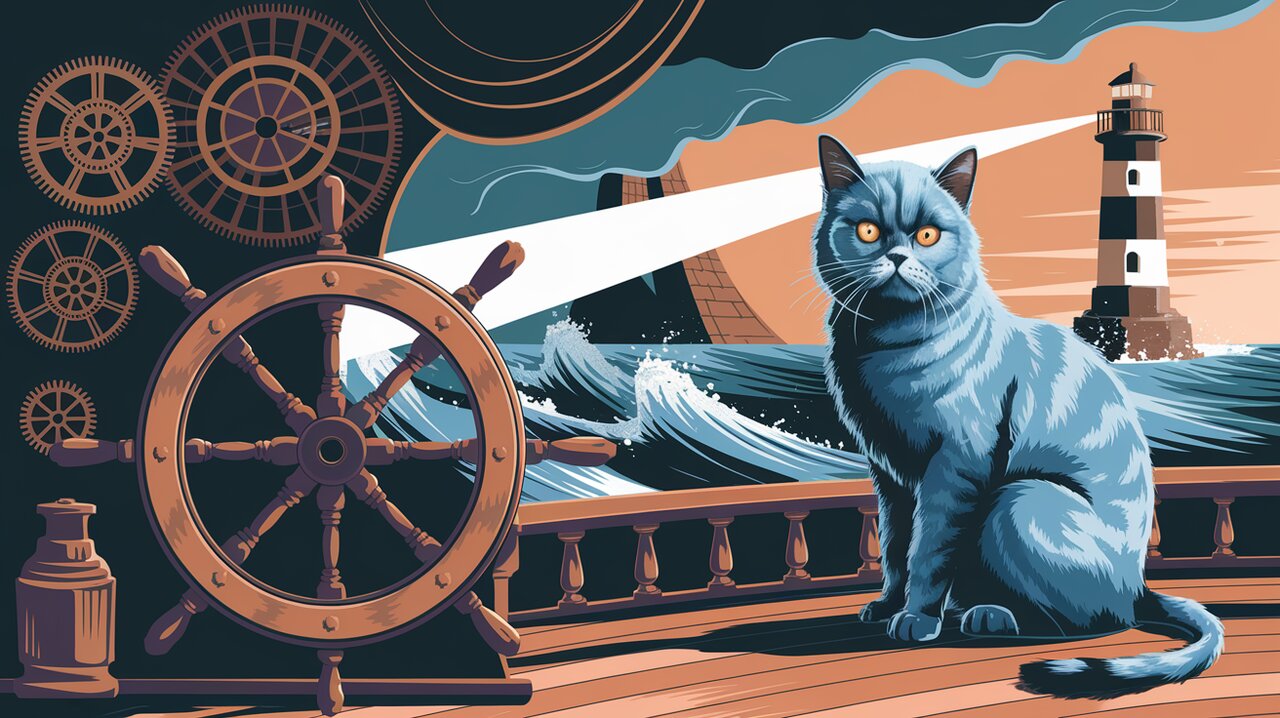
In the vast ocean of React development, synchronizing your application’s URL with its state can feel like navigating treacherous waters. Enter Redux VStack Router, a lighthouse guiding developers to smoother sailing. This powerful library seamlessly integrates routing with Redux state management, allowing you to chart a clear course through your application’s structure.
Anchoring Your Project
Before we set sail, let’s get Redux VStack Router on board. You can add it to your project using npm or yarn:
npm install redux-vstack-router
Or if you prefer yarn:
yarn add redux-vstack-router
Charting Your Course
Now that we’ve got our navigation tools, let’s plot our route. Redux VStack Router provides a simple API to connect your Redux store with your application’s routing.
Setting Up the Navigation
First, we need to create our router and connect it to our Redux store:
import { createStore, applyMiddleware } from 'redux'
import { createRouter, routerMiddleware } from 'redux-vstack-router'
const router = createRouter({
'/': { component: Home },
'/about': { component: About },
'/users/:id': { component: User }
})
const store = createStore(
rootReducer,
applyMiddleware(routerMiddleware(router))
)
In this example, we’re creating a router with three routes and applying the routerMiddleware
to our Redux store. This middleware is the key to keeping our URL and state in sync.
Navigating the Waters
With our router set up, we can now navigate through our application using Redux actions:
import { push } from 'redux-vstack-router'
// In a component or action creator
dispatch(push('/about'))
This action will update both the URL and the Redux state, ensuring they remain synchronized.
Advanced Navigation Techniques
Redux VStack Router isn’t just for simple navigation. It provides advanced features for more complex routing scenarios.
Nested Routes
For larger applications, nested routes can help organize your navigation structure:
const router = createRouter({
'/': { component: Home },
'/users': {
component: Users,
routes: {
'/:id': { component: User },
'/new': { component: NewUser }
}
}
})
This setup allows for URLs like /users/123
or /users/new
, with each level of nesting corresponding to a component in your UI hierarchy.
Route Parameters
Redux VStack Router makes it easy to access route parameters in your components:
import { useRouteParams } from 'redux-vstack-router'
function User() {
const { id } = useRouteParams()
return <div>User ID: {id}</div>
}
This hook provides access to any parameters defined in your route, making it simple to fetch and display dynamic data.
Programmatic Navigation
For more complex navigation scenarios, Redux VStack Router provides a suite of actions:
import { push, replace, go, goBack, goForward } from 'redux-vstack-router'
// Navigate to a new URL
dispatch(push('/users/123'))
// Replace the current URL without adding to history
dispatch(replace('/not-found'))
// Go back or forward in history
dispatch(goBack())
dispatch(goForward())
// Go to a specific point in history
dispatch(go(-2))
These actions give you fine-grained control over your application’s navigation, allowing for complex user flows and interactions.
Staying on Course
Redux VStack Router doesn’t just help with navigation; it also keeps your application state in sync with the URL. The router state is stored in your Redux store, allowing you to access routing information anywhere in your application:
import { useSelector } from 'react-redux'
function NavigationInfo() {
const { pathname, search, hash } = useSelector(state => state.router)
return <div>Current URL: {pathname}{search}{hash}</div>
}
This tight integration between routing and state management ensures that your application always knows where it stands, preventing the all-too-common issues of desynchronized UI and URL states.
Conclusion
Navigating the complex waters of state management and routing in React applications can be daunting, but Redux VStack Router provides a sturdy vessel for your journey. By seamlessly integrating routing with Redux, it allows developers to focus on building great user experiences without worrying about the underlying navigation mechanics.
Whether you’re building a simple website or a complex web application, Redux VStack Router offers the tools you need to keep your application’s state and URL in perfect harmony. So hoist the sails, set your course, and let Redux VStack Router guide you through the seas of web development!