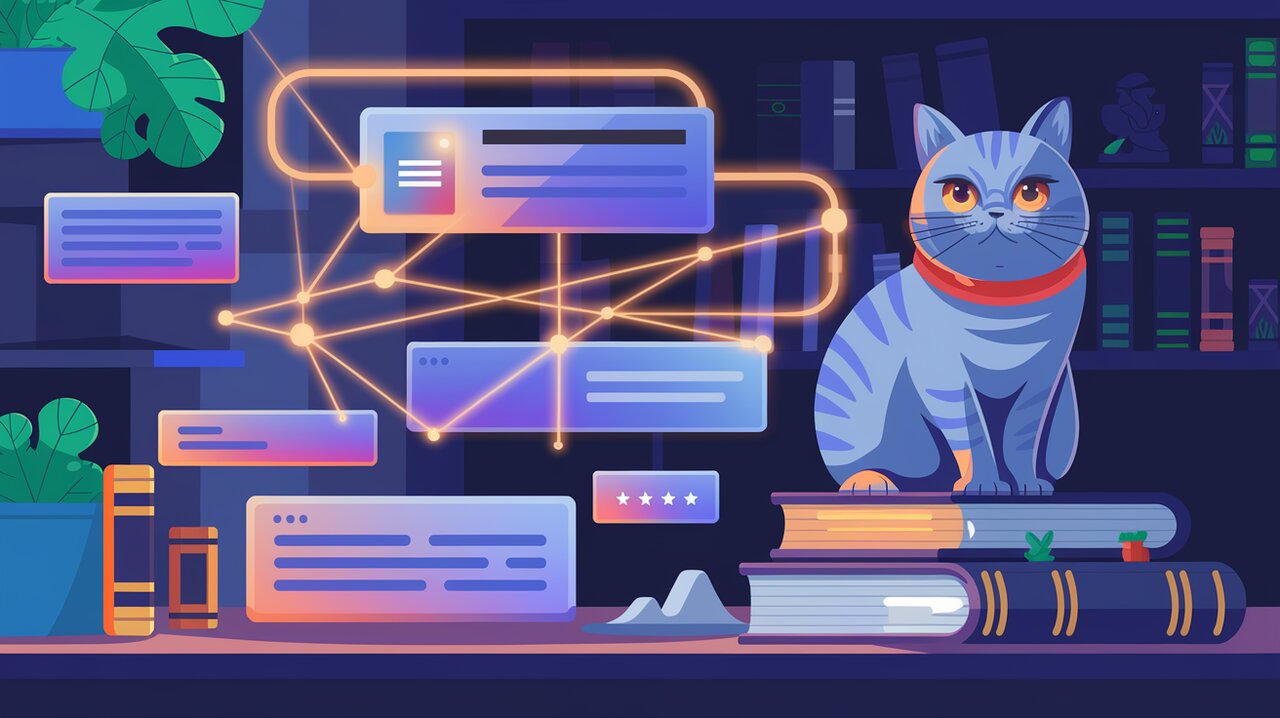
Query Building Wizardry: Unleashing the Power of react-querybuilder
In the realm of React development, creating intuitive and powerful query interfaces can be a daunting task. Enter react-querybuilder, a magical component that transforms the complex art of query building into a delightful experience for both developers and users alike. This versatile library empowers you to craft fully customizable query builders with ease, opening up a world of possibilities for data filtering and exploration in your React applications.
Summoning the Query Builder
Before we dive into the enchanting features of react-querybuilder, let’s begin by summoning it into our project. Open your terminal and cast the following spell:
npm install react-querybuilder
For those who prefer the yarn incantation:
yarn add react-querybuilder
With the library now at your fingertips, it’s time to weave it into the fabric of your React application.
Conjuring Your First Query Builder
To bring the query builder to life, we’ll need to import the necessary components and styles. Add the following incantations to your React component:
import { QueryBuilder } from 'react-querybuilder';
import 'react-querybuilder/dist/query-builder.css';
function MyQueryBuilder() {
const [query, setQuery] = useState({
combinator: 'and',
rules: [],
});
return (
<QueryBuilder
fields={[
{ name: 'firstName', label: 'First Name' },
{ name: 'lastName', label: 'Last Name' },
{ name: 'age', label: 'Age', inputType: 'number' },
]}
query={query}
onQueryChange={q => setQuery(q)}
/>
);
}
This spell creates a basic query builder with fields for first name, last name, and age. The query
state holds the current query configuration, while onQueryChange
updates it as the user interacts with the interface.
Customizing the Enchantment
One of the most powerful aspects of react-querybuilder is its flexibility. Let’s explore some ways to tailor the query builder to your specific needs.
Field of Dreams
The fields
prop is where you define the available fields for querying. Each field can have various properties to control its behavior:
const fields = [
{ name: 'status', label: 'Status', valueEditorType: 'select', values: ['Active', 'Inactive', 'Pending'] },
{ name: 'createdAt', label: 'Created Date', inputType: 'date' },
{ name: 'score', label: 'Score', inputType: 'number', validators: [
{ name: 'min', args: 0 },
{ name: 'max', args: 100 }
]},
];
This enchantment creates fields for status (with predefined options), creation date, and a score with validation.
Styling Sorcery
While react-querybuilder comes with default styles, you can easily customize its appearance to match your application’s theme. Create a custom CSS file and import it after the default styles:
import 'react-querybuilder/dist/query-builder.css';
import './my-query-builder-styles.css';
In your custom CSS, you can override the default styles:
.rqb-rule {
background-color: #f0f8ff;
border: 1px solid #4682b4;
}
.rqb-operator {
color: #8b4513;
}
Validator’s Vigil
To ensure data integrity, react-querybuilder allows you to implement custom validators:
const validators = {
email: ({ value }) => {
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return emailRegex.test(value) ? null : 'Invalid email format';
},
};
<QueryBuilder
fields={fields}
validators={validators}
query={query}
onQueryChange={setQuery}
/>
This spell adds an email validator to ensure proper formatting.
Advanced Incantations
For those seeking to push the boundaries of query building magic, react-querybuilder offers advanced features that can elevate your interface to new heights.
The Art of Translation
If your application caters to a global audience, you’ll be pleased to know that react-querybuilder supports internationalization out of the box:
import { QueryBuilder, defaultTranslations } from 'react-querybuilder';
const frenchTranslations = {
...defaultTranslations,
fields: {
title: 'Champs',
},
operators: {
'=': 'est égal à',
'!=': "n'est pas égal à",
},
};
<QueryBuilder
fields={fields}
query={query}
onQueryChange={setQuery}
translations={frenchTranslations}
/>
This enchantment translates the query builder interface into French, creating a seamless experience for French-speaking users.
Summoning External Data
For dynamic field options, you can use the getValues
prop to fetch data asynchronously:
const getCountries = () => {
return fetch('https://api.example.com/countries')
.then(response => response.json())
.then(data => data.map(country => ({ name: country.code, label: country.name })));
};
<QueryBuilder
fields={[
{ name: 'country', label: 'Country', valueEditorType: 'select', getValues: getCountries },
]}
query={query}
onQueryChange={setQuery}
/>
This spell allows the query builder to dynamically load country options from an API.
Exporting Your Magical Creations
Once users have crafted their queries, you may want to export them for use in other parts of your application. React-querybuilder provides utility functions for this purpose:
import { formatQuery } from 'react-querybuilder';
const sqlQuery = formatQuery(query, 'sql');
console.log(sqlQuery);
This incantation transforms the query into SQL format, ready to be used in your backend or database queries.
Conclusion: Mastering the Art of Query Building
As we conclude our journey through the enchanting world of react-querybuilder, we’ve only scratched the surface of its capabilities. This powerful library offers a perfect blend of simplicity and flexibility, allowing developers to create sophisticated query interfaces with minimal effort.
By harnessing the magic of react-querybuilder, you can empower your users to explore and filter data in ways that were once the domain of database wizards. Whether you’re building a complex data analysis tool or a simple search interface, react-querybuilder provides the spells you need to create intuitive and powerful query experiences.
As you continue your quest to master React development, consider exploring other powerful libraries that can enhance your applications. For instance, you might find the article on react-beautiful-dnd useful for creating drag-and-drop interfaces, or dive into advanced form handling with react-hook-form.
Remember, the true power of react-querybuilder lies not just in its features, but in how you wield them. Experiment, customize, and let your creativity flow. With practice and imagination, you’ll soon be crafting query interfaces that are not just functional, but truly magical.