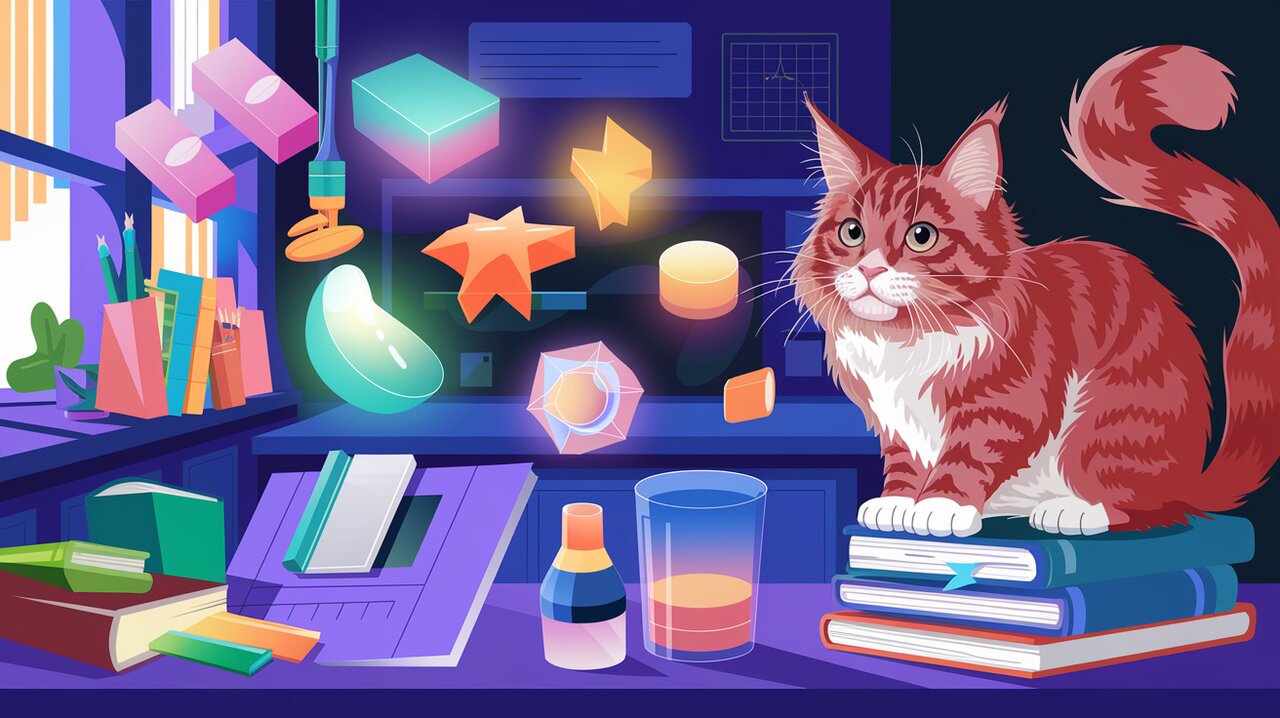
PrimeReact: Unleashing UI Wizardry for React Developers
In the ever-evolving landscape of web development, creating captivating and functional user interfaces is a constant challenge. Enter PrimeReact, a powerful UI component library that equips React developers with the tools they need to craft exceptional web experiences. With its extensive collection of over 90 customizable components, PrimeReact empowers developers to build sophisticated applications while maintaining flexibility and ease of use.
Unveiling the Magic: PrimeReact’s Key Features
PrimeReact stands out in the crowded field of UI libraries, offering a wealth of features that cater to both novice and experienced developers:
- Rich Component Library: From basic buttons to complex data tables, PrimeReact provides a comprehensive set of UI elements to cover all your interface needs.
- Theming Flexibility: Choose from pre-designed themes or create your own using the powerful theming engine, allowing for seamless integration with your project’s visual identity.
- Responsive Design: Built with mobile-first principles, PrimeReact ensures your applications look great on devices of all sizes.
- Accessibility: WCAG 2.0 compliant components make your applications more inclusive and user-friendly for all.
- TypeScript Support: Enjoy top-notch TypeScript integration for enhanced development productivity and code quality.
- Customization Options: Tailor components to your exact specifications with an extensive API and styling options.
Conjuring PrimeReact: Installation and Setup
Getting started with PrimeReact is as simple as casting a spell. Let’s begin by summoning the library into your project:
# Using npm
npm install primereact
# Using yarn
yarn add primereact
Once installed, you can import individual components as needed, ensuring your bundle size remains optimized:
import { Button } from 'primereact/button';
const MyComponent: React.FC = () => {
return <Button label="Cast Spell" />;
};
Mastering the Basics: PrimeReact in Action
Crafting a Simple Form
Let’s dive into a practical example by creating a basic form using PrimeReact components:
import React, { useState } from 'react';
import { InputText } from 'primereact/inputtext';
import { Button } from 'primereact/button';
const SimpleForm: React.FC = () => {
const [name, setName] = useState('');
const handleSubmit = (e: React.FormEvent) => {
e.preventDefault();
console.log('Submitted name:', name);
};
return (
<form onSubmit={handleSubmit}>
<InputText
value={name}
onChange={(e) => setName(e.target.value)}
placeholder="Enter your name"
/>
<Button type="submit" label="Submit" />
</form>
);
};
This example demonstrates how easily you can create interactive forms using PrimeReact’s InputText
and Button
components. The InputText
component handles user input with built-in state management, while the Button
component provides a stylish submit button.
Displaying Data with DataTable
PrimeReact’s DataTable component is a powerful tool for presenting data in a structured format:
import React from 'react';
import { DataTable } from 'primereact/datatable';
import { Column } from 'primereact/column';
interface User {
id: number;
name: string;
email: string;
}
const users: User[] = [
{ id: 1, name: 'Alice', email: 'alice@example.com' },
{ id: 2, name: 'Bob', email: 'bob@example.com' },
{ id: 3, name: 'Charlie', email: 'charlie@example.com' },
];
const UserTable: React.FC = () => {
return (
<DataTable value={users}>
<Column field="id" header="ID" />
<Column field="name" header="Name" />
<Column field="email" header="Email" />
</DataTable>
);
};
The DataTable component automatically handles sorting, pagination, and responsive layout, making it easy to create sophisticated data presentations with minimal code.
Advanced Incantations: Leveraging PrimeReact’s Power
Theming with Style
PrimeReact offers two theming modes: styled and unstyled. Let’s explore how to implement a custom theme:
import React from 'react';
import { PrimeReactProvider } from 'primereact/api';
import { Button } from 'primereact/button';
// Import your custom theme CSS
import './themes/my-custom-theme.css';
const App: React.FC = () => {
return (
<PrimeReactProvider>
<Button label="Styled Button" />
</PrimeReactProvider>
);
};
By wrapping your application with PrimeReactProvider
and importing a custom theme CSS, you can easily apply a consistent look and feel across all PrimeReact components.
Creating Dynamic Dialogs
PrimeReact’s Dialog component allows for creating interactive modal windows:
import React, { useState } from 'react';
import { Button } from 'primereact/button';
import { Dialog } from 'primereact/dialog';
const DynamicDialog: React.FC = () => {
const [visible, setVisible] = useState(false);
return (
<>
<Button label="Open Dialog" onClick={() => setVisible(true)} />
<Dialog
header="Greetings"
visible={visible}
onHide={() => setVisible(false)}
>
<p>Welcome to the magical world of PrimeReact!</p>
</Dialog>
</>
);
};
This example demonstrates how to create a dialog that can be dynamically shown and hidden, providing a smooth user experience for displaying additional information or capturing user input.
Conclusion: Embracing the Power of PrimeReact
PrimeReact offers a treasure trove of UI components and features that empower React developers to create stunning, functional, and accessible web applications. From basic form elements to advanced data visualization tools, PrimeReact provides the building blocks needed to bring your UI visions to life.
By leveraging PrimeReact’s extensive component library, flexible theming system, and responsive design capabilities, you can significantly accelerate your development process while maintaining high-quality, professional-looking interfaces. Whether you’re building a simple landing page or a complex enterprise application, PrimeReact equips you with the tools to craft magical user experiences that captivate and engage your audience.
As you continue your journey with PrimeReact, remember to explore its vast documentation, experiment with different components, and unleash your creativity. The world of UI wizardry awaits – it’s time to cast your spells and create interfaces that truly shine!