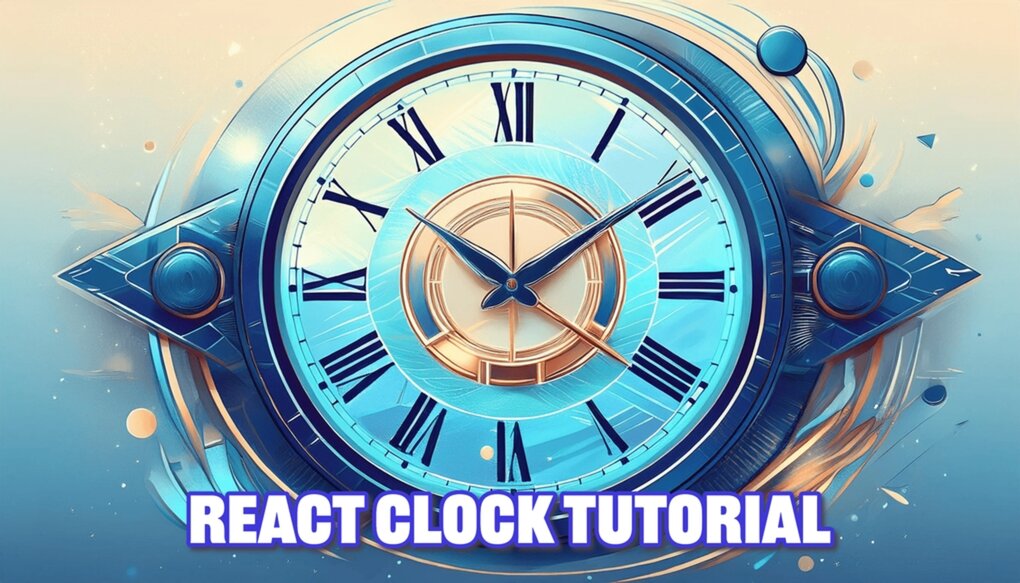
React-Clock: Adding Customizable Analog Clocks to Your React Apps
Hey there, React developers! Are you looking for an easy way to add a sleek analog clock to your React application? Look no further than react-clock
! This nifty little library allows you to create beautiful, customizable analog clocks with just a few lines of code. Let’s dive in and see how it works!
What is react-clock?
react-clock
is a React component that renders an analog clock. It’s perfect for adding a visual time display to your web applications, dashboards, or anywhere else you need to show the current time in a stylish way.
Getting Started
First things first, let’s install the library. Open your terminal and run:
npm install react-clock
or if you’re using Yarn:
yarn add react-clock
Now, let’s create a basic clock component. Here’s a simple example:
import React, { useState, useEffect } from 'react';
import Clock from 'react-clock';
import 'react-clock/dist/Clock.css';
function MyClock() {
const [value, setValue] = useState(new Date());
useEffect(() => {
const interval = setInterval(() => setValue(new Date()), 1000);
return () => clearInterval(interval);
}, []);
return (
<div>
<h2>Current Time:</h2>
<Clock value={value} />
</div>
);
}
export default MyClock;
In this example, we’re importing the Clock
component and its default styles. We use the useState
and useEffect
hooks to update the clock every second. The value
prop of the Clock
component is set to the current date and time.
Customizing Your Clock
One of the great things about react-clock
is how customizable it is. Let’s look at some ways to make your clock unique:
Changing the Size
You can easily adjust the size of your clock using the size
prop:
<Clock value={value} size={200} />
This will create a clock that’s 200 pixels wide.
Customizing Hands
You can modify the length and width of the clock hands:
<Clock
value={value}
hourHandLength={60}
hourHandWidth={4}
minuteHandLength={80}
minuteHandWidth={3}
secondHandLength={90}
secondHandWidth={2}
/>
Showing or Hiding Elements
You can choose which elements of the clock to display:
<Clock
value={value}
renderNumbers={true}
renderSecondHand={false}
/>
This will show numbers on the clock face and hide the second hand.
Advanced Usage
Custom Hour Formatting
You can provide a custom function to format the hour marks:
const formatHour = (locale?: string, hour?: number) => {
const index = [12, 3, 6, 9].indexOf(hour ?? 0);
return ['XII', 'III', 'VI', 'IX'][index] || '';
};
<Clock value={value} formatHour={formatHour} />
This will display Roman numerals at 12, 3, 6, and 9 o’clock positions.
Millisecond Precision
For applications that require extremely precise time display, you can enable millisecond precision:
<Clock value={value} useMillisecondPrecision={true} />
Localization
You can set the locale for the clock, which can be useful for server-side rendering or when you want to display time in a specific format:
<Clock value={value} locale="fr-FR" />
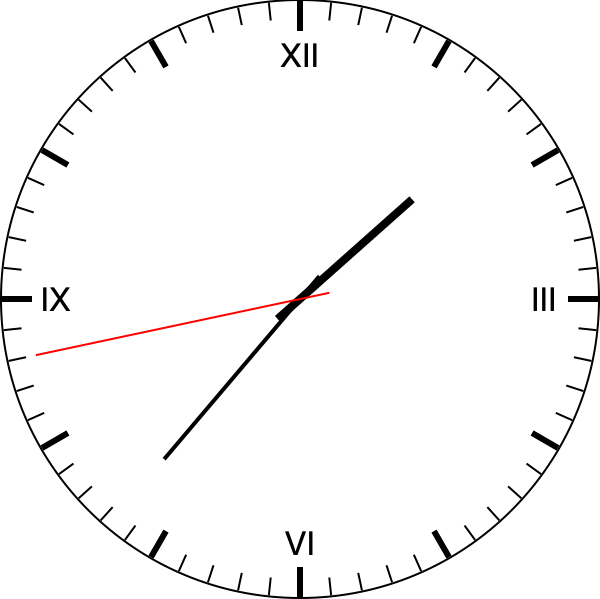
Wrapping Up
The react-clock
library offers a simple yet powerful way to add analog clocks to your React applications. With its extensive customization options, you can create clocks that perfectly match your app’s design.
Remember to check out the official documentation for a full list of props and more advanced usage examples. Happy coding, and may your React apps always show the right time! 😊
Would you like me to explain or break down any part of this article further?