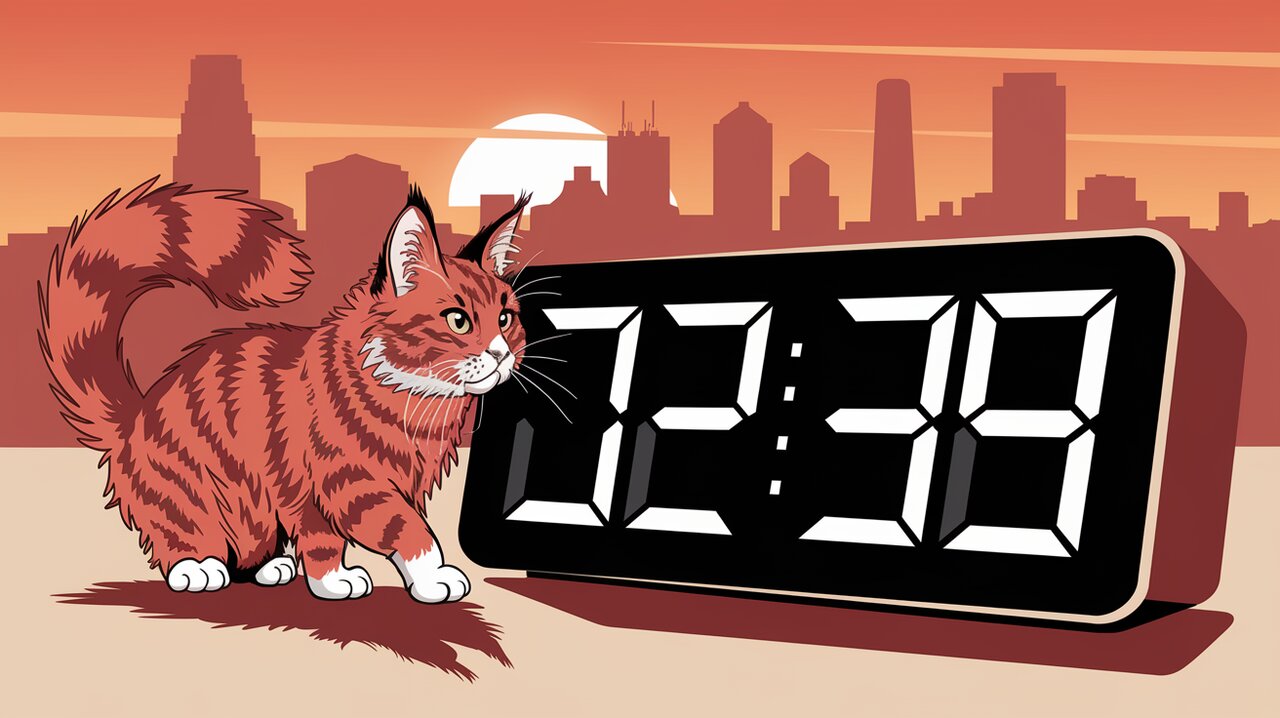
Counting Down in Style: React Transitive Number's Numeric Wizardry
In the realm of React development, creating visually appealing and interactive user interfaces often involves incorporating subtle yet impactful animations. When it comes to displaying changing numeric values, such as countdowns, timers, or dynamic statistics, a smooth transition between numbers can significantly enhance the user experience. This is where React Transitive Number comes into play, offering a simple yet powerful solution for animating numeric strings in your React applications.
Unveiling React Transitive Number
React Transitive Number is a specialized component designed to apply transition effects to numeric strings. Inspired by the eye-catching timers once used on Groupon, this library allows developers to easily implement smooth, animated transitions between different numeric values. Whether you’re building a countdown timer, a real-time statistics display, or any interface element that involves changing numbers, React Transitive Number can add that extra touch of polish to your application.
Key Features
- Smooth Transitions: Seamlessly animate changes in numeric values.
- Flexible Input: Accepts any numeric string, including those with colons (e.g., time formats).
- Customizable: Offers options to control initial animation and styling.
- React-Friendly: Designed specifically for use in React applications.
Getting Started with React Transitive Number
Installation
To start using React Transitive Number in your project, you’ll need to install it via npm or yarn. Open your terminal and run one of the following commands:
Using npm:
npm install react-transitive-number
Using yarn:
yarn add react-transitive-number
Basic Implementation
Once installed, you can start using React Transitive Number in your React components. Here’s a simple example to get you started:
import React from 'react';
import TransitiveNumber from 'react-transitive-number';
const CountdownDisplay: React.FC = () => {
return (
<div>
<TransitiveNumber>2:00</TransitiveNumber>
</div>
);
};
export default CountdownDisplay;
In this basic example, we’re rendering a TransitiveNumber
component with the initial value of “2:00”. As this value changes (for instance, in a countdown scenario), React Transitive Number will automatically apply smooth transitions between the changing digits.
Diving Deeper: Advanced Usage
Enabling Initial Animation
By default, React Transitive Number doesn’t animate when the component is first mounted. However, you can enable this initial animation by setting the enableInitialAnimation
prop:
import React from 'react';
import TransitiveNumber from 'react-transitive-number';
const AnimatedCounter: React.FC = () => {
return (
<TransitiveNumber enableInitialAnimation={true}>
1000
</TransitiveNumber>
);
};
This setup will cause the number to animate into view when the component first renders, providing an eye-catching entrance effect.
Styling Your Transitive Number
React Transitive Number allows for easy styling through the use of CSS classes. You can pass a custom class name to the component using the className
prop:
import React from 'react';
import TransitiveNumber from 'react-transitive-number';
import './CustomStyles.css';
const StyledCounter: React.FC = () => {
return (
<TransitiveNumber className="fancy-number">
5000
</TransitiveNumber>
);
};
In your CSS file (CustomStyles.css
in this example), you can then define styles for the .fancy-number
class to customize the appearance of your transitive number display.
Dynamic Value Updates
One of the most powerful features of React Transitive Number is its ability to smoothly transition between changing values. Here’s an example of how you might use it in a component with changing state:
import React, { useState, useEffect } from 'react';
import TransitiveNumber from 'react-transitive-number';
const DynamicCounter: React.FC = () => {
const [count, setCount] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setCount(prevCount => prevCount + 1);
}, 1000);
return () => clearInterval(interval);
}, []);
return (
<div>
<h2>Dynamic Counter:</h2>
<TransitiveNumber>{count}</TransitiveNumber>
</div>
);
};
In this example, we’ve created a counter that increments every second. React Transitive Number will smoothly animate the transition between each number, creating a fluid and engaging visual effect.
Wrapping Up
React Transitive Number offers a straightforward yet powerful way to add engaging numeric transitions to your React applications. Whether you’re building a countdown timer, a live statistics dashboard, or any interface that involves changing numbers, this library can help you create smooth, eye-catching animations with minimal effort.
By leveraging the features of React Transitive Number, developers can significantly enhance the visual appeal and interactivity of their applications. The library’s simplicity in implementation, combined with its flexibility in handling various numeric formats, makes it an excellent choice for projects ranging from simple counters to complex data visualization tools.
As you continue to explore the capabilities of React Transitive Number, you’ll likely discover even more creative ways to incorporate smooth numeric transitions into your React projects, elevating the overall user experience and adding that extra layer of polish to your applications.