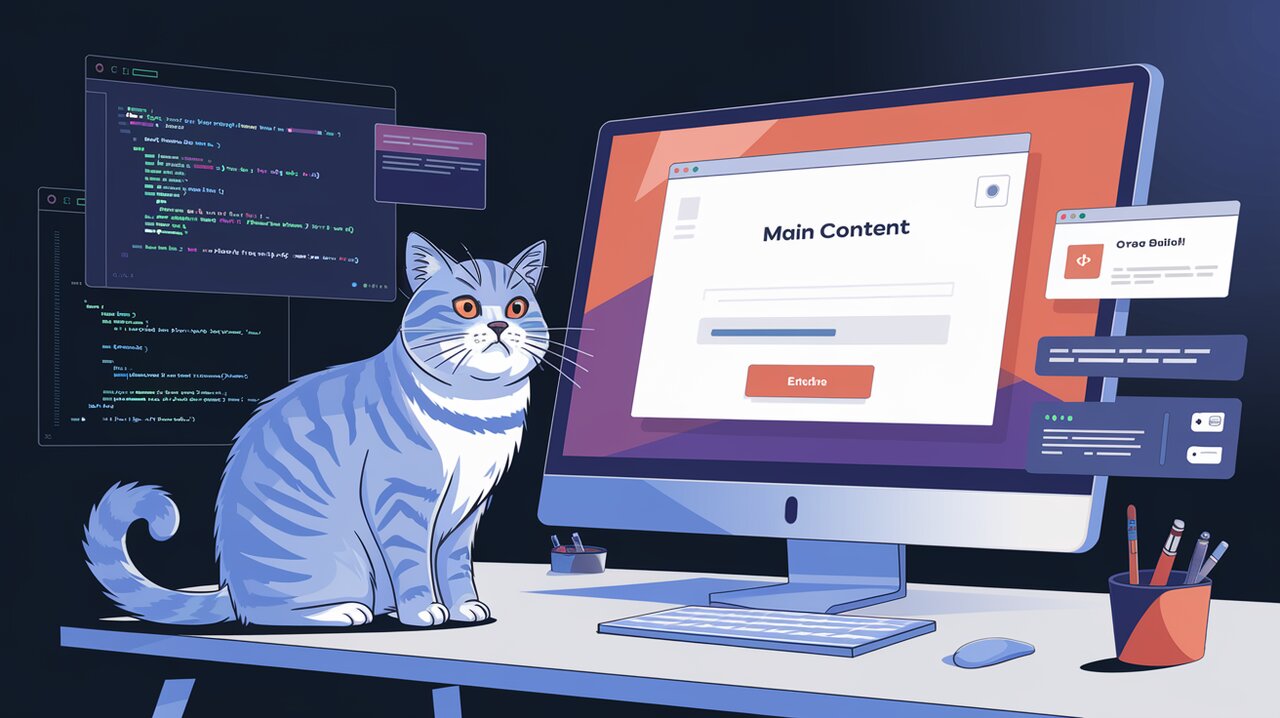
Radix Dialog is a powerful and flexible library for creating accessible modal dialogs in React applications. It provides a set of customizable primitives that allow developers to build feature-rich, responsive, and user-friendly dialog components with ease. Whether you’re creating simple confirmation prompts or complex forms, Radix Dialog offers the tools to craft seamless user experiences.
Unlocking the Power of Radix Dialog
Radix Dialog comes packed with features that make it a go-to choice for React developers:
- Support for both modal and non-modal dialog modes
- Automatic focus trapping for enhanced keyboard navigation
- Flexible control options (controlled and uncontrolled modes)
- Built-in screen reader announcements with Title and Description components
- Automatic closure on Esc key press
Setting Up Your Modal Stage
To begin your journey with Radix Dialog, install the package using npm or yarn:
npm install @radix-ui/react-dialog
# or
yarn add @radix-ui/react-dialog
Crafting Your First Dialog
Let’s dive into creating a basic dialog using Radix Dialog. Here’s a simple example to get you started:
import * as Dialog from '@radix-ui/react-dialog';
import React from 'react';
const SimpleDialog: React.FC = () => (
<Dialog.Root>
<Dialog.Trigger asChild>
<button>Open Dialog</button>
</Dialog.Trigger>
<Dialog.Portal>
<Dialog.Overlay className="dialog-overlay" />
<Dialog.Content className="dialog-content">
<Dialog.Title>Welcome</Dialog.Title>
<Dialog.Description>
This is a simple dialog created with Radix UI.
</Dialog.Description>
<Dialog.Close asChild>
<button>Close</button>
</Dialog.Close>
</Dialog.Content>
</Dialog.Portal>
</Dialog.Root>
);
In this example, we’ve created a basic dialog with a trigger button, an overlay, content area, title, description, and a close button. The asChild
prop allows you to use custom elements as triggers and close buttons.
Elevating Your Dialog Game
Controlled Dialogs for Complex Scenarios
For more advanced use cases, you might want to control the dialog’s open state programmatically. Here’s how you can create a controlled dialog:
import * as Dialog from '@radix-ui/react-dialog';
import React, { useState } from 'react';
const ControlledDialog: React.FC = () => {
const [open, setOpen] = useState(false);
return (
<Dialog.Root open={open} onOpenChange={setOpen}>
<Dialog.Trigger asChild>
<button>Open Controlled Dialog</button>
</Dialog.Trigger>
<Dialog.Portal>
<Dialog.Overlay className="dialog-overlay" />
<Dialog.Content className="dialog-content">
<Dialog.Title>Controlled Dialog</Dialog.Title>
<Dialog.Description>
This dialog's state is controlled programmatically.
</Dialog.Description>
<button onClick={() => setOpen(false)}>Close</button>
</Dialog.Content>
</Dialog.Portal>
</Dialog.Root>
);
};
This controlled dialog allows you to manage its open state, which is particularly useful for scenarios involving form submissions or asynchronous operations.
Customizing Dialog Behavior
Radix Dialog provides various props to customize the behavior of your dialogs. Let’s explore some of these options:
import * as Dialog from '@radix-ui/react-dialog';
import React from 'react';
const CustomBehaviorDialog: React.FC = () => (
<Dialog.Root>
<Dialog.Trigger>Open Custom Dialog</Dialog.Trigger>
<Dialog.Portal>
<Dialog.Overlay />
<Dialog.Content
onOpenAutoFocus={(event) => event.preventDefault()}
onCloseAutoFocus={(event) => event.preventDefault()}
onEscapeKeyDown={() => console.log('Escape key pressed')}
>
<Dialog.Title>Custom Behavior Dialog</Dialog.Title>
<Dialog.Description>
This dialog has custom focus and escape key behavior.
</Dialog.Description>
<Dialog.Close>Close</Dialog.Close>
</Dialog.Content>
</Dialog.Portal>
</Dialog.Root>
);
In this example, we’ve customized the auto-focus behavior and added a custom action for the Escape key press.
Accessibility: The Radix Advantage
One of the standout features of Radix Dialog is its focus on accessibility. The library adheres to the Dialog WAI-ARIA design pattern, ensuring that your dialogs are usable by all, including those relying on assistive technologies.
Here’s a quick rundown of the keyboard interactions supported by Radix Dialog:
Space
orEnter
: Opens/closes the dialogTab
: Moves focus to the next focusable element within the dialogShift + Tab
: Moves focus to the previous focusable elementEsc
: Closes the dialog and returns focus to the trigger element
Wrapping Up
Radix Dialog offers a powerful set of tools for creating accessible, customizable, and feature-rich modal dialogs in React applications. By leveraging its primitives and customization options, you can craft dialogs that not only look great but also provide an excellent user experience for all users, regardless of their abilities or preferred input methods.
As you continue to explore Radix Dialog, remember that its flexibility allows you to create complex dialog systems tailored to your specific needs. Whether you’re building a simple confirmation prompt or a multi-step form wizard, Radix Dialog provides the foundation for creating robust and accessible modal experiences in your React applications.