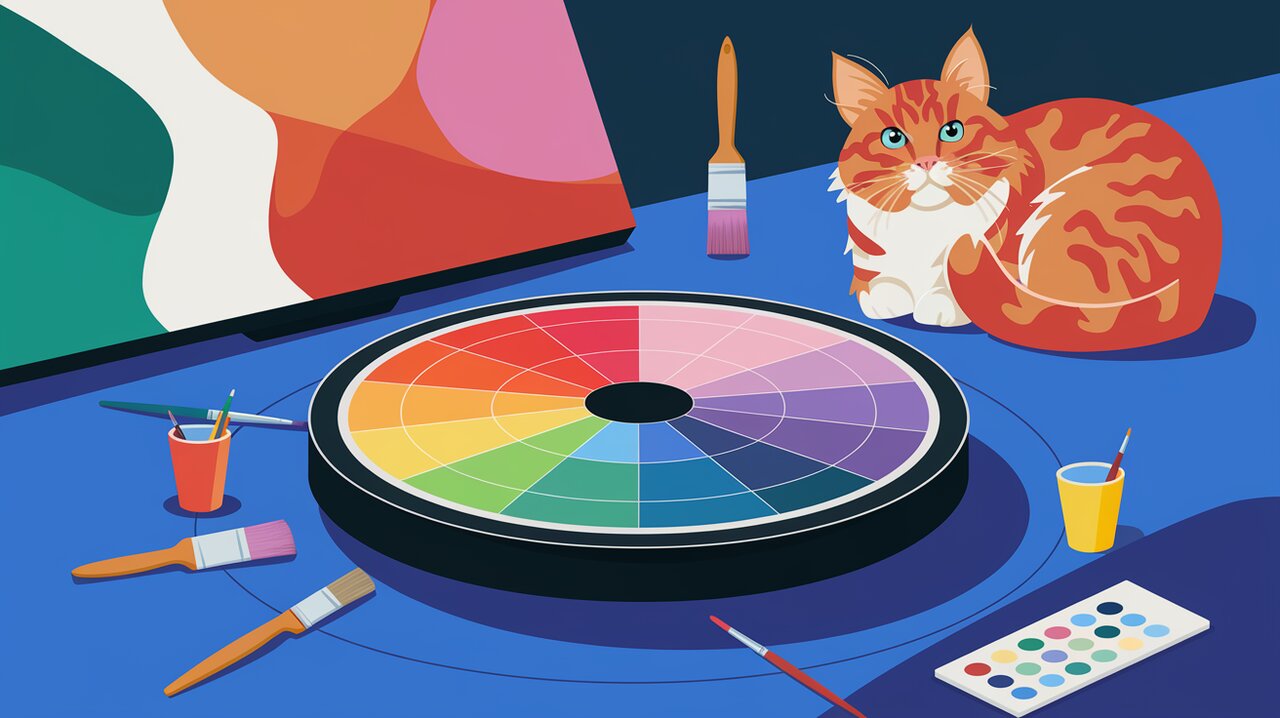
Painting with Pixels: Unleash the Power of react-input-color in React
React Input Color is a powerful and user-friendly color picker component for React applications. Inspired by the sleek design of Sketch’s color picker, this library offers developers an elegant solution for integrating color selection functionality into their projects. With its intuitive interface and robust features, react-input-color
simplifies the process of working with colors in web applications.
Vibrant Features
React Input Color comes packed with a range of features that make it stand out:
- HSV (Hue, Saturation, Value) color model support
- Hex and RGBA color format compatibility
- Customizable placement options
- Emotion-based styling for easy integration
- Support for 6-digit (#RRGGBB) and 8-digit (#RRGGBBAA) hex values
Setting Up Your Palette
To begin using React Input Color in your project, you’ll need to install it first. You can do this using either npm or yarn:
npm install react-input-color --save
Or if you prefer yarn:
yarn add react-input-color
Brushing Up on Basic Usage
Let’s start with a simple example to demonstrate how to implement React Input Color in your React application.
Creating a Color Picker Component
Here’s a basic implementation of the color picker:
import React, { useState } from 'react';
import InputColor from 'react-input-color';
function ColorPicker() {
const [color, setColor] = useState({});
return (
<div>
<InputColor
initialValue="#5e72e4"
onChange={setColor}
placement="right"
/>
<div
style={{
width: 50,
height: 50,
marginTop: 20,
backgroundColor: color.rgba,
}}
/>
</div>
);
}
In this example, we create a simple color picker component. The InputColor
component is initialized with a default color value of “#5e72e4”. When the user selects a new color, the onChange
function updates the color
state. We also display a small square div that reflects the currently selected color.
Understanding the Color Object
The color
object returned by React Input Color contains several useful properties:
interface ColorObject {
hex: string; // e.g., "#ff0000"
rgb: { r: number; g: number; b: number }; // e.g., { r: 255, g: 0, b: 0 }
rgba: string; // e.g., "rgba(255, 0, 0, 1)"
hsl: { h: number; s: number; l: number }; // e.g., { h: 0, s: 100, l: 50 }
}
You can use these properties to work with the selected color in various formats, depending on your application’s needs.
Advanced Techniques for Color Mastery
Now that we’ve covered the basics, let’s explore some more advanced usage scenarios of React Input Color.
Customizing Placement
The placement
prop allows you to control where the color picker popup appears relative to the input:
import React, { useState } from 'react';
import InputColor from 'react-input-color';
function CustomPlacementPicker() {
const [color, setColor] = useState({});
return (
<div>
<InputColor
initialValue="#3498db"
onChange={setColor}
placement="top"
/>
<InputColor
initialValue="#e74c3c"
onChange={setColor}
placement="bottom"
/>
<InputColor
initialValue="#2ecc71"
onChange={setColor}
placement="left"
/>
<InputColor
initialValue="#f39c12"
onChange={setColor}
placement="right"
/>
</div>
);
}
This example demonstrates how to create multiple color pickers with different placement options, giving you flexibility in designing your user interface.
Working with Alpha Values
React Input Color supports 8-digit hex values, allowing you to work with colors that have an alpha (transparency) component:
import React, { useState } from 'react';
import InputColor from 'react-input-color';
function AlphaColorPicker() {
const [color, setColor] = useState({});
return (
<div>
<InputColor
initialValue="#3498db80"
onChange={setColor}
/>
<div
style={{
width: 100,
height: 100,
marginTop: 20,
backgroundColor: color.rgba,
border: '1px solid black',
}}
/>
<p>Selected color: {color.rgba}</p>
</div>
);
}
In this example, we initialize the color picker with a semi-transparent blue color (#3498db80). The resulting color object will include the alpha value, which you can see in the rgba
property.
Creating a Color Palette Selector
You can use multiple React Input Color components to create a color palette selector:
import React, { useState } from 'react';
import InputColor from 'react-input-color';
function ColorPaletteSelector() {
const [palette, setPalette] = useState({
primary: {},
secondary: {},
accent: {},
});
const updateColor = (key: string) => (color: any) => {
setPalette(prevPalette => ({
...prevPalette,
[key]: color,
}));
};
return (
<div>
<h3>Select Your Color Palette</h3>
<div>
<label>Primary Color:</label>
<InputColor
initialValue="#3498db"
onChange={updateColor('primary')}
/>
</div>
<div>
<label>Secondary Color:</label>
<InputColor
initialValue="#e74c3c"
onChange={updateColor('secondary')}
/>
</div>
<div>
<label>Accent Color:</label>
<InputColor
initialValue="#2ecc71"
onChange={updateColor('accent')}
/>
</div>
<div style={{ marginTop: 20 }}>
<div style={{ backgroundColor: palette.primary.rgba, width: 100, height: 50 }} />
<div style={{ backgroundColor: palette.secondary.rgba, width: 100, height: 50 }} />
<div style={{ backgroundColor: palette.accent.rgba, width: 100, height: 50 }} />
</div>
</div>
);
}
This example creates a color palette selector with three color pickers for primary, secondary, and accent colors. The selected colors are displayed in a preview section below the pickers.
Wrapping Up
React Input Color offers a powerful and flexible solution for implementing color selection in React applications. Its intuitive interface, inspired by Sketch’s color picker, provides a familiar and user-friendly experience for your users. Whether you’re building a design tool, a theming system, or any application that requires color input, this library simplifies the process while offering advanced features like alpha channel support and customizable placement.
By leveraging the examples and techniques discussed in this article, you can create sophisticated color selection interfaces that enhance the functionality and user experience of your React applications. Remember to explore the library’s documentation for even more advanced usage scenarios and customization options. Happy color picking!