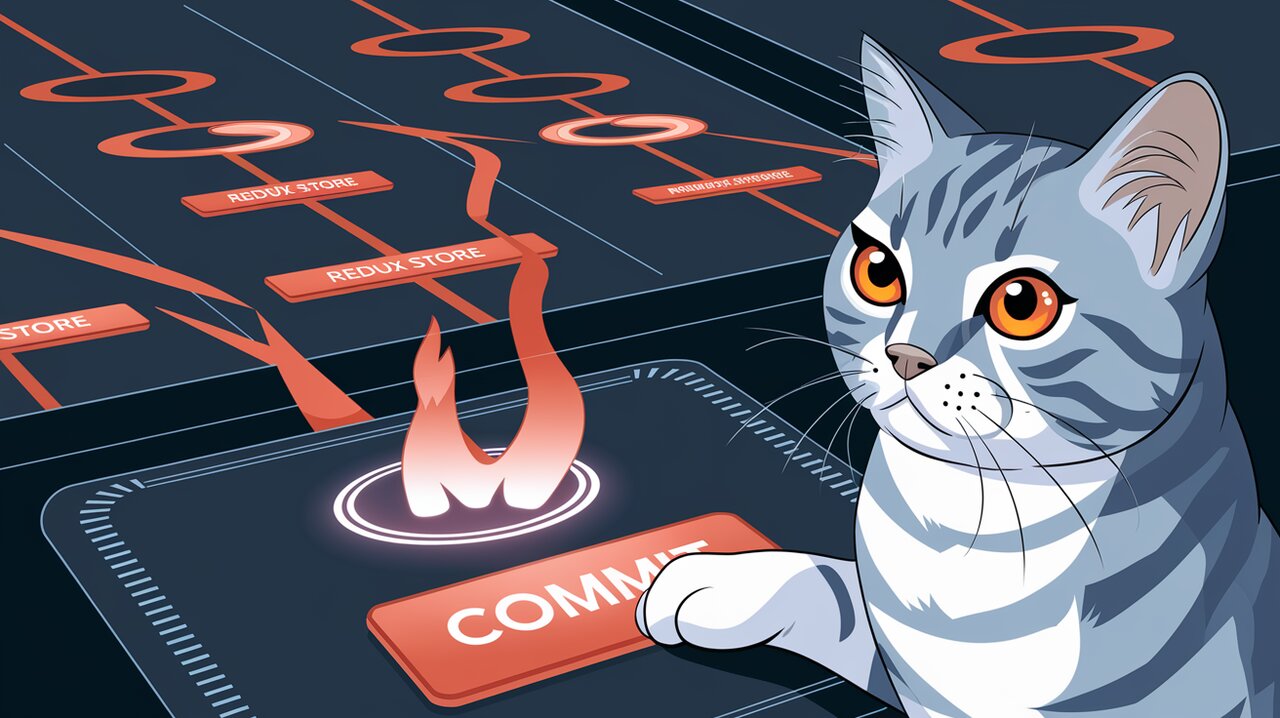
Redux Optimist: Time-Traveling Optimistic Updates for React Apps
Redux Optimist is a powerful library that brings the concept of optimistic updates to React applications using Redux for state management. By allowing developers to apply actions optimistically before they are confirmed, this library significantly enhances the user experience, making applications feel more responsive and snappy.
What are Optimistic Updates?
Optimistic updates are a technique used in user interface design where the app updates its state immediately in response to a user action, without waiting for confirmation from the server. This creates a perception of instant responsiveness, even when dealing with operations that may take some time to complete.
For example, when a user likes a post on a social media app, the like count increases immediately, even before the server confirms the action. If the server request fails, the app can then revert the change, ensuring data consistency.
Getting Started with Redux Optimist
To begin using redux-optimist in your React application, you first need to install it:
npm install redux-optimist
or if you’re using Yarn:
yarn add redux-optimist
Basic Usage
The core concept of redux-optimist revolves around wrapping your root reducer and marking certain actions as optimistic. Let’s walk through the basic setup and usage.
Wrapping the Root Reducer
First, you need to wrap your root reducer with the optimist
higher-order reducer:
import optimist from 'redux-optimist';
import { combineReducers } from 'redux';
import todos from './todos';
import status from './status';
const rootReducer = optimist(combineReducers({
todos,
status
}));
export default rootReducer;
This setup allows redux-optimist to intercept and handle optimistic actions.
Marking Actions as Optimistic
To make an action optimistic, you need to add an optimist
property to the action object. This property should contain a type
(either BEGIN
, COMMIT
, or REVERT
) and a unique id
:
import { BEGIN, COMMIT, REVERT } from 'redux-optimist';
// Optimistic action
const addTodoOptimistic = (text) => ({
type: 'ADD_TODO',
text,
optimist: { type: BEGIN, id: 'add-todo-1' }
});
// Commit action
const addTodoSuccess = (text, response) => ({
type: 'ADD_TODO_SUCCESS',
text,
response,
optimist: { type: COMMIT, id: 'add-todo-1' }
});
// Revert action
const addTodoFailure = (text, error) => ({
type: 'ADD_TODO_FAILURE',
text,
error,
optimist: { type: REVERT, id: 'add-todo-1' }
});
Advanced Usage
Handling Asynchronous Operations
In real-world applications, optimistic updates often involve asynchronous operations. Let’s look at how we can use redux-optimist with a middleware like Redux Thunk to handle asynchronous actions:
import { BEGIN, COMMIT, REVERT } from 'redux-optimist';
import api from './api';
let nextTransactionID = 0;
export const addTodo = (text) => (dispatch) => {
const transactionID = nextTransactionID++;
// Dispatch optimistic update
dispatch({
type: 'ADD_TODO',
text,
optimist: { type: BEGIN, id: transactionID }
});
// Perform API call
api.addTodo(text)
.then(response => {
// If successful, commit the optimistic update
dispatch({
type: 'ADD_TODO_SUCCESS',
text,
response,
optimist: { type: COMMIT, id: transactionID }
});
})
.catch(error => {
// If failed, revert the optimistic update
dispatch({
type: 'ADD_TODO_FAILURE',
text,
error,
optimist: { type: REVERT, id: transactionID }
});
});
};
This pattern allows you to update the UI immediately while handling the asynchronous operation in the background. If the operation fails, the optimistic update is automatically reverted.
Managing Complex State
When dealing with more complex state structures, you might need to handle multiple optimistic updates simultaneously. redux-optimist allows you to manage this by using unique transaction IDs for each optimistic action:
const updateUserProfile = (userId, updates) => (dispatch) => {
const transactionID = `update-user-${userId}-${Date.now()}`;
dispatch({
type: 'UPDATE_USER_PROFILE',
userId,
updates,
optimist: { type: BEGIN, id: transactionID }
});
api.updateUserProfile(userId, updates)
.then(response => {
dispatch({
type: 'UPDATE_USER_PROFILE_SUCCESS',
userId,
updates,
response,
optimist: { type: COMMIT, id: transactionID }
});
})
.catch(error => {
dispatch({
type: 'UPDATE_USER_PROFILE_FAILURE',
userId,
updates,
error,
optimist: { type: REVERT, id: transactionID }
});
});
};
By using unique transaction IDs, you can ensure that each optimistic update is handled independently, allowing for multiple concurrent optimistic updates in your application.
Best Practices
When working with redux-optimist, keep these best practices in mind:
- Always use unique transaction IDs for each optimistic action.
- Ensure that every
BEGIN
action is followed by either aCOMMIT
orREVERT
action to prevent memory leaks. - Design your reducers to handle both optimistic and non-optimistic versions of actions.
- Use meaningful action types for optimistic, success, and failure cases to make debugging easier.
Conclusion
Redux Optimist is a powerful tool for enhancing the user experience in React applications. By enabling optimistic updates, it allows developers to create responsive interfaces that provide instant feedback while handling asynchronous operations in the background.
As you integrate redux-optimist into your projects, you’ll find that it seamlessly fits into the Redux ecosystem, working well with other middleware and enhancing your application’s overall performance and user satisfaction.
For more insights into Redux and state management in React, check out our articles on Redux Logger: Detective Tracking State Changes and Redux Persist: Store State Preservation Symphony. These complementary tools can further enhance your Redux workflow and debugging capabilities.
By mastering redux-optimist, you’re taking a significant step towards creating more responsive and user-friendly React applications. Happy coding!