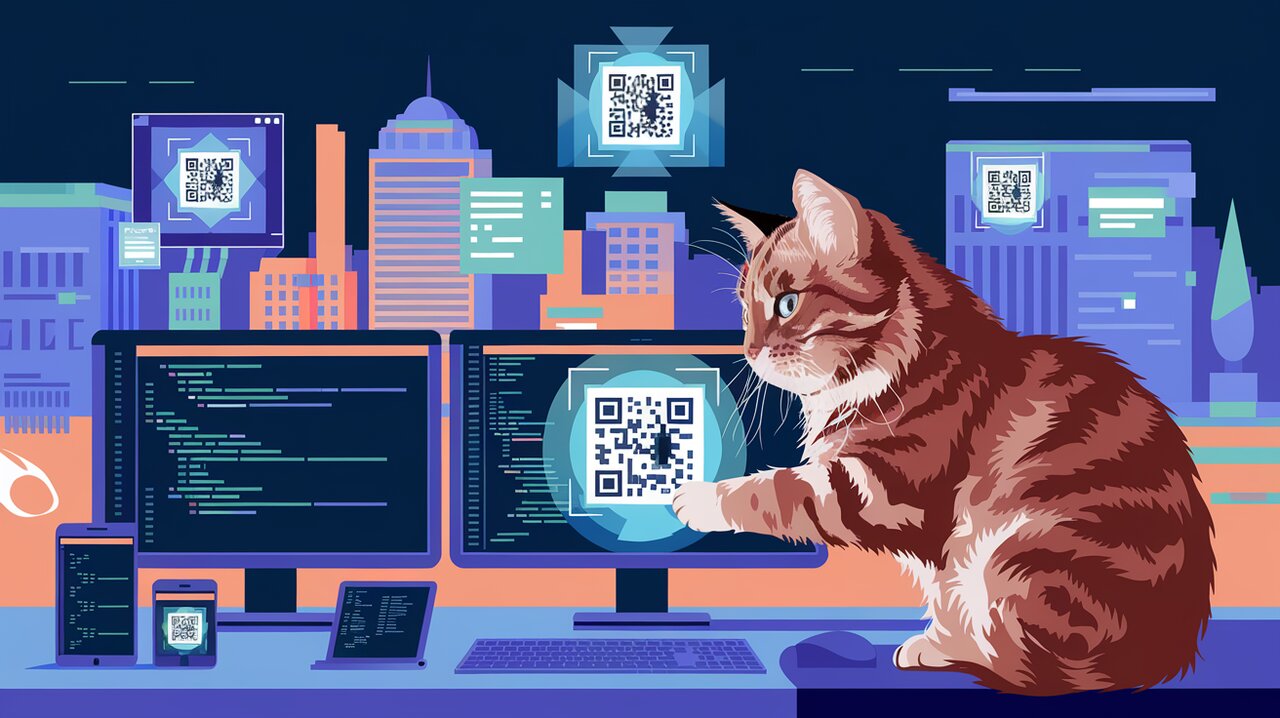
QR Code Wizardry: Conjuring Digital Portals with react-qrcode
In today’s digital age, QR codes have become an indispensable tool for quickly sharing information and connecting the physical world with the digital realm. For React developers looking to incorporate QR code functionality into their applications, the react-qrcode
library offers a powerful and flexible solution. This article will guide you through the process of using react-qrcode
to generate QR codes in your React projects, from basic implementation to advanced customization.
Features
react-qrcode
comes packed with a range of features that make it a versatile choice for QR code generation:
- Easy integration with React components
- Customizable QR code appearance
- Support for various output formats (SVG, Canvas)
- Error correction level adjustment
- Responsive design support
Installation
To get started with react-qrcode
, you’ll need to install it in your React project. You can do this using npm or yarn:
# Using npm
npm install react-qrcode
# Using yarn
yarn add react-qrcode
Basic Usage
Let’s dive into the basics of using react-qrcode
in your React application.
Simple QR Code Generation
To create a basic QR code, you can use the QRCode
component provided by react-qrcode
. Here’s a simple example:
import React from 'react';
import { QRCode } from 'react-qrcode';
const SimpleQRCode: React.FC = () => {
return <QRCode value="https://example.com" />;
};
export default SimpleQRCode;
This code will generate a QR code that, when scanned, will direct users to “https://example.com”. The QRCode
component automatically handles the encoding and rendering of the QR code.
Customizing Size and Color
You can easily customize the appearance of your QR code by adjusting its size and colors:
import React from 'react';
import { QRCode } from 'react-qrcode';
const CustomQRCode: React.FC = () => {
return (
<QRCode
value="https://example.com"
size={256}
fgColor="#0000ff"
bgColor="#ffffff"
/>
);
};
export default CustomQRCode;
In this example, we’ve set the size to 256 pixels, the foreground color to blue, and the background color to white. These properties allow you to match the QR code’s appearance to your application’s design.
Advanced Usage
Now that we’ve covered the basics, let’s explore some more advanced features of react-qrcode
.
Error Correction Levels
QR codes have built-in error correction capabilities. react-qrcode
allows you to adjust the error correction level:
import React from 'react';
import { QRCode } from 'react-qrcode';
const ErrorCorrectionQRCode: React.FC = () => {
return (
<QRCode
value="https://example.com"
errorCorrectionLevel="H"
/>
);
};
export default ErrorCorrectionQRCode;
The errorCorrectionLevel
prop accepts four values: ‘L’, ‘M’, ‘Q’, and ‘H’, with ‘H’ providing the highest level of error correction. This is useful when you expect the QR code might be partially obscured or damaged.
Responsive QR Codes
To create QR codes that adapt to different screen sizes, you can use the style
prop:
import React from 'react';
import { QRCode } from 'react-qrcode';
const ResponsiveQRCode: React.FC = () => {
return (
<QRCode
value="https://example.com"
style={{ width: '100%', height: 'auto', maxWidth: '256px' }}
/>
);
};
export default ResponsiveQRCode;
This approach ensures that the QR code scales appropriately on different devices while maintaining its readability.
Using Canvas Instead of SVG
By default, react-qrcode
renders QR codes as SVG elements. However, you can switch to canvas rendering if needed:
import React from 'react';
import { QRCode } from 'react-qrcode';
const CanvasQRCode: React.FC = () => {
return (
<QRCode
value="https://example.com"
renderAs="canvas"
/>
);
};
export default CanvasQRCode;
Canvas rendering can be useful in certain performance-sensitive scenarios or when working with older browsers.
Adding a Logo to QR Code
For branding purposes, you might want to add a logo to the center of your QR code. While react-qrcode
doesn’t directly support this feature, you can achieve it by overlaying an image on top of the QR code:
import React from 'react';
import { QRCode } from 'react-qrcode';
const LogoQRCode: React.FC = () => {
return (
<div style={{ position: 'relative', display: 'inline-block' }}>
<QRCode
value="https://example.com"
size={256}
style={{ display: 'block' }}
/>
<img
src="/path/to/your/logo.png"
style={{
position: 'absolute',
top: '50%',
left: '50%',
transform: 'translate(-50%, -50%)',
width: '20%',
height: 'auto',
}}
alt="Logo"
/>
</div>
);
};
export default LogoQRCode;
This technique overlays a logo on top of the QR code. Be careful not to make the logo too large, as it might interfere with the QR code’s readability.
Conclusion
The react-qrcode
library provides a powerful and flexible way to generate QR codes in React applications. From simple implementations to advanced customizations, this library offers the tools you need to integrate QR code functionality seamlessly into your projects. By leveraging the features we’ve explored, you can create QR codes that not only serve their functional purpose but also align with your application’s design and user experience goals.
As QR codes continue to play an important role in bridging the physical and digital worlds, mastering libraries like react-qrcode
will undoubtedly enhance your capabilities as a React developer. Whether you’re building e-commerce platforms, event management systems, or any application that benefits from quick information sharing, react-qrcode
is a valuable addition to your toolkit.