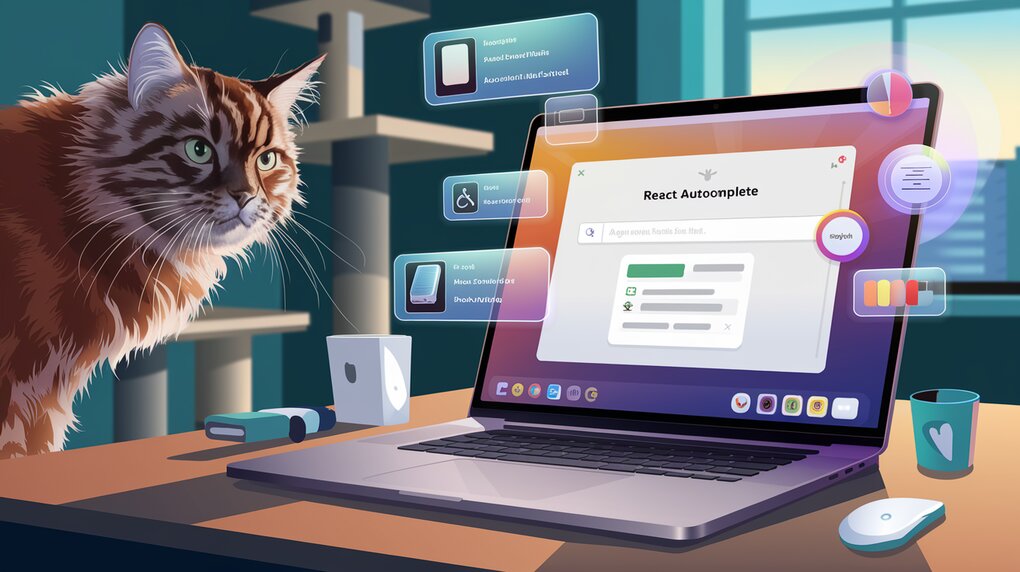
Supercharge Your Forms with React Autosuggest: The Smart Way to Enhance User Input
React Autosuggest is a powerful library that transforms ordinary input fields into intelligent, user-friendly interfaces. By providing customizable suggestion capabilities, it enhances the user experience and streamlines data entry in your React applications. Let’s dive into the world of React Autosuggest and discover how it can supercharge your forms.
Features
React Autosuggest comes packed with an impressive array of features that make it stand out:
- WAI-ARIA compliance, ensuring accessibility for all users
- Mobile-friendly design for seamless use across devices
- Flexibility to integrate with Flux and Redux applications
- Customizable suggestion rendering
- Support for plain lists or multiple sections of suggestions
- Asynchronous suggestion retrieval
- Option to highlight the first suggestion
- Compatibility with various styling solutions (CSS Modules, Radium, Aphrodite, JSS)
- Controlled rendering of suggestions based on custom logic
- Ability to always render suggestions (great for mobile and modals)
- Extensible input props for maximum customization
Installation
Getting started with React Autosuggest is straightforward. You can install it using npm or yarn:
npm install react-autosuggest --save
or
yarn add react-autosuggest
For those who prefer using a CDN, you can include the UMD build in your HTML:
<script src="https://unpkg.com/react-autosuggest/dist/standalone/autosuggest.js"></script>
Basic Usage
Let’s walk through a simple example to demonstrate the basic usage of React Autosuggest:
import React, { useState } from 'react';
import Autosuggest from 'react-autosuggest';
// Sample data
const languages = [
{ name: 'C', year: 1972 },
{ name: 'Elm', year: 2012 },
{ name: 'JavaScript', year: 1995 },
];
// Suggestion generation function
const getSuggestions = (value: string) => {
const inputValue = value.trim().toLowerCase();
const inputLength = inputValue.length;
return inputLength === 0
? []
: languages.filter(
(lang) => lang.name.toLowerCase().slice(0, inputLength) === inputValue
);
};
// Function to get the value for a suggestion
const getSuggestionValue = (suggestion: { name: string }) => suggestion.name;
// Render function for suggestions
const renderSuggestion = (suggestion: { name: string; year: number }) => (
<div>
{suggestion.name} ({suggestion.year})
</div>
);
const AutosuggestExample: React.FC = () => {
const [value, setValue] = useState('');
const [suggestions, setSuggestions] = useState<any[]>([]);
const onChange = (event: any, { newValue }: { newValue: string }) => {
setValue(newValue);
};
const onSuggestionsFetchRequested = ({ value }: { value: string }) => {
setSuggestions(getSuggestions(value));
};
const onSuggestionsClearRequested = () => {
setSuggestions([]);
};
const inputProps = {
placeholder: 'Type a programming language',
value,
onChange,
};
return (
<Autosuggest
suggestions={suggestions}
onSuggestionsFetchRequested={onSuggestionsFetchRequested}
onSuggestionsClearRequested={onSuggestionsClearRequested}
getSuggestionValue={getSuggestionValue}
renderSuggestion={renderSuggestion}
inputProps={inputProps}
/>
);
};
export default AutosuggestExample;
This example sets up a basic autosuggest component for programming languages. Let’s break down the key elements:
- We define a list of languages and functions to generate and render suggestions.
- The component uses React hooks to manage state for the input value and suggestions.
- The
Autosuggest
component is configured with necessary props, including callbacks for fetching and clearing suggestions.
Advanced Usage
Custom Styling
React Autosuggest doesn’t come with predefined styles, giving you full control over the appearance. You can use various styling solutions, including CSS Modules:
import styles from './theme.css';
// ...
<Autosuggest
// ... other props
theme={{
container: styles.container,
input: styles.input,
suggestionsContainer: styles.suggestionsContainer,
suggestionsList: styles.suggestionsList,
suggestion: styles.suggestion,
suggestionHighlighted: styles.suggestionHighlighted,
}}
/>
Multi-Section Rendering
For more complex scenarios, you might want to group suggestions into sections:
const multiSectionSuggestions = [
{
title: 'A',
languages: [
{ name: 'ActionScript', year: 1995 },
{ name: 'AppleScript', year: 1993 },
],
},
{
title: 'C',
languages: [
{ name: 'C', year: 1972 },
{ name: 'C#', year: 2000 },
],
},
];
const renderSectionTitle = (section: { title: string }) => (
<strong>{section.title}</strong>
);
const getSectionSuggestions = (section: { languages: any[] }) => section.languages;
// ...
<Autosuggest
multiSection={true}
suggestions={multiSectionSuggestions}
renderSectionTitle={renderSectionTitle}
getSectionSuggestions={getSectionSuggestions}
// ... other props
/>
Asynchronous Suggestions
For scenarios where suggestions need to be fetched from an API, you can implement asynchronous loading:
const fetchSuggestions = async (value: string) => {
const response = await fetch(`https://api.example.com/suggestions?q=${value}`);
return response.json();
};
const onSuggestionsFetchRequested = async ({ value }: { value: string }) => {
const suggestions = await fetchSuggestions(value);
setSuggestions(suggestions);
};
// ...
<Autosuggest
// ... other props
onSuggestionsFetchRequested={onSuggestionsFetchRequested}
/>
Conclusion
React Autosuggest is a versatile and powerful library that can significantly enhance the user experience of your forms and input fields. Its flexibility allows for seamless integration into various React applications, from simple autocomplete inputs to complex, multi-section suggestion interfaces.
By leveraging React Autosuggest, you can create more intuitive, efficient, and accessible user interfaces. Whether you’re building a search feature, a form with predictive input, or any interface that benefits from intelligent suggestions, React Autosuggest provides the tools you need to create a polished and user-friendly experience.
Remember to explore the extensive documentation and examples provided by the React Autosuggest team to unlock its full potential in your projects. Happy coding!