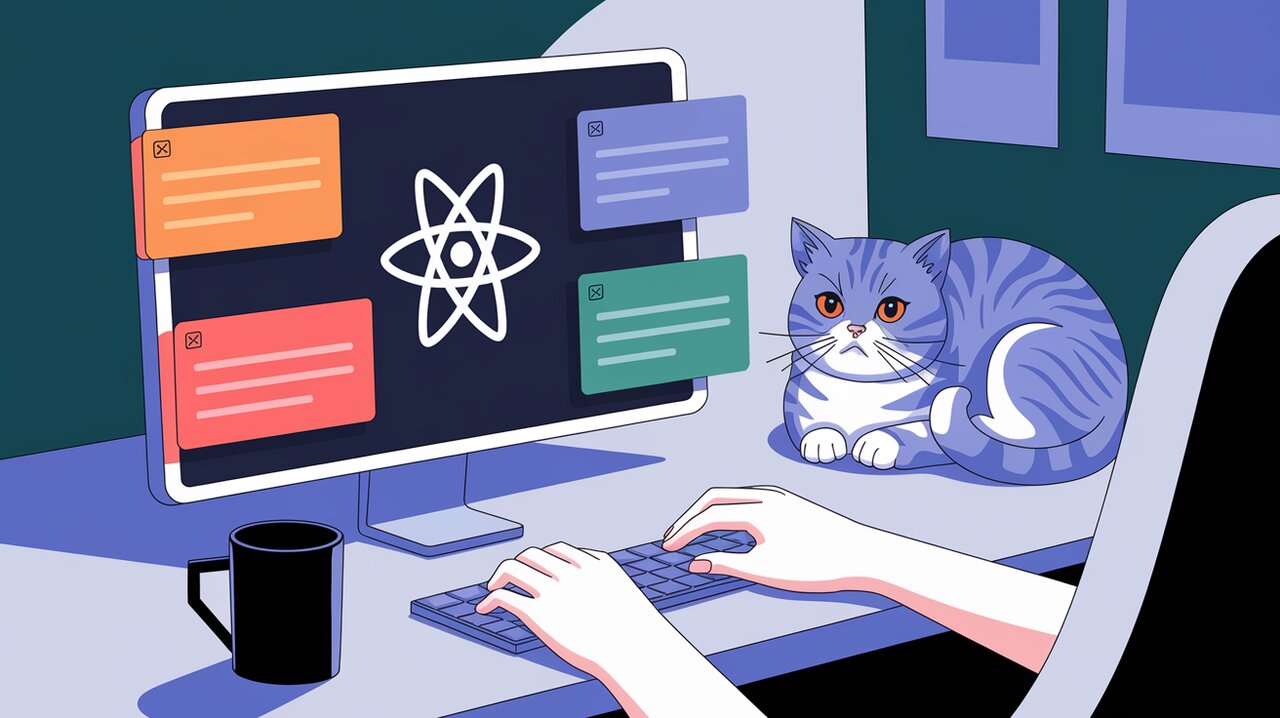
Popping Up with React Tiny Popover: A Lightweight Solution for Dynamic UI Elements
React Tiny Popover is a lightweight and highly customizable library that allows developers to easily add popover functionality to their React applications. With its non-intrusive approach and TypeScript support, it has become a popular choice for creating dynamic and interactive user interfaces.
Features
- Lightweight with no dependencies
- Highly customizable
- Non-intrusive rendering
- TypeScript support
- Boundary detection and repositioning
- Custom positioning options
Installation
To get started with React Tiny Popover, you can install it using npm or yarn:
npm install react-tiny-popover --save
or
yarn add react-tiny-popover
Basic Usage
Let’s start with a simple example of how to use React Tiny Popover in your application:
Creating a Basic Popover
import React, { useState } from 'react';
import { Popover } from 'react-tiny-popover';
const BasicPopover: React.FC = () => {
const [isPopoverOpen, setIsPopoverOpen] = useState(false);
return (
<Popover
isOpen={isPopoverOpen}
positions={['top', 'bottom', 'left', 'right']}
content={<div>Hi! I'm popover content.</div>}
>
<button onClick={() => setIsPopoverOpen(!isPopoverOpen)}>
Toggle Popover
</button>
</Popover>
);
};
In this example, we create a simple button that toggles the popover when clicked. The popover content is a div with some text, and we specify that it can appear in any of the four main positions around the button.
Using Render Props
React Tiny Popover also supports render props, allowing you to access the popover’s state:
import React, { useState } from 'react';
import { Popover } from 'react-tiny-popover';
const RenderPropPopover: React.FC = () => {
const [isPopoverOpen, setIsPopoverOpen] = useState(false);
return (
<Popover
isOpen={isPopoverOpen}
positions={['top', 'bottom', 'left', 'right']}
content={({ position, nudgedLeft, nudgedTop }) => (
<div>
<p>Current position: {position}</p>
<p>Nudged left: {nudgedLeft}px</p>
<p>Nudged top: {nudgedTop}px</p>
</div>
)}
onClickOutside={() => setIsPopoverOpen(false)}
>
<button onClick={() => setIsPopoverOpen(!isPopoverOpen)}>
Toggle Popover
</button>
</Popover>
);
};
This example demonstrates how to use render props to access the popover’s position and nudge values. We also add an onClickOutside
handler to close the popover when clicking outside of it.
Advanced Usage
React Tiny Popover offers several advanced features for more complex use cases.
Custom Positioning
You can use the transform
prop to customize the popover’s position:
import React, { useState } from 'react';
import { Popover } from 'react-tiny-popover';
const CustomPositionPopover: React.FC = () => {
const [isPopoverOpen, setIsPopoverOpen] = useState(false);
return (
<Popover
isOpen={isPopoverOpen}
positions={['bottom']}
content={<div>Custom positioned popover</div>}
transform={{
top: 20,
left: -10,
}}
transformMode="relative"
>
<button onClick={() => setIsPopoverOpen(!isPopoverOpen)}>
Custom Position
</button>
</Popover>
);
};
This example shows how to use the transform
prop to adjust the popover’s position relative to its default placement.
Using ArrowContainer
React Tiny Popover provides an ArrowContainer
component for adding an arrow to your popover:
import React, { useState } from 'react';
import { Popover, ArrowContainer } from 'react-tiny-popover';
const ArrowPopover: React.FC = () => {
const [isPopoverOpen, setIsPopoverOpen] = useState(false);
return (
<Popover
isOpen={isPopoverOpen}
positions={['top', 'bottom', 'left', 'right']}
content={({ position, childRect, popoverRect }) => (
<ArrowContainer
position={position}
childRect={childRect}
popoverRect={popoverRect}
arrowColor="lightblue"
arrowSize={10}
>
<div style={{ backgroundColor: 'lightblue', padding: '10px' }}>
Popover with an arrow!
</div>
</ArrowContainer>
)}
>
<button onClick={() => setIsPopoverOpen(!isPopoverOpen)}>
Arrow Popover
</button>
</Popover>
);
};
This example demonstrates how to use the ArrowContainer
component to add an arrow to your popover, with customizable color and size.
Conclusion
React Tiny Popover offers a lightweight and flexible solution for adding popover functionality to your React applications. Its non-intrusive approach, customization options, and TypeScript support make it an excellent choice for developers looking to enhance their user interfaces with dynamic and interactive elements.
By leveraging the features we’ve explored, such as custom positioning, render props, and the ArrowContainer
component, you can create sophisticated popovers that fit seamlessly into your application’s design. Whether you’re building a simple tooltip or a complex menu system, React Tiny Popover provides the tools you need to create engaging user experiences.