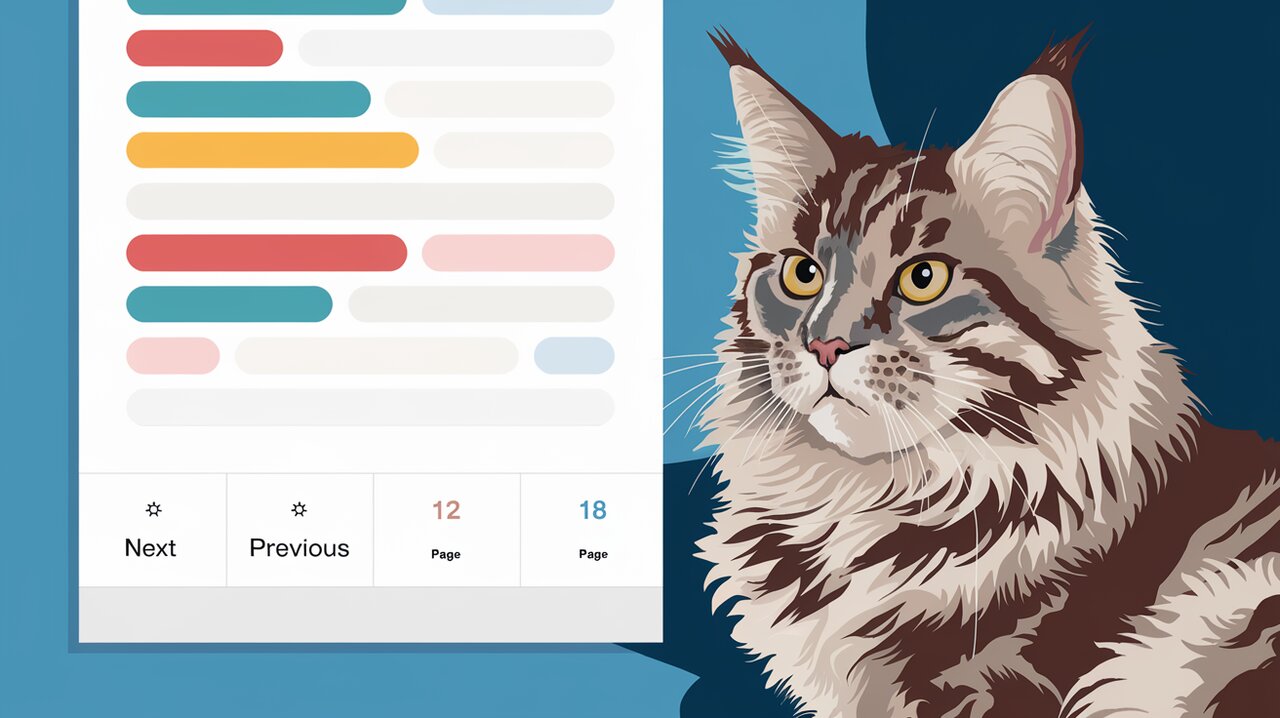
Paginate Like a Pro: Mastering React-Paginate for Seamless Data Navigation
React-paginate is a powerful and flexible pagination component for React applications. It allows developers to easily implement pagination functionality, enabling users to navigate through large sets of data efficiently. Whether you’re building a blog, an e-commerce platform, or any application that deals with extensive data, react-paginate provides a seamless solution for breaking content into manageable pages.
Features
React-paginate comes packed with several features that make it a go-to choice for pagination in React applications:
- Customizable page range
- Support for previous and next buttons
- Ability to handle both client-side and server-side pagination
- Keyboard navigation support
- Responsive design
- Customizable styling
- Accessibility features
Installation
To get started with react-paginate
, you need to install it in your React project. You can do this using either npm or yarn.
Using npm:
npm install react-paginate --save
Using yarn:
yarn add react-paginate
Basic Usage
Let’s dive into the basic usage of react-paginate
with a simple example.
Setting Up the Component
First, import the necessary dependencies and set up a basic component structure:
import React, { useState } from 'react';
import ReactPaginate from 'react-paginate';
const PaginatedItems = ({ itemsPerPage }) => {
// Component logic will go here
};
In this setup, we’re creating a PaginatedItems
component that will accept itemsPerPage
as a prop to determine how many items should be displayed on each page.
Implementing Pagination Logic
Next, let’s implement the pagination logic:
const PaginatedItems = ({ itemsPerPage }) => {
const [itemOffset, setItemOffset] = useState(0);
const items = [/* Your array of items */];
const endOffset = itemOffset + itemsPerPage;
const currentItems = items.slice(itemOffset, endOffset);
const pageCount = Math.ceil(items.length / itemsPerPage);
const handlePageClick = (event: { selected: number }) => {
const newOffset = (event.selected * itemsPerPage) % items.length;
setItemOffset(newOffset);
};
return (
<>
{/* Render your items here */}
<ReactPaginate
breakLabel="..."
nextLabel="next >"
onPageChange={handlePageClick}
pageRangeDisplayed={5}
pageCount={pageCount}
previousLabel="< previous"
renderOnZeroPageCount={null}
/>
</>
);
};
This code sets up the basic structure for pagination. It calculates the current items to display based on the itemOffset
and itemsPerPage
, determines the total number of pages, and handles page changes.
Rendering Items
To complete the basic usage, let’s add a function to render the current items:
const PaginatedItems = ({ itemsPerPage }) => {
// ... previous code
const renderItems = () => {
return currentItems.map((item, index) => (
<div key={index}>
{/* Render your item content here */}
{item}
</div>
));
};
return (
<>
{renderItems()}
<ReactPaginate
// ... pagination props
/>
</>
);
};
This renderItems
function maps over the currentItems
array and renders each item. You can customize this function to match your specific item structure and styling needs.
Advanced Usage
React-paginate offers several advanced features for more complex pagination scenarios. Let’s explore some of these capabilities.
Custom Page Range
You can customize the number of pages displayed in the pagination control:
<ReactPaginate
// ... other props
pageRangeDisplayed={3}
marginPagesDisplayed={2}
/>
This configuration will display 3 pages in the middle range and 2 pages on each margin.
Styling and Customization
React-paginate allows for extensive styling customization. You can pass className props to style different parts of the pagination component:
<ReactPaginate
// ... other props
containerClassName="pagination"
pageClassName="page-item"
pageLinkClassName="page-link"
activeClassName="active"
previousClassName="page-item"
nextClassName="page-item"
previousLinkClassName="page-link"
nextLinkClassName="page-link"
disabledClassName="disabled"
/>
These class names can be targeted in your CSS to achieve the desired look and feel.
Server-Side Pagination
For large datasets, server-side pagination is often necessary. Here’s how you can implement it with react-paginate
:
const ServerSidePagination = () => {
const [pageCount, setPageCount] = useState(0);
const [items, setItems] = useState([]);
const fetchItems = async (currentPage: number) => {
const res = await fetch(`/api/items?page=${currentPage}&limit=10`);
const data = await res.json();
setItems(data.items);
setPageCount(data.totalPages);
};
const handlePageClick = (event: { selected: number }) => {
fetchItems(event.selected);
};
useEffect(() => {
fetchItems(0);
}, []);
return (
<>
{/* Render items */}
<ReactPaginate
pageCount={pageCount}
onPageChange={handlePageClick}
// ... other props
/>
</>
);
};
This example demonstrates how to fetch data from an API based on the current page and update the component state accordingly.
Keyboard Navigation
React-paginate supports keyboard navigation out of the box. Users can use the Tab key to focus on pagination controls and the Enter key to select a page. You can enhance this feature by adding custom key handlers:
<ReactPaginate
// ... other props
onPageActive={(selectedItem) => {
console.log(`Page ${selectedItem.selected + 1} is now active`);
}}
/>
This callback allows you to perform additional actions when a page becomes active through keyboard navigation.
Conclusion
React-paginate is a versatile and powerful tool for implementing pagination in React applications. Its ease of use, customization options, and support for both client-side and server-side pagination make it an excellent choice for developers looking to enhance their data navigation interfaces.
By leveraging react-paginate
, you can create more user-friendly and efficient applications that handle large datasets with ease. Whether you’re building a simple blog or a complex data-driven platform, react-paginate provides the flexibility and functionality needed to create smooth, intuitive pagination experiences.
Remember to style your pagination component to match your application’s design, and consider accessibility when implementing your pagination logic. With react-paginate, you’re well-equipped to tackle the challenges of data presentation and navigation in your React projects.