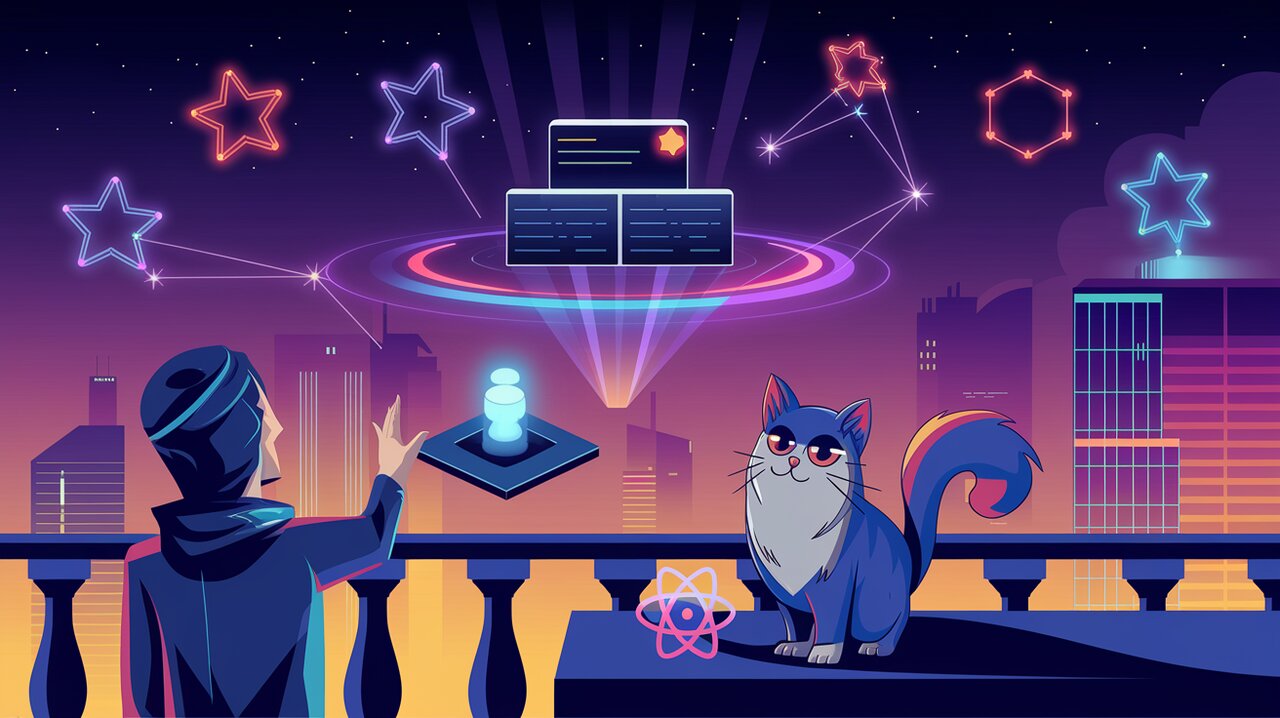
Reaching for the GraphQL Stars with React Reach
React Reach is a lightweight library that simplifies the integration of GraphQL into React applications. By providing a seamless communication layer between React components and GraphQL APIs, React Reach enables developers to efficiently fetch and manage data without the need for complex setup or additional state management libraries.
Stellar Features of React Reach
React Reach offers a constellation of features that make working with GraphQL in React a breeze:
- Effortless GraphQL Communication: React Reach provides simple functions to query and mutate data using GraphQL, eliminating the need for complex boilerplate code.
- Redux Integration: The library is designed to work alongside Redux, allowing you to cache responses in your Redux store for optimal performance.
- React Router Compatibility: When used with React Router, React Reach can dynamically request data needed for specific routes using
onEnter
andonLeave
hooks. - Lightweight and Focused: Unlike more complex solutions, React Reach does one thing and does it well, making it easy to integrate into existing projects.
Launching Your React Reach Journey
To begin your voyage with React Reach, you’ll need to install the library in your React project. Open your terminal and run:
npm install react-reach
# or
yarn add react-reach
Basic Usage: Your First GraphQL Query
Let’s explore how to make a simple GraphQL query using React Reach. Here’s an example component that fetches data about starships:
import React, { useEffect, useState } from 'react';
import { reachGraphQL } from 'react-reach';
interface Starship {
name: string;
model: string;
}
const StarshipList: React.FC = () => {
const [starships, setStarships] = useState<Starship[]>([]);
useEffect(() => {
const fetchStarships = async () => {
const result = await reachGraphQL(
'http://localhost:4000/',
`
query {
allStarships(first: 5) {
edges {
node {
name
model
}
}
}
}
`,
{}
);
setStarships(result.allStarships.edges.map((edge: any) => edge.node));
};
fetchStarships();
}, []);
return (
<div>
<h1>Starship Fleet</h1>
<ul>
{starships.map((ship, index) => (
<li key={index}>
{ship.name} - {ship.model}
</li>
))}
</ul>
</div>
);
};
export default StarshipList;
In this example, we use the reachGraphQL
function to fetch data from our GraphQL server. The function takes three parameters: the server URL, the GraphQL query, and any variables (in this case, an empty object).
Advanced Maneuvers: Caching with Redux
React Reach can work in tandem with Redux to cache API responses. Here’s how you can use the reachWithDispatch
function to automatically cache data in your Redux store:
import { reachWithDispatch } from 'react-reach';
import { useDispatch } from 'react-redux';
const UserProfile: React.FC<{ userId: string }> = ({ userId }) => {
const dispatch = useDispatch();
useEffect(() => {
const fetchUserProfile = async () => {
await reachWithDispatch(
'http://localhost:4000/',
`
query($id: ID!) {
user(id: $id) {
name
email
}
}
`,
{ id: userId },
(data) => ({ type: 'SET_USER_PROFILE', payload: data.user })
);
};
fetchUserProfile();
}, [userId, dispatch]);
// Render user profile...
};
In this advanced example, reachWithDispatch
not only fetches the data but also dispatches an action to update the Redux store with the fetched user profile.
Navigating CORS Space
When working with React Reach, you might encounter CORS (Cross-Origin Resource Sharing) issues. To avoid these, ensure your server is configured to handle CORS requests. Here’s an example of how to set up CORS with Express:
import express from 'express';
import cors from 'cors';
const app = express();
app.use(cors({
origin: 'http://localhost:3000', // Your React app's URL
methods: ['GET', 'POST'],
allowedHeaders: ['Content-Type', 'Authorization']
}));
// Your GraphQL endpoint setup...
Conclusion: Charting Your Course with React Reach
React Reach offers a streamlined approach to integrating GraphQL into your React applications. Its simplicity and focus on doing one thing well make it an excellent choice for developers looking to add GraphQL capabilities without the overhead of more complex libraries.
As you continue your journey with React Reach, you’ll discover how it can simplify your data fetching logic and improve the overall structure of your React applications. Whether you’re building a small project or a large-scale application, React Reach provides the tools you need to efficiently communicate with your GraphQL APIs.
For more insights on working with GraphQL in React, you might want to explore our articles on Adrenaline Rush: React Data Fetching and Mastering React Modals with React Modal. These resources can complement your React Reach knowledge and help you build more robust and efficient React applications.