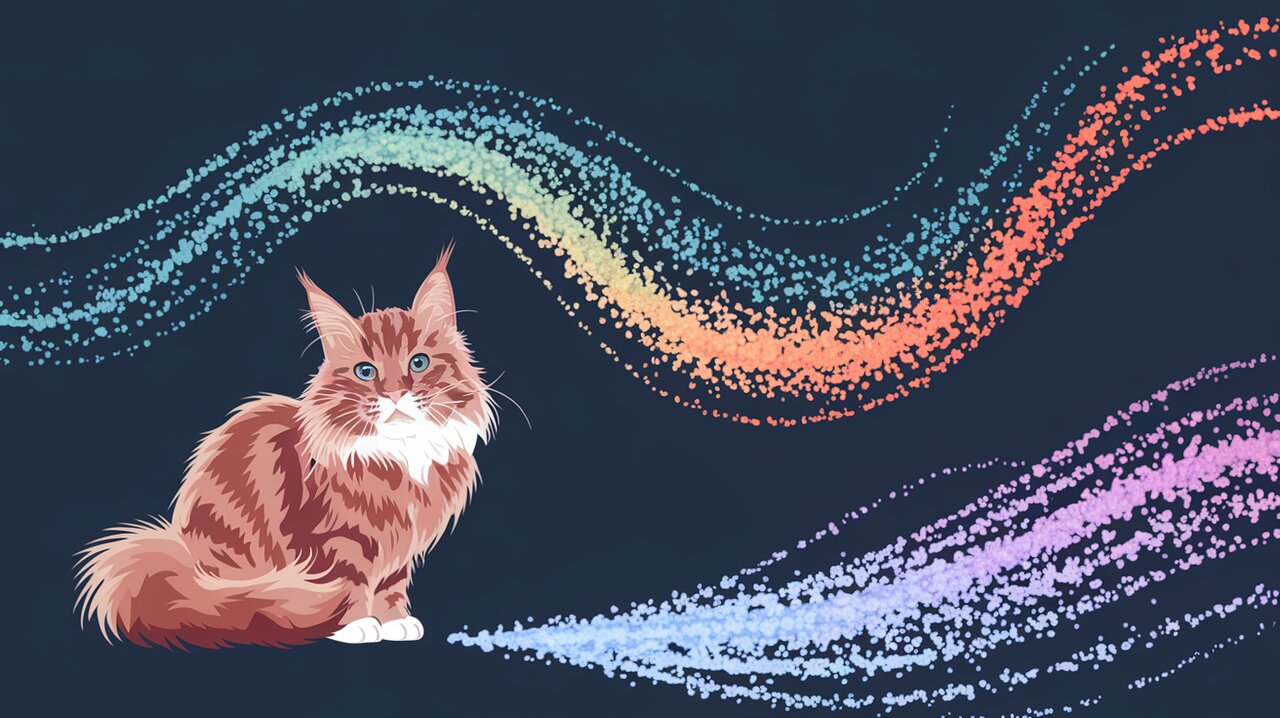
Particle Party: Unleash the Power of react-tsparticles
React developers, are you ready to add some sparkle to your applications? Say hello to react-tsparticles, the library that’s about to become your new best friend for creating stunning visual effects. Whether you want to add a touch of whimsy to your landing page or create an immersive interactive experience, react-tsparticles has got you covered. Let’s dive in and discover how to throw a particle party in your React apps!
Particle Power: What Can react-tsparticles Do?
Before we start coding, let’s take a quick look at what makes react-tsparticles so special:
- Highly customizable particle effects
- Interactive animations responding to user input
- Optimized performance for smooth rendering
- Easy integration with React applications
- Support for various particle shapes and behaviors
- Ability to create complex animations like fireworks and confetti
Getting the Party Started: Installation
First things first, let’s get react-tsparticles into your project. Open your terminal and run:
npm install @tsparticles/react @tsparticles/engine
Or if you’re a yarn fan:
yarn add @tsparticles/react @tsparticles/engine
Basic Usage: Your First Particle Animation
Let’s create a simple particle animation to get you started. Here’s a basic example:
import { useCallback } from "react";
import Particles from "@tsparticles/react";
import { loadSlim } from "@tsparticles/slim";
import type { Engine } from "@tsparticles/engine";
const App = () => {
const particlesInit = useCallback(async (engine: Engine) => {
await loadSlim(engine);
}, []);
return (
<div className="App">
<h1>Welcome to the Particle Party!</h1>
<Particles
id="tsparticles"
init={particlesInit}
options={{
background: {
color: {
value: "#0d47a1",
},
},
particles: {
color: {
value: "#ffffff",
},
links: {
color: "#ffffff",
distance: 150,
enable: true,
opacity: 0.5,
width: 1,
},
move: {
enable: true,
speed: 6,
},
number: {
value: 80,
},
opacity: {
value: 0.5,
},
shape: {
type: "circle",
},
size: {
value: { min: 1, max: 5 },
},
},
}}
/>
</div>
);
};
export default App;
This code creates a simple particle animation with white circles connected by lines on a blue background. The particles move around and interact with each other, creating a mesmerizing effect.
Customization: Making It Your Own
One of the best things about react-tsparticles is how customizable it is. Let’s explore some ways to make your particle animation unique:
Shapes and Colors
Want stars instead of circles? Or maybe a mix of different shapes? No problem!
particles: {
color: {
value: ["#ff0000", "#00ff00", "#0000ff"],
},
shape: {
type: ["circle", "triangle", "star"],
},
// ... other options
}
Interactivity
Make your particles respond to user actions:
interactivity: {
events: {
onClick: {
enable: true,
mode: "push",
},
onHover: {
enable: true,
mode: "repulse",
},
},
modes: {
push: {
quantity: 4,
},
repulse: {
distance: 200,
},
},
},
This configuration allows users to add particles by clicking and repel them by hovering.
Advanced Techniques: Particle Wizardry
Ready to take your particle game to the next level? Let’s look at some advanced techniques.
Confetti Explosion
Celebrate user achievements with a confetti explosion:
import { useCallback } from "react";
import Particles from "@tsparticles/react";
import { loadConfettiPreset } from "tsparticles-preset-confetti";
const ConfettiComponent = () => {
const particlesInit = useCallback(async (engine) => {
await loadConfettiPreset(engine);
}, []);
return (
<Particles
id="tsparticles"
init={particlesInit}
options={{
preset: "confetti",
emitters: {
position: { x: 50, y: 50 },
rate: { quantity: 50, delay: 0.15 },
},
}}
/>
);
};
Fireworks Display
Create a stunning fireworks effect for special occasions:
import { useCallback } from "react";
import Particles from "@tsparticles/react";
import { loadFireworksPreset } from "tsparticles-preset-fireworks";
const FireworksComponent = () => {
const particlesInit = useCallback(async (engine) => {
await loadFireworksPreset(engine);
}, []);
return (
<Particles
id="tsparticles"
init={particlesInit}
options={{
preset: "fireworks",
}}
/>
);
};
Performance Optimization: Keeping the Party Smooth
While particle animations are fun, we don’t want them to slow down our app. Here are some tips to keep things running smoothly:
- Use
loadSlim
instead ofloadFull
when initializing, unless you need all features. - Adjust the
fpsLimit
option to control the animation frame rate. - Reduce the number of particles for better performance on lower-end devices.
- Use the
detectRetina
option to optimize for high-DPI displays.
options={{
fpsLimit: 60,
particles: {
number: {
value: 50,
density: {
enable: true,
area: 800,
},
},
},
detectRetina: true,
}}
Wrapping Up: Your Particle Journey Begins
Congratulations! You’re now equipped to create amazing particle animations in your React applications. From simple backgrounds to interactive explosions, react-tsparticles opens up a world of creative possibilities.
Remember, the key to great particle effects is experimentation. Don’t be afraid to play around with different configurations and see what you can create. Your users will be delighted by the dynamic and engaging experiences you can now offer.
As you continue your particle adventure, you might want to explore other React animation techniques. Check out our articles on Animate with Framer Motion for more ways to bring your UI to life, or dive into React Web Animation Magic for even more animation goodness.
Now go forth and let your creativity explode like a fireworks display! Happy coding, and may your React apps be forever particle-powered!