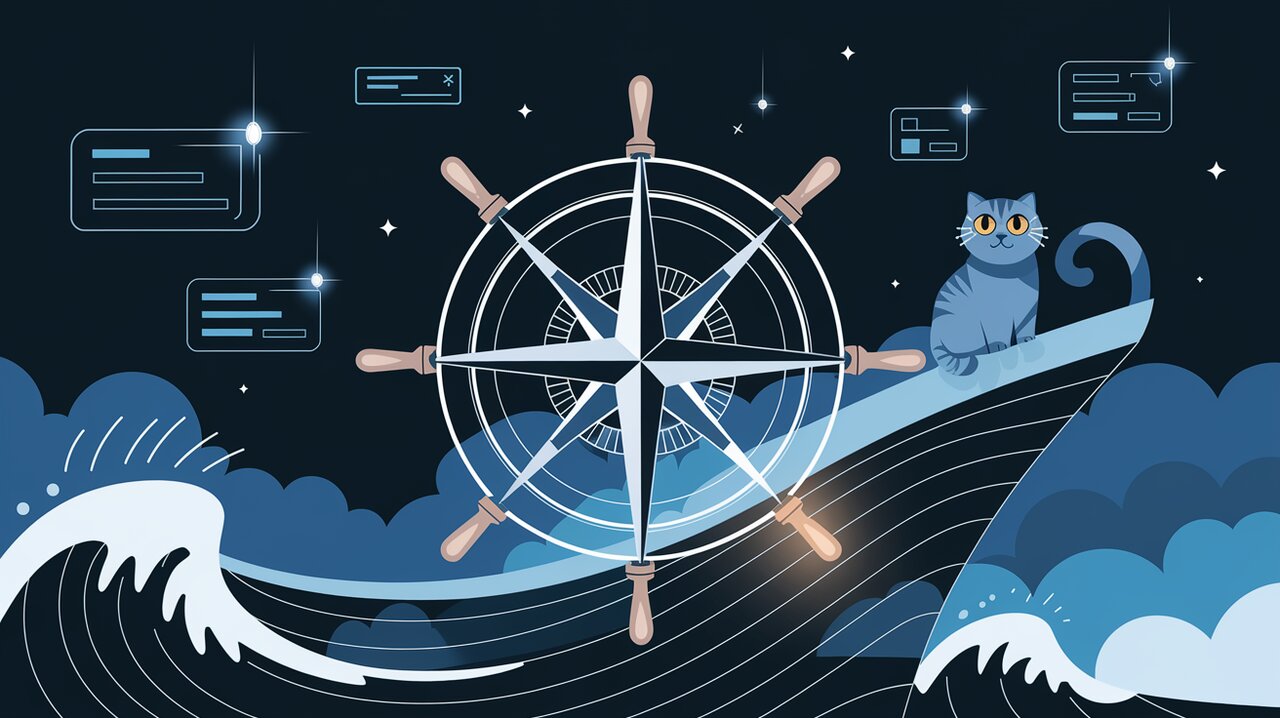
Charting the Course: Navigating Redux Seas with React Router Redux
React Router Redux is a powerful library that bridges the gap between Redux, a predictable state container, and React Router, the standard routing library for React applications. It ensures that your routing information stays in sync with your Redux store, enabling powerful features like time travel debugging while maintaining a single source of truth for your application state.
Setting Sail: Installation and Setup
Before we embark on our journey, let’s equip our ship with the necessary tools. Install react-router-redux along with its peer dependencies:
npm install --save react-router-redux react-router redux
With our supplies on board, let’s configure our application to use react-router-redux:
import React from 'react';
import ReactDOM from 'react-dom';
import { createStore, combineReducers, applyMiddleware } from 'redux';
import { Provider } from 'react-redux';
import { Router, Route, browserHistory } from 'react-router';
import { syncHistoryWithStore, routerReducer } from 'react-router-redux';
// Import your reducers
import * as reducers from './reducers';
// Add the routerReducer to your rootReducer
const rootReducer = combineReducers({
...reducers,
routing: routerReducer
});
// Create the Redux store
const store = createStore(rootReducer);
// Create an enhanced history that syncs navigation events with the store
const history = syncHistoryWithStore(browserHistory, store);
// Render your app
ReactDOM.render(
<Provider store={store}>
<Router history={history}>
<Route path="/" component={App}>
{/* Your routes go here */}
</Route>
</Router>
</Provider>,
document.getElementById('root')
);
This setup integrates react-router-redux into your application, allowing your routing state to be managed by Redux.
Charting the Course: Basic Navigation
With react-router-redux, you can dispatch navigation actions just like any other Redux action. Here’s how you can programmatically navigate in your application:
import { push } from 'react-router-redux';
// In a connected component
const mapDispatchToProps = (dispatch) => ({
navigateToAbout: () => dispatch(push('/about'))
});
// In your component
const MyComponent = ({ navigateToAbout }) => (
<button onClick={navigateToAbout}>Go to About</button>
);
This approach allows you to treat navigation as part of your application’s state, making it predictable and easy to manage.
Navigating the Depths: Advanced Usage
Listening for Navigation Events
react-router-redux allows you to listen for navigation events, which can be useful for analytics or other side effects:
const history = syncHistoryWithStore(browserHistory, store);
history.listen(location => {
// Perform actions based on the new location
console.log('New location:', location);
});
Custom Routing Reducer
If you need more control over how routing state is stored, you can create a custom routing reducer:
import { LOCATION_CHANGE } from 'react-router-redux';
const initialState = {
locationBeforeTransitions: null
};
const routingReducer = (state = initialState, action) => {
if (action.type === LOCATION_CHANGE) {
return { ...state, locationBeforeTransitions: action.payload };
}
return state;
};
Steering Clear of Rough Waters: Common Pitfalls
-
Accessing Router State in Components: Always access router state through props provided by React Router, not directly from the Redux store. This ensures your components stay in sync with asynchronous routing updates.
-
Time Travel and URL Sync: By default, react-router-redux keeps the URL in sync during time travel. If this causes issues, you can disable it:
const history = syncHistoryWithStore(browserHistory, store, {
adjustUrlOnReplay: false
});
- Using with Immutable.js: If you’re using Immutable.js for your Redux state, you’ll need to provide a custom
selectLocationState
function:
const history = syncHistoryWithStore(browserHistory, store, {
selectLocationState: (state) => state.get('routing').toJS()
});
Anchoring Your Knowledge
react-router-redux provides a seamless way to integrate routing with your Redux state management. By keeping your location in sync with your store, you gain the ability to time travel through your application state while maintaining consistent routing.
For more advanced React routing techniques, you might want to explore Wouter for React JS routing, which offers a lightweight alternative to React Router. Additionally, for those interested in type-safe routing, the TanStack Router React Type-Safe Routing Revolution article provides insights into a modern, type-safe routing solution.
As you continue to navigate the vast seas of React development, remember that react-router-redux acts as your compass, ensuring that your application’s state and routing remain in perfect harmony. Happy sailing!