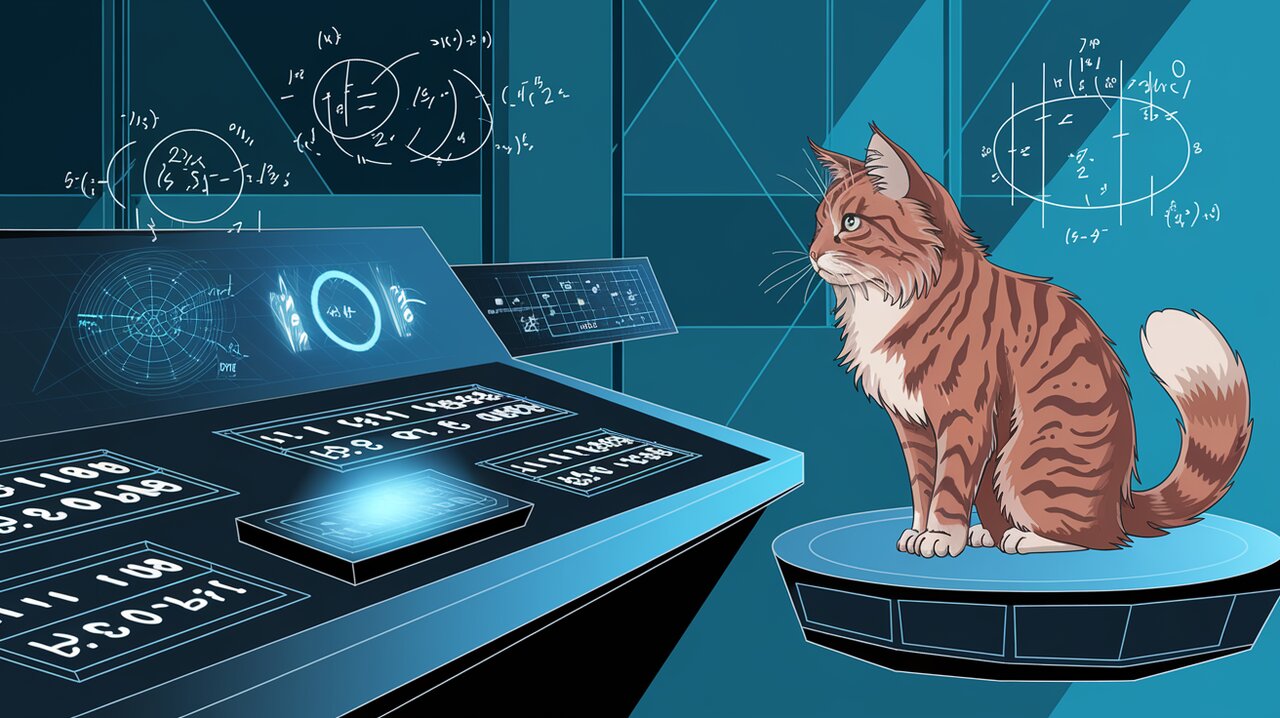
Numeric Wizardry: Unleashing the Power of react-numeric-input
react-numeric-input
is a powerful React component that enhances the functionality of number input fields in web applications. It addresses the limitations of native number inputs by providing consistent appearance across browsers and offering a wide range of customization options. Whether you’re building a simple form or a complex data entry interface, this library can significantly improve the user experience when dealing with numeric values.
Features
- Cross-browser compatibility with consistent styling
- Customizable min and max values
- Step increments for precise value control
- Floating-point number support
- Custom formatting and parsing
- Keyboard navigation
- Mobile-friendly interface
- Strict and loose input modes
Installation
To get started with react-numeric-input
, you can install it using npm or yarn:
npm install react-numeric-input --save
or
yarn add react-numeric-input
After installation, you can import the component in your React application:
import NumericInput from 'react-numeric-input';
Basic Usage
Simple Number Input
The most basic usage of react-numeric-input
is similar to a standard HTML number input:
import React from 'react';
import NumericInput from 'react-numeric-input';
const SimpleNumberInput: React.FC = () => {
return <NumericInput />;
};
This will create an empty numeric input that starts changing from zero. The component ensures consistent appearance across different browsers.
Setting Min, Max, and Default Value
In most cases, you’ll want to specify minimum and maximum values, as well as a default value:
import React from 'react';
import NumericInput from 'react-numeric-input';
const BoundedNumberInput: React.FC = () => {
return <NumericInput min={0} max={100} value={50} />;
};
This example creates a numeric input with a range from 0 to 100 and a default value of 50.
Advanced Usage
Working with Floating-Point Numbers
To handle decimal numbers, you can use the step
and precision
props:
import React from 'react';
import NumericInput from 'react-numeric-input';
const FloatingPointInput: React.FC = () => {
return <NumericInput step={0.1} precision={2} value={0} />;
};
This input will allow increments of 0.1 and display numbers with two decimal places.
Custom Formatting
You can customize how the value is displayed using the format
prop:
import React from 'react';
import NumericInput from 'react-numeric-input';
const CurrencyInput: React.FC = () => {
const formatCurrency = (num: number) => `$${num.toFixed(2)}`;
return <NumericInput format={formatCurrency} />;
};
This example formats the input value as a currency with a dollar sign and two decimal places.
Snap to Step
The snap
prop allows the input to automatically adjust to the nearest step value:
import React from 'react';
import NumericInput from 'react-numeric-input';
const SnappingInput: React.FC = () => {
return <NumericInput step={5} snap value={0} />;
};
With this configuration, the input will snap to the nearest multiple of 5 when incrementing or decrementing.
Mobile-Friendly Configuration
react-numeric-input
can adapt to mobile devices:
import React from 'react';
import NumericInput from 'react-numeric-input';
const MobileInput: React.FC = () => {
return <NumericInput mobile />;
};
This ensures a touch-friendly interface on mobile devices.
Dynamic Props
Some props can be functions, allowing dynamic behavior:
import React from 'react';
import NumericInput from 'react-numeric-input';
const DynamicStepInput: React.FC = () => {
const dynamicStep = (component: any) => {
return component.state.value < 10 ? 0.1 : 1;
};
return <NumericInput step={dynamicStep} />;
};
In this example, the step size changes based on the current value.
Styling
react-numeric-input
uses inline styles by default, which can be customized:
import React from 'react';
import NumericInput from 'react-numeric-input';
const StyledInput: React.FC = () => {
return (
<NumericInput
style={{
input: {
color: 'red',
fontSize: '18px',
},
'input:focus': {
border: '2px solid blue',
},
}}
/>
);
};
You can also use CSS by targeting the .react-numeric-input
class:
.react-numeric-input input {
font-weight: bold;
}
Keyboard Navigation
react-numeric-input
supports keyboard navigation for a better user experience:
- Up/Down arrows: Increment/decrement by
step
- Ctrl/Cmd + Up/Down: Smaller increment/decrement (
step / 10
) - Shift + Up/Down: Larger increment/decrement (
step * 10
)
Conclusion
react-numeric-input
is a versatile and powerful component that brings enhanced functionality to numeric inputs in React applications. Its wide range of features, from custom formatting to keyboard navigation, makes it an excellent choice for developers looking to create user-friendly and robust number input interfaces. By leveraging this library, you can ensure a consistent experience across browsers while providing advanced capabilities that go beyond the native HTML number input.
Whether you’re building a simple form or a complex data entry system, react-numeric-input
offers the flexibility and power to meet your needs. Its ease of use, combined with extensive customization options, makes it a valuable tool in any React developer’s toolkit.