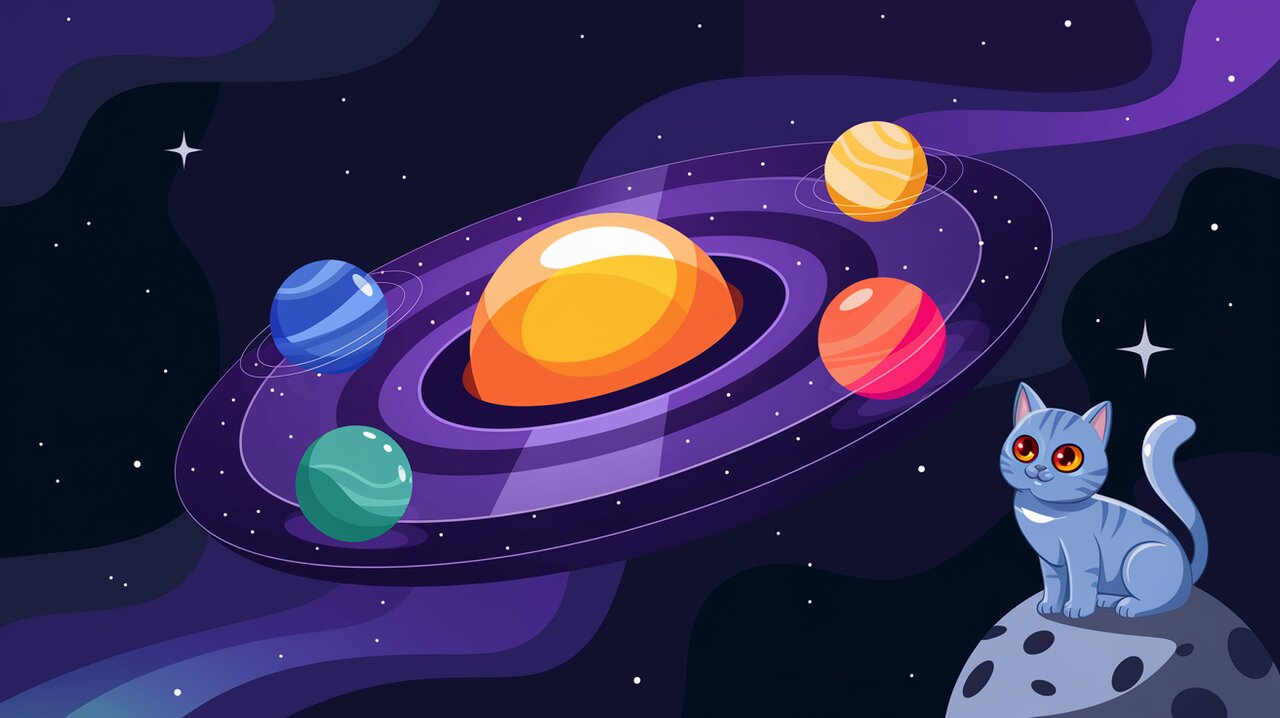
Orbit Your UI: Crafting Stellar Circular Menus with React Planet
In the vast universe of React components, React Planet shines as a brilliant star, offering developers an elegant solution for creating circular menus. This innovative library empowers you to craft interactive, orbit-like UI elements that can elevate your application’s user experience to celestial heights. Whether you’re building a space-themed app or simply want to add a touch of cosmic flair to your interface, React Planet provides the gravitational pull your project needs.
Stellar Features
React Planet boasts an array of features that make it a standout choice for developers:
- Customizable Orbits: Adjust the size and spacing of your circular menu with ease.
- Flexible Styling: Apply your own CSS to create unique looks for each orbiting element.
- Smooth Animations: Built-in transitions for a polished, professional feel.
- Responsive Design: Adapts seamlessly to different screen sizes and devices.
- TypeScript Support: Enjoy full type safety and improved developer experience.
Launching React Planet
Before we embark on our cosmic journey, let’s install React Planet in your project. You can use either npm or yarn:
npm install --save react-planet
Or if you prefer yarn:
yarn add react-planet
Basic Flight Path
Let’s start with a simple example to get our circular menu off the ground. First, we’ll import the Planet
component and create a basic structure:
import React from 'react';
import { Planet } from 'react-planet';
const MyPlanet: React.FC = () => {
return (
<Planet
centerContent={
<div
style={{
height: 100,
width: 100,
borderRadius: '50%',
backgroundColor: '#ffa500',
}}
/>
}
hideOrbit
>
<div style={{ backgroundColor: '#ff0000' }}>Mars</div>
<div style={{ backgroundColor: '#0000ff' }}>Earth</div>
<div style={{ backgroundColor: '#008000' }}>Venus</div>
</Planet>
);
};
export default MyPlanet;
In this example, we’ve created a central orange “sun” with three orbiting planets. The hideOrbit
prop ensures that our planetary system appears clean and minimalist.
Navigating the Cosmos
Now that we’ve launched our basic planet system, let’s explore some more advanced features of React Planet. We’ll create a more interactive and visually appealing menu:
Customizing Orbits
You can adjust the orbit size and spacing to create the perfect layout for your menu:
import React from 'react';
import { Planet } from 'react-planet';
const AdvancedPlanet: React.FC = () => {
return (
<Planet
centerContent={
<button
style={{
height: 80,
width: 80,
borderRadius: '50%',
backgroundColor: '#24292e',
color: '#fff',
border: 'none',
cursor: 'pointer',
}}
>
Menu
</button>
}
orbitRadius={120}
bounceOnClose
bounceOnOpen
rotation={105}
orbitStyle={() => ({
border: '1px solid #24292e',
})}
>
{['Profile', 'Settings', 'Logout'].map((item, index) => (
<div
key={index}
style={{
height: 60,
width: 60,
borderRadius: '50%',
backgroundColor: `hsl(${index * 60}, 70%, 60%)`,
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
color: '#fff',
}}
>
{item}
</div>
))}
</Planet>
);
};
export default AdvancedPlanet;
This advanced example showcases several key features:
orbitRadius
: Sets the distance of orbiting elements from the center.bounceOnClose
andbounceOnOpen
: Adds a playful bounce animation when opening or closing the menu.rotation
: Adjusts the starting angle of the first orbiting element.orbitStyle
: Allows custom styling of the orbit itself.
Implementing Interactivity
Let’s add some interactivity to our cosmic menu:
import React, { useState } from 'react';
import { Planet } from 'react-planet';
const InteractivePlanet: React.FC = () => {
const [open, setOpen] = useState(false);
const handleToggle = () => setOpen(!open);
return (
<Planet
centerContent={
<button
onClick={handleToggle}
style={{
height: 100,
width: 100,
borderRadius: '50%',
backgroundColor: open ? '#4CAF50' : '#2196F3',
color: '#fff',
border: 'none',
cursor: 'pointer',
transition: 'background-color 0.3s',
}}
>
{open ? 'Close' : 'Open'}
</button>
}
open={open}
orbitRadius={120}
autoClose
orbitStyle={{
borderWidth: 2,
borderStyle: 'dashed',
borderColor: '#E91E63',
}}
>
{['Home', 'About', 'Contact', 'Blog'].map((item, index) => (
<button
key={index}
onClick={() => console.log(`Navigating to ${item}`)}
style={{
height: 80,
width: 80,
borderRadius: '50%',
backgroundColor: `hsl(${index * 90}, 70%, 60%)`,
color: '#fff',
border: 'none',
cursor: 'pointer',
}}
>
{item}
</button>
))}
</Planet>
);
};
export default InteractivePlanet;
In this interactive example, we’ve introduced:
- State management to control the open/closed status of the menu.
- A toggle button in the center that changes color based on the menu state.
- The
autoClose
prop, which automatically closes the menu when clicking outside. - Clickable orbiting elements that log navigation actions to the console.
Cosmic Conclusion
React Planet offers a universe of possibilities for creating engaging and interactive circular menus in your React applications. From simple orbits to complex, interactive systems, this library provides the tools you need to craft user interfaces that are truly out of this world.
By leveraging React Planet’s customizable features, smooth animations, and responsive design, you can create UI elements that not only look stellar but also provide an intuitive and enjoyable user experience. Whether you’re building a space-themed application or simply want to add a touch of celestial charm to your interface, React Planet is your ticket to the stars.
So, why settle for static, linear menus when you can have your UI elements dance in perfect orbits? Embrace the cosmic power of React Planet and watch your user interfaces transform into captivating celestial bodies that users will love to explore. The universe of engaging UI design is at your fingertips – it’s time to launch your creativity into orbit!