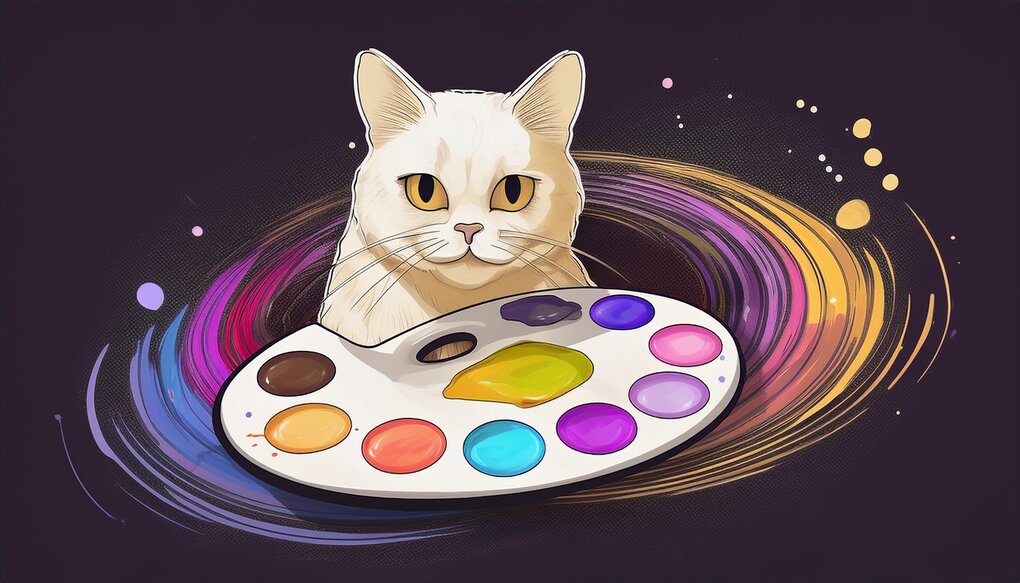
Painting with Pixels: Unleash Your Creativity with react-color-palette
React developers often face the challenge of implementing color selection in their applications. Whether you’re building a design tool, a customization interface, or simply need to offer color options to your users, having a reliable and easy-to-use color picker is essential. Enter react-color-palette, a lightweight yet powerful solution that seamlessly integrates into your React projects.
Features
react-color-palette comes packed with features that make it stand out:
- Lightweight: Minimizes impact on your application’s bundle size.
- Customizable: Offers flexibility in layout and component usage.
- Multiple Color Formats: Supports HEX, RGB, HSV, and named colors.
- Accessibility: Includes keyboard navigation for improved usability.
- Controlled and Uncontrolled Modes: Fits various state management needs.
- TypeScript Support: Provides type definitions for enhanced development experience.
Installation
Getting started with react-color-palette is straightforward. You can install it using either npm or yarn:
# Using npm
npm install react-color-palette
# Using yarn
yarn add react-color-palette
Once installed, you’ll also need to import the CSS file in your project:
import "react-color-palette/css";
Basic Usage
Let’s dive into how you can quickly implement a color picker in your React application:
import React from 'react';
import { ColorPicker, useColor } from "react-color-palette";
import "react-color-palette/css";
export function App() {
const [color, setColor] = useColor("#561ecb");
return <ColorPicker color={color} onChange={setColor} />;
}
In this example, we’re using the useColor
hook to manage the color state. The ColorPicker
component is then rendered with the current color and an onChange handler to update the color when the user makes a selection.
Advanced Usage
Custom Layout
One of the strengths of react-color-palette is its flexibility. You can create custom layouts using individual components:
import React from 'react';
import { Saturation, Hue, useColor } from "react-color-palette";
import "react-color-palette/css";
export function CustomColorPicker() {
const [color, setColor] = useColor("hsl(120 100% 50% / .5)");
return (
<div className="custom-layout">
<Saturation height={300} color={color} onChange={setColor} />
<Hue color={color} onChange={setColor} />
</div>
);
}
This custom layout uses the Saturation
and Hue
components separately, allowing for more control over the picker’s appearance.
Hiding Specific Inputs
You can customize which color format inputs are displayed:
import React from 'react';
import { ColorPicker, useColor } from "react-color-palette";
import "react-color-palette/css";
export function HexOnlyPicker() {
const [color, setColor] = useColor("#123123");
return (
<ColorPicker
hideInput={["rgb", "hsv"]}
color={color}
onChange={setColor}
/>
);
}
This example hides the RGB and HSV inputs, leaving only the HEX input visible.
Using the onChangeComplete Callback
For scenarios where you need to perform an action when the user finishes selecting a color, you can use the onChangeComplete
prop:
import React from 'react';
import { ColorPicker, useColor, IColor } from "react-color-palette";
import "react-color-palette/css";
export function PersistentColorPicker() {
const [color, setColor] = useColor("#123123");
const onChangeComplete = (color: IColor) => {
localStorage.setItem("userColor", color.hex);
};
return (
<ColorPicker
color={color}
onChange={setColor}
onChangeComplete={onChangeComplete}
/>
);
}
This example saves the selected color to localStorage when the user completes their selection.
Accessibility Considerations
react-color-palette
includes keyboard navigation, making it accessible to users who rely on keyboard input. The arrow keys can be used to navigate through color options, and the Enter key selects the chosen color.
Conclusion
react-color-palette
offers a robust solution for adding color selection capabilities to your React applications. Its lightweight nature, customizable components, and support for multiple color formats make it a versatile choice for developers. Whether you’re building a simple color picker or a complex design tool, this library provides the flexibility and ease of use you need to bring color to your user interfaces.
By leveraging react-color-palette, you can enhance your application’s functionality and user experience, allowing your users to express their creativity through color. As you continue to explore the library’s capabilities, you’ll find that it opens up a spectrum of possibilities for your React projects.