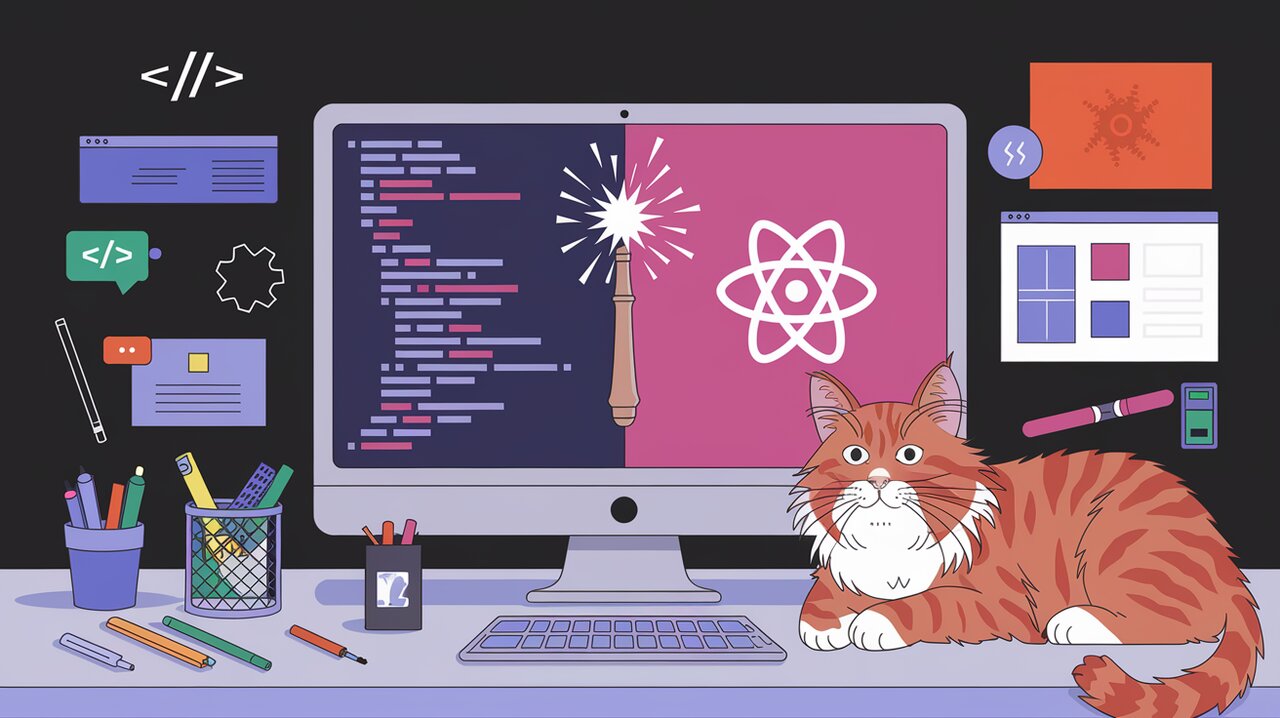
Transforming HTML Strings into React Elements with html-react-parser
The html-react-parser library is a powerful tool that allows developers to convert HTML strings into React elements. This versatile parser works seamlessly on both the server (Node.js) and the client (browser), making it an essential utility for many React applications.
Features
- Converts HTML strings to React elements
- Works on both server-side and client-side
- Supports custom element replacement
- Handles nested HTML structures
- Preserves attributes and styles
- TypeScript support
Installation
You can install html-react-parser using npm or yarn:
npm install html-react-parser
yarn add html-react-parser
For those who prefer using a CDN, you can include the library in your HTML file:
<script src="https://unpkg.com/react@18/umd/react.production.min.js"></script>
<script src="https://unpkg.com/html-react-parser@latest/dist/html-react-parser.min.js"></script>
Basic Usage
Parsing Simple HTML
To start using html-react-parser, import it into your React component:
import parse from 'html-react-parser';
Now you can parse HTML strings into React elements:
const htmlString = '<p>Hello, <strong>World!</strong></p>';
const reactElement = parse(htmlString);
This will create a React element equivalent to:
<p>Hello, <strong>World!</strong></p>
Handling Multiple Elements
The parser can handle multiple elements as well:
const htmlString = '<li>Item 1</li><li>Item 2</li>';
const reactElements = parse(htmlString);
Remember to wrap multiple elements in a parent container when rendering:
<ul>{reactElements}</ul>
Parsing Nested Elements
html-react-parser can handle complex nested structures:
const htmlString = '<div><p>Nested <em>content</em></p></div>';
const reactElement = parse(htmlString);
Advanced Usage
Custom Element Replacement
One of the most powerful features of html-react-parser is the ability to replace elements with custom React components:
import parse, { domToReact, Element } from 'html-react-parser';
const CustomButton = ({ color, children }) => (
<button style={{ color }}>{children}</button>
);
const htmlString = '<div>Click <button color="red">here</button></div>';
const options = {
replace: (domNode) => {
if (domNode instanceof Element && domNode.name === 'button') {
return <CustomButton color={domNode.attribs.color}>{domToReact(domNode.children)}</CustomButton>;
}
}
};
const reactElement = parse(htmlString, options);
This example replaces all <button>
elements with a custom CustomButton
component, preserving attributes and children.
Handling Attributes
html-react-parser automatically converts HTML attributes to React props:
const htmlString = '<div id="main" class="container" data-custom="value"></div>';
const reactElement = parse(htmlString);
This will create a React element with the appropriate props:
<div id="main" className="container" data-custom="value"></div>
Server-Side Rendering
html-react-parser works seamlessly in server-side rendering scenarios:
import parse from 'html-react-parser';
import { renderToString } from 'react-dom/server';
const htmlString = '<p>Server-side rendered content</p>';
const reactElement = parse(htmlString);
const serverRenderedString = renderToString(reactElement);
Conclusion
The html-react-parser library offers a robust solution for converting HTML strings into React elements. Its flexibility, ease of use, and support for both client and server-side rendering make it an invaluable tool for React developers. Whether you’re working with content from a CMS, legacy HTML, or dynamically generated markup, html-react-parser provides the functionality you need to seamlessly integrate HTML into your React applications.
By leveraging its advanced features like custom element replacement and attribute handling, you can create powerful and flexible components that bridge the gap between traditional HTML and modern React development. As you explore the capabilities of html-react-parser, you’ll find it to be an essential addition to your React toolkit, enabling you to handle a wide range of HTML parsing scenarios with ease and efficiency.