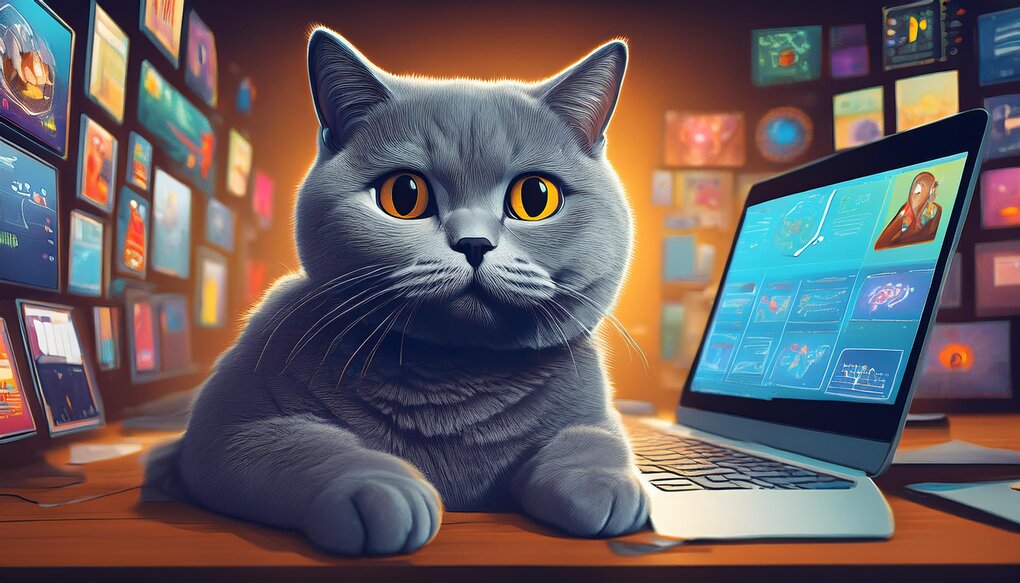
Introducing the React Avatar Editor Library
The React Avatar Editor library is a versatile tool designed to help you create a user-friendly avatar editor for your React applications. This library allows you to resize, crop, and rotate your uploaded images, making it perfect for profile picture and avatar needs. In this article, we will explore the basics and advanced usage of this library, simplifying the original documentation to make it easier to understand and faster to grasp for beginners.
Installation
To get started with the React Avatar Editor library, you can use your favorite package manager to add it to your project:
yarn add react-avatar-editor
npm install --save react-avatar-editor
pnpm add react-avatar-editor
Basic Usage
Here is a simple example of how to use the React Avatar Editor library:
import React from 'react';
import AvatarEditor from 'react-avatar-editor';
const MyEditor = () => {
return (
<AvatarEditor
image="http://example.com/initialimage.jpg"
width={250}
height={250}
border={50}
color={[255, 255, 255, 0.6]} // RGBA
scale={1.2}
rotate={0}
/>
);
};
export default MyEditor;
Advanced Usage
For more advanced scenarios, you can use the library to add drag and drop support and access the resulting image:
import React, { useRef } from 'react';
import AvatarEditor from 'react-avatar-editor';
import Dropzone from 'react-dropzone';
const MyEditor = () => {
const editor = useRef(null);
return (
<div>
<Dropzone
onDrop={(dropped) => {
if (editor.current) {
const canvas = editor.current.getImage();
const canvasScaled = editor.current.getImageScaledToCanvas();
}
}}
noClick
noKeyboard
style={{ width: '250px', height: '250px' }}
>
{({ getRootProps, getInputProps }) => (
<div {...getRootProps()}>
<AvatarEditor
ref={editor}
image="http://example.com/initialimage.jpg"
width={250}
height={250}
border={50}
scale={1.2}
/>
<input {...getInputProps()} />
</div>
)}
</Dropzone>
</div>
);
};
export default MyEditor;
Conclusion
The React Avatar Editor library is a powerful tool for creating versatile and user-friendly avatar editors in your React applications. With its ability to resize, crop, and rotate images, it is perfect for profile picture and avatar needs. By following the basic and advanced usage examples provided in this article, you can easily integrate this library into your projects and enhance your user experience.
For more information, you can visit the official documentation and contribute to the project if you wish to further enhance its capabilities.