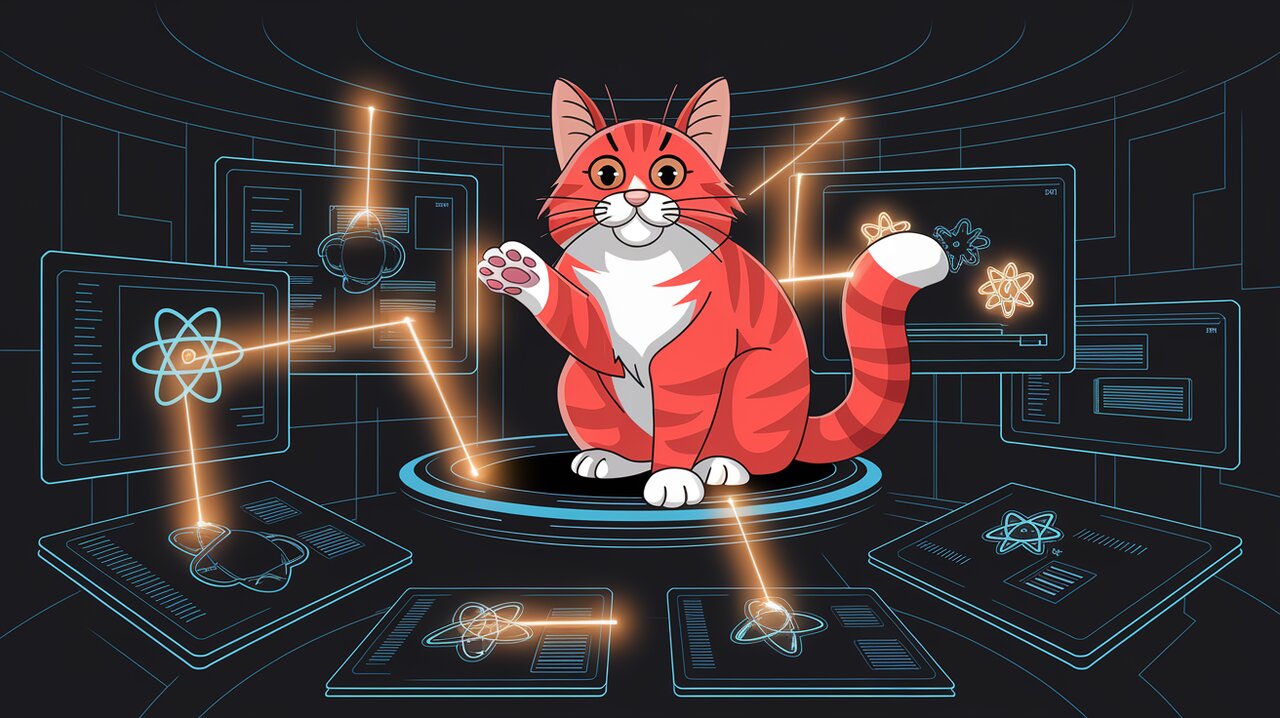
React-Call: Simplifying Component Communication in React
Introduction
React applications often require complex communication between components, especially when dealing with asynchronous operations or managing global state. While solutions like Redux or Context API exist, they can sometimes feel overly complex for simpler use cases. This is where react-call comes in, offering a more intuitive and straightforward approach to component communication.
Features
React-call provides several key features that make it stand out:
- Simplified API Calls: Easily make asynchronous calls from any component without prop drilling.
- Type-Safe Communication: Leverages TypeScript for type-safe component interactions.
- Flexible Usage: Can be used with both class and functional components.
- Minimal Boilerplate: Reduces the amount of code needed for component communication.
Installation
To get started with react-call, you can install it using npm or yarn:
npm install react-call
or
yarn add react-call
Basic Usage
Creating a Callable Component
Let’s start by creating a simple confirmation dialog using react-call:
import { callable } from 'react-call';
interface ConfirmProps {
message: string;
}
const Confirm = callable<ConfirmProps, boolean>(({ message, resolve }) => {
return (
<div>
<p>{message}</p>
<button onClick={() => resolve(true)}>Yes</button>
<button onClick={() => resolve(false)}>No</button>
</div>
);
});
export default Confirm;
In this example, we’ve created a Confirm
component that takes a message
prop and resolves with a boolean value indicating the user’s choice.
Using the Callable Component
Now, let’s see how we can use this Confirm
component in another part of our application:
import React from 'react';
import Confirm from './Confirm';
const DeleteItem: React.FC = () => {
const handleDelete = async () => {
const confirmed = await Confirm.call({ message: 'Are you sure you want to delete this item?' });
if (confirmed) {
// Perform delete operation
console.log('Item deleted');
}
};
return <button onClick={handleDelete}>Delete Item</button>;
};
export default DeleteItem;
Here, we use the Confirm.call()
method to show the confirmation dialog. The call()
method returns a promise that resolves with the user’s choice, allowing us to handle the result in an async/await fashion.
Advanced Usage
Using with Class Components
React-call is not limited to functional components. Here’s how you can use it with class components:
import React from 'react';
import { withCall, CallProp } from 'react-call';
import Confirm from './Confirm';
interface Props extends CallProp {}
class DeleteButton extends React.Component<Props> {
handleDelete = async () => {
const { call } = this.props;
const confirmed = await call(Confirm, { message: 'Are you sure?' });
if (confirmed) {
console.log('Item deleted');
}
};
render() {
return <button onClick={this.handleDelete}>Delete</button>;
}
}
export default withCall(DeleteButton);
The withCall
higher-order component provides the call
prop, which can be used to invoke callable components.
Creating Complex Dialogs
React-call shines when creating more complex interactions. Let’s create a dialog for editing user information:
import { callable } from 'react-call';
interface UserInfo {
name: string;
email: string;
}
interface EditUserProps {
initialData: UserInfo;
}
const EditUser = callable<EditUserProps, UserInfo | null>(({ initialData, resolve }) => {
const [userData, setUserData] = React.useState(initialData);
const handleSubmit = (e: React.FormEvent) => {
e.preventDefault();
resolve(userData);
};
const handleCancel = () => {
resolve(null);
};
return (
<form onSubmit={handleSubmit}>
<input
value={userData.name}
onChange={(e) => setUserData({ ...userData, name: e.target.value })}
/>
<input
value={userData.email}
onChange={(e) => setUserData({ ...userData, email: e.target.value })}
/>
<button type="submit">Save</button>
<button type="button" onClick={handleCancel}>Cancel</button>
</form>
);
});
export default EditUser;
Using this component is just as straightforward:
const handleEditUser = async () => {
const updatedUser = await EditUser.call({ initialData: currentUser });
if (updatedUser) {
// Update user information
console.log('User updated:', updatedUser);
}
};
Conclusion
React-call offers a fresh perspective on component communication in React applications. By providing a simple and intuitive API, it allows developers to create interactive and responsive user interfaces without the complexity of traditional state management solutions. Whether you’re building a small project or a large-scale application, react-call can significantly simplify your component interactions, leading to cleaner and more maintainable code.
As you explore react-call further, you’ll discover its flexibility in handling various scenarios, from simple confirmations to complex data entry forms. Its seamless integration with both functional and class components makes it a versatile tool in any React developer’s toolkit. Give react-call a try in your next project and experience the simplicity of component communication it brings to your React applications.