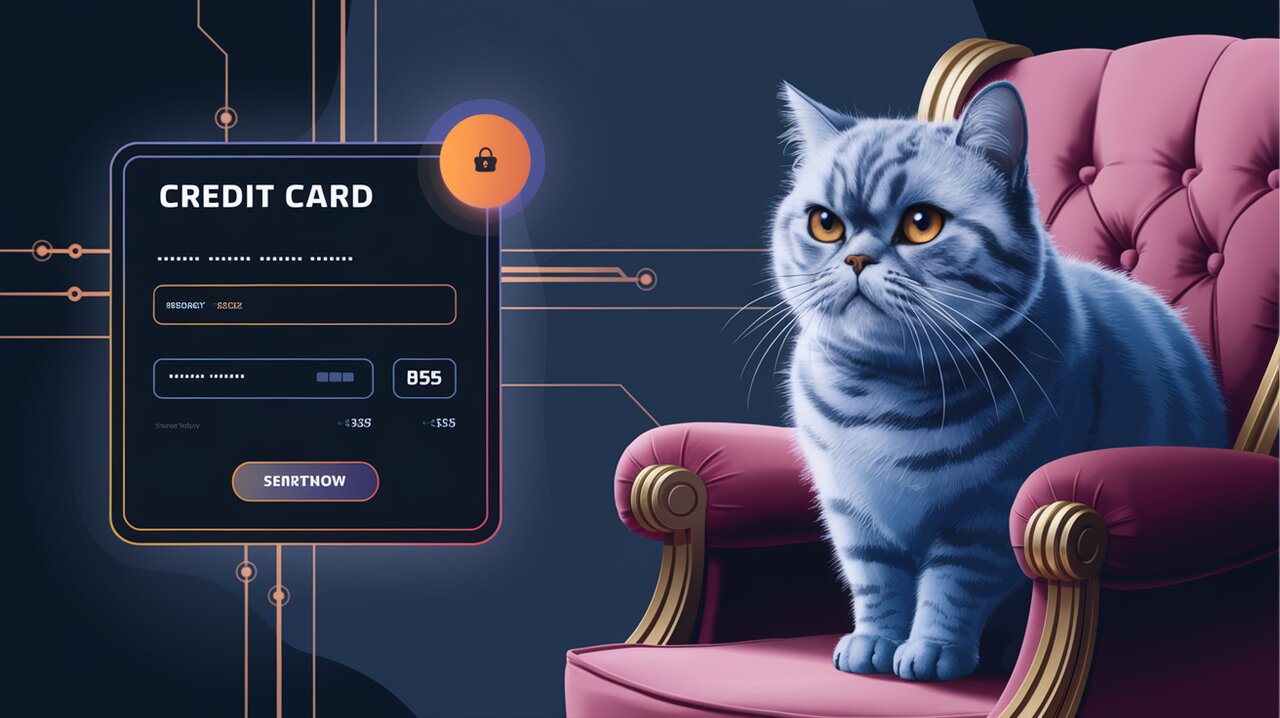
Orchestrating Payment Forms with react-payment-inputs
React Payment Inputs is a powerful library that simplifies the process of creating credit card input forms in React applications. With its intuitive API and robust validation features, it enables developers to build secure and user-friendly payment interfaces with minimal effort. Let’s dive into the world of react-payment-inputs and explore how it can enhance your React projects.
Features
react-payment-inputs comes packed with a set of features that make it stand out:
- Customizable input fields for card number, expiry date, and CVC
- Real-time validation and formatting
- Support for various card types
- Accessibility-friendly design
- Flexible styling options
- Easy integration with form libraries
Installation
To get started with react-payment-inputs, you’ll need to install it in your React project. You can do this using npm or yarn:
npm install react-payment-inputs --save
or
yarn add react-payment-inputs
Basic Usage
react-payment-inputs offers two main ways to use the library: with hooks or with render props. Let’s explore both approaches.
Using Hooks
The hooks approach provides a clean and modern way to implement payment inputs in your React components. Here’s a basic example:
import React from 'react';
import { usePaymentInputs } from 'react-payment-inputs';
export default function PaymentForm() {
const {
meta,
getCardNumberProps,
getExpiryDateProps,
getCVCProps
} = usePaymentInputs();
return (
<div>
<input {...getCardNumberProps()} />
<input {...getExpiryDateProps()} />
<input {...getCVCProps()} />
{meta.isTouched && meta.error && <span>Error: {meta.error}</span>}
</div>
);
}
In this example, we use the usePaymentInputs
hook to get the necessary props and meta information. The getCardNumberProps
, getExpiryDateProps
, and getCVCProps
functions return the props that should be spread onto the respective input elements.
Using Render Props
For those who prefer the render props pattern, react-payment-inputs provides a PaymentInputsContainer
component:
import React from 'react';
import { PaymentInputsContainer } from 'react-payment-inputs';
export default function PaymentForm() {
return (
<PaymentInputsContainer>
{({ meta, getCardNumberProps, getExpiryDateProps, getCVCProps }) => (
<div>
<input {...getCardNumberProps()} />
<input {...getExpiryDateProps()} />
<input {...getCVCProps()} />
{meta.isTouched && meta.error && <span>Error: {meta.error}</span>}
</div>
)}
</PaymentInputsContainer>
);
}
This approach achieves the same result as the hooks version but uses the render props pattern instead.
Advanced Usage
react-payment-inputs offers several advanced features that allow for greater customization and control over the payment form.
Custom Validation
You can provide custom validation functions for each input field. Here’s an example of a custom card number validator:
const cardNumberValidator = ({ cardNumber, cardType, errorMessages }) => {
if (cardType.displayName !== 'Visa' && cardType.displayName !== 'Mastercard') {
return 'We only accept Visa or Mastercard';
}
return undefined;
};
function PaymentForm() {
const { getCardNumberProps } = usePaymentInputs({ cardNumberValidator });
return <input {...getCardNumberProps()} />;
}
Styled Wrapper
react-payment-inputs provides a styled wrapper component that combines the card number, expiry, and CVC fields with a default style:
import React from 'react';
import { PaymentInputsWrapper, usePaymentInputs } from 'react-payment-inputs';
import images from 'react-payment-inputs/images';
export default function StyledPaymentForm() {
const { wrapperProps, getCardImageProps, getCardNumberProps, getExpiryDateProps, getCVCProps } = usePaymentInputs();
return (
<PaymentInputsWrapper {...wrapperProps}>
<svg {...getCardImageProps({ images })} />
<input {...getCardNumberProps()} />
<input {...getExpiryDateProps()} />
<input {...getCVCProps()} />
</PaymentInputsWrapper>
);
}
This approach provides a quick way to implement a styled payment form with minimal effort.
Integration with Form Libraries
react-payment-inputs can be easily integrated with popular form libraries like Formik. Here’s an example:
import { Formik, Field } from 'formik';
import { PaymentInputsWrapper, usePaymentInputs } from 'react-payment-inputs';
function PaymentForm() {
const { meta, getCardImageProps, getCardNumberProps, getExpiryDateProps, getCVCProps, wrapperProps } = usePaymentInputs();
return (
<Formik
initialValues={{ cardNumber: '', expiryDate: '', cvc: '' }}
onSubmit={data => console.log(data)}
validate={() => {
let errors = {};
if (meta.erroredInputs.cardNumber) {
errors.cardNumber = meta.erroredInputs.cardNumber;
}
if (meta.erroredInputs.expiryDate) {
errors.expiryDate = meta.erroredInputs.expiryDate;
}
if (meta.erroredInputs.cvc) {
errors.cvc = meta.erroredInputs.cvc;
}
return errors;
}}
>
{({ handleSubmit }) => (
<form onSubmit={handleSubmit}>
<PaymentInputsWrapper {...wrapperProps}>
<svg {...getCardImageProps({ images })} />
<Field name="cardNumber">
{({ field }) => (
<input {...getCardNumberProps({ onBlur: field.onBlur, onChange: field.onChange })} />
)}
</Field>
<Field name="expiryDate">
{({ field }) => (
<input {...getExpiryDateProps({ onBlur: field.onBlur, onChange: field.onChange })} />
)}
</Field>
<Field name="cvc">
{({ field }) => (
<input {...getCVCProps({ onBlur: field.onBlur, onChange: field.onChange })} />
)}
</Field>
</PaymentInputsWrapper>
<button type="submit">Submit</button>
</form>
)}
</Formik>
);
}
This integration allows you to leverage Formik’s form handling capabilities while using react-payment-inputs for the payment-specific input fields.
Conclusion
react-payment-inputs is a versatile and powerful library that simplifies the creation of payment forms in React applications. Its flexible API, robust validation features, and easy integration with other libraries make it an excellent choice for developers looking to implement secure and user-friendly payment interfaces.
By using react-payment-inputs, you can significantly reduce the time and effort required to build payment forms, allowing you to focus on other aspects of your application. Whether you’re building a small e-commerce site or a large-scale payment processing system, react-payment-inputs provides the tools you need to create a smooth and reliable payment experience for your users.
For more insights on enhancing your React applications, check out our articles on react-credit-cards for additional credit card form functionality, and react-stripe-js-easy-integration for integrating with the Stripe payment platform.