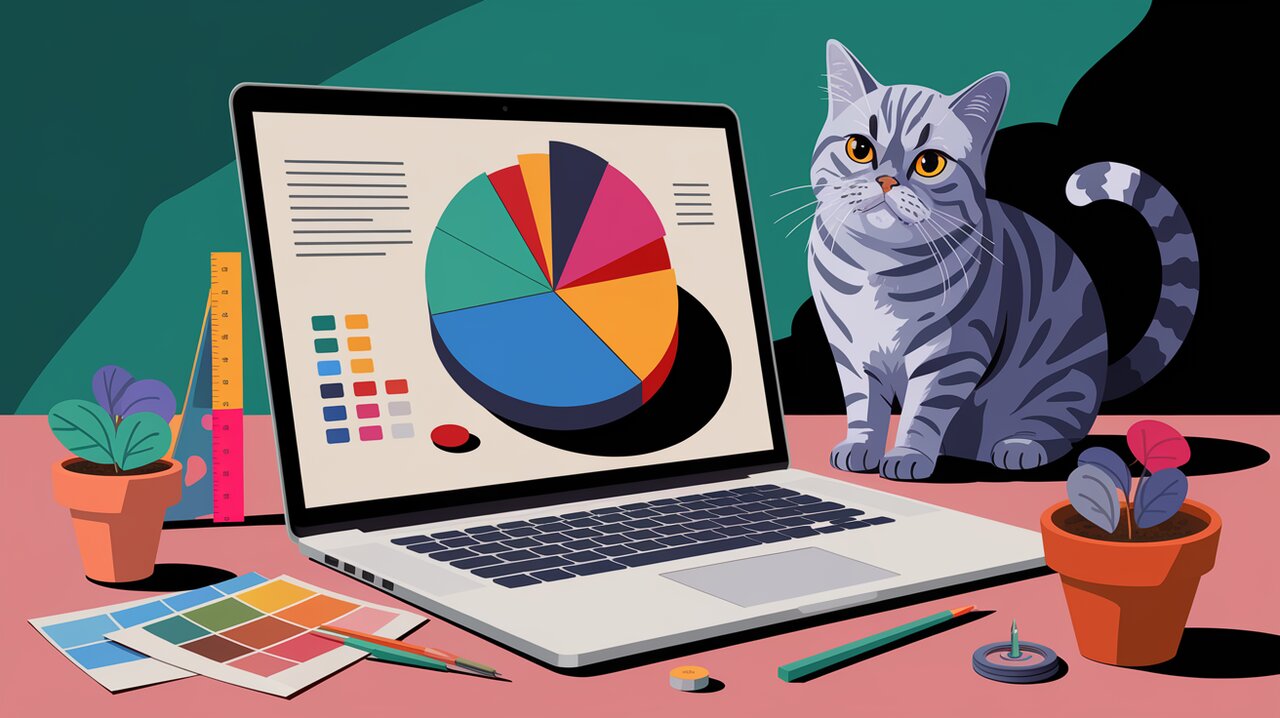
Slice and Dice Data with React Minimal Pie Chart
React Minimal Pie Chart is a lightweight and versatile library for creating stunning pie charts in React applications. With its small footprint and powerful features, it’s the perfect solution for developers who want to add beautiful data visualizations to their projects without sacrificing performance.
Features
React Minimal Pie Chart comes packed with an array of features that make it stand out:
- Incredibly lightweight at just ~2kB gzipped
- Supports pie, donut, and completion charts
- Customizable chart labels and CSS animations
- Written in TypeScript for improved developer experience
- No dependencies, keeping your project lean
Installation
Getting started with React Minimal Pie Chart is a breeze. You can install it using npm or yarn:
npm install react-minimal-pie-chart
or
yarn add react-minimal-pie-chart
Basic Usage
Let’s dive into creating your first pie chart with React Minimal Pie Chart:
import { PieChart } from 'react-minimal-pie-chart';
const MyPieChart = () => {
return (
<PieChart
data={[
{ title: 'One', value: 10, color: '#E38627' },
{ title: 'Two', value: 15, color: '#C13C37' },
{ title: 'Three', value: 20, color: '#6A2135' },
]}
/>
);
};
This simple example creates a basic pie chart with three segments. The data
prop is an array of objects, each representing a segment of the pie chart. The title
is used for accessibility, value
determines the size of the segment, and color
sets its appearance.
Advanced Customization
React Minimal Pie Chart offers extensive customization options to tailor your charts to your specific needs.
Donut Charts
Creating a donut chart is as simple as adjusting the lineWidth
prop:
<PieChart
data={[
{ title: 'One', value: 10, color: '#E38627' },
{ title: 'Two', value: 15, color: '#C13C37' },
{ title: 'Three', value: 20, color: '#6A2135' },
]}
lineWidth={20} // Percentage of chart's radius
/>
Custom Labels
You can add custom labels to your chart using the label
prop:
<PieChart
data={[
{ title: 'One', value: 10, color: '#E38627' },
{ title: 'Two', value: 15, color: '#C13C37' },
{ title: 'Three', value: 20, color: '#6A2135' },
]}
label={({ dataEntry }) => `${Math.round(dataEntry.percentage)}%`}
labelStyle={{
fontSize: '5px',
fontFamily: 'sans-serif',
}}
/>
This example adds percentage labels to each segment of the pie chart.
Animations
React Minimal Pie Chart supports CSS animations out of the box. Enable them with the animate
prop:
<PieChart
data={[
{ title: 'One', value: 10, color: '#E38627' },
{ title: 'Two', value: 15, color: '#C13C37' },
{ title: 'Three', value: 20, color: '#6A2135' },
]}
animate
animationDuration={500}
animationEasing="ease-out"
/>
This creates a smooth animation when the chart first renders or when data changes.
Handling User Interactions
React Minimal Pie Chart allows you to handle user interactions with ease. You can add click, hover, and focus events to chart segments:
<PieChart
data={[
{ title: 'One', value: 10, color: '#E38627' },
{ title: 'Two', value: 15, color: '#C13C37' },
{ title: 'Three', value: 20, color: '#6A2135' },
]}
onClick={(event, index) => {
console.log('Segment clicked:', index);
}}
onMouseOver={(event, index) => {
console.log('Segment hovered:', index);
}}
/>
This example logs the index of the clicked or hovered segment to the console.
Accessibility Considerations
React Minimal Pie Chart is built with accessibility in mind. Each segment can have a title
attribute, which is used to provide screen reader information:
<PieChart
data={[
{ title: 'Sales', value: 10, color: '#E38627' },
{ title: 'Marketing', value: 15, color: '#C13C37' },
{ title: 'Development', value: 20, color: '#6A2135' },
]}
/>
This ensures that users relying on assistive technologies can understand the content of your charts.
Performance Optimization
One of the key advantages of React Minimal Pie Chart is its small size and excellent performance. To further optimize your charts, consider the following tips:
-
Use the
segmentsShift
prop to create visual separation between segments instead of usingpaddingAngle
, which can be more computationally expensive. -
If you don’t need labels, avoid using the
label
prop to reduce rendering time. -
For large datasets, consider grouping smaller segments into an “Other” category to improve rendering performance and chart readability.
Conclusion
React Minimal Pie Chart offers a powerful yet lightweight solution for creating beautiful pie charts in React applications. Its small footprint, extensive customization options, and focus on performance make it an excellent choice for developers looking to add data visualization to their projects without compromising on speed or flexibility.
Whether you’re creating simple pie charts or complex donut charts with custom animations and interactions, React Minimal Pie Chart provides the tools you need to bring your data to life. Start slicing and dicing your data today with this versatile library!
For more React charting solutions, check out our articles on Recharts and React Google Charts. These libraries offer different approaches to data visualization that might complement your use of React Minimal Pie Chart.